mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
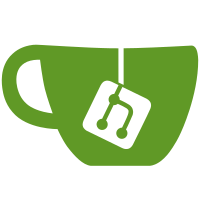
dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64 refactor: test: use _ variable for unused loop counters (Sebastian Falbesoner)
Pull request description:
This tiny PR substitutes Python loops in the form of `for x in range(N): ...` by `for _ in range(N): ...` where applicable. The idea is indicating to the reader that a block (or statement, in list comprehensions) is just repeated N times, and that the loop counter is not used in the body, hence using the throwaway variable. This is already done quite often in the current tests (see e.g. `$ git grep "for _ in range("`). Another alternative would be using `itertools.repeat` (according to Python core developer Raymond Hettinger it's [even faster](https://twitter.com/raymondh/status/1144527183341375488)), but that doesn't seem to be widespread in use and I'm not sure about a readability increase.
The only drawback I see is that whenever one wants to debug loop iterations, one would need to introduce a loop variable again. Reviewing this is basically a no-brainer, since tests would fail immediately if a a substitution has taken place on a loop where the variable is used.
Instances to replace were found by `$ git grep "for.*in range("` and manually checked.
ACKs for top commit:
darosior:
ACK dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64
instagibbs:
manual inspection ACK dac7a111bd
practicalswift:
ACK dac7a111bdd3b0233d94cf68dae7a8bfc6ac9c64 -- the updated code is easier to reason about since the throwaway nature of a variable is expressed explicitly (using the Pythonic `_` idiom) instead of implicitly. Explicit is better than implicit was we all know by now :)
Tree-SHA512: 5f43ded9ce14e5e00b3876ec445b90acda1842f813149ae7bafa93f3ac3d510bb778e2c701187fd2c73585e6b87797bb2d2987139bd1a9ba7d58775a59392406
75 lines
2.9 KiB
Python
Executable File
75 lines
2.9 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2018 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import (
|
|
assert_equal,
|
|
assert_raises_rpc_error,
|
|
)
|
|
|
|
|
|
class CreateTxWalletTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.num_nodes = 1
|
|
|
|
def skip_test_if_missing_module(self):
|
|
self.skip_if_no_wallet()
|
|
|
|
def run_test(self):
|
|
self.test_anti_fee_sniping()
|
|
self.test_tx_size_too_large()
|
|
|
|
def test_anti_fee_sniping(self):
|
|
self.log.info('Check that we have some (old) blocks and that anti-fee-sniping is disabled')
|
|
self.bump_mocktime(8 * 60 * 60 + 1)
|
|
assert_equal(self.nodes[0].getblockchaininfo()['blocks'], 200)
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[0].getnewaddress(), 1)
|
|
tx = self.nodes[0].decoderawtransaction(self.nodes[0].gettransaction(txid)['hex'])
|
|
assert_equal(tx['locktime'], 0)
|
|
|
|
self.log.info('Check that anti-fee-sniping is enabled when we mine a recent block')
|
|
self.nodes[0].generate(1)
|
|
txid = self.nodes[0].sendtoaddress(self.nodes[0].getnewaddress(), 1)
|
|
tx = self.nodes[0].decoderawtransaction(self.nodes[0].gettransaction(txid)['hex'])
|
|
assert 0 < tx['locktime'] <= 201
|
|
|
|
def test_tx_size_too_large(self):
|
|
# More than 10kB of outputs, so that we hit -maxtxfee with a high feerate
|
|
outputs = {self.nodes[0].getnewaddress(): 0.000025 for _ in range(400)}
|
|
raw_tx = self.nodes[0].createrawtransaction(inputs=[], outputs=outputs)
|
|
|
|
for fee_setting in ['-minrelaytxfee=0.01', '-mintxfee=0.01', '-paytxfee=0.01']:
|
|
self.log.info('Check maxtxfee in combination with {}'.format(fee_setting))
|
|
self.restart_node(0, extra_args=[fee_setting])
|
|
assert_raises_rpc_error(
|
|
-6,
|
|
"Fee exceeds maximum configured by -maxtxfee",
|
|
lambda: self.nodes[0].sendmany(dummy="", amounts=outputs),
|
|
)
|
|
assert_raises_rpc_error(
|
|
-4,
|
|
"Fee exceeds maximum configured by -maxtxfee",
|
|
lambda: self.nodes[0].fundrawtransaction(hexstring=raw_tx),
|
|
)
|
|
|
|
self.log.info('Check maxtxfee in combination with settxfee')
|
|
self.restart_node(0)
|
|
self.nodes[0].settxfee(0.01)
|
|
assert_raises_rpc_error(
|
|
-6,
|
|
"Fee exceeds maximum configured by -maxtxfee",
|
|
lambda: self.nodes[0].sendmany(dummy="", amounts=outputs),
|
|
)
|
|
assert_raises_rpc_error(
|
|
-4,
|
|
"Fee exceeds maximum configured by -maxtxfee",
|
|
lambda: self.nodes[0].fundrawtransaction(hexstring=raw_tx),
|
|
)
|
|
self.nodes[0].settxfee(0)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
CreateTxWalletTest().main()
|