mirror of
https://github.com/dashpay/dash.git
synced 2024-12-27 21:12:48 +01:00
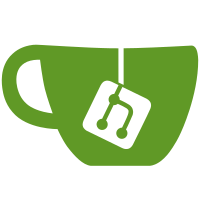
54326a6
Increase maximum orphan size to 100,000 bytes. (Gregory Maxwell)8c99d1b
Treat orphans as implicit inv for parents, discard when parents rejected. (Gregory Maxwell)11cc143
Adds an expiration time for orphan tx. (Gregory Maxwell)db0ffe8
This eliminates the primary leak that causes the orphan map to always grow to its maximum size. (Gregory Maxwell)1b0bcc5
Track orphan by prev COutPoint rather than prev hash (Pieter Wuille)
65 lines
2.5 KiB
C++
65 lines
2.5 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_NET_PROCESSING_H
|
|
#define BITCOIN_NET_PROCESSING_H
|
|
|
|
#include "net.h"
|
|
#include "validationinterface.h"
|
|
|
|
/** Default for -maxorphantx, maximum number of orphan transactions kept in memory */
|
|
static const unsigned int DEFAULT_MAX_ORPHAN_TRANSACTIONS = 100;
|
|
/** Expiration time for orphan transactions in seconds */
|
|
static const int64_t ORPHAN_TX_EXPIRE_TIME = 20 * 60;
|
|
/** Minimum time between orphan transactions expire time checks in seconds */
|
|
static const int64_t ORPHAN_TX_EXPIRE_INTERVAL = 5 * 60;
|
|
|
|
/** Headers download timeout expressed in microseconds
|
|
* Timeout = base + per_header * (expected number of headers) */
|
|
static constexpr int64_t HEADERS_DOWNLOAD_TIMEOUT_BASE = 15 * 60 * 1000000; // 15 minutes
|
|
static constexpr int64_t HEADERS_DOWNLOAD_TIMEOUT_PER_HEADER = 1000; // 1ms/header
|
|
|
|
/** Register with a network node to receive its signals */
|
|
void RegisterNodeSignals(CNodeSignals& nodeSignals);
|
|
/** Unregister a network node */
|
|
void UnregisterNodeSignals(CNodeSignals& nodeSignals);
|
|
|
|
class PeerLogicValidation : public CValidationInterface {
|
|
private:
|
|
CConnman* connman;
|
|
|
|
public:
|
|
PeerLogicValidation(CConnman* connmanIn);
|
|
|
|
virtual void UpdatedBlockTip(const CBlockIndex *pindexNew, const CBlockIndex *pindexFork, bool fInitialDownload);
|
|
virtual void BlockChecked(const CBlock& block, const CValidationState& state);
|
|
};
|
|
|
|
struct CNodeStateStats {
|
|
int nMisbehavior;
|
|
int nSyncHeight;
|
|
int nCommonHeight;
|
|
std::vector<int> vHeightInFlight;
|
|
};
|
|
|
|
/** Get statistics from node state */
|
|
bool GetNodeStateStats(NodeId nodeid, CNodeStateStats &stats);
|
|
/** Increase a node's misbehavior score. */
|
|
void Misbehaving(NodeId nodeid, int howmuch);
|
|
|
|
/** Process protocol messages received from a given node */
|
|
bool ProcessMessages(CNode* pfrom, CConnman& connman, std::atomic<bool>& interrupt);
|
|
/**
|
|
* Send queued protocol messages to be sent to a give node.
|
|
*
|
|
* @param[in] pto The node which we are sending messages to.
|
|
* @param[in] connman The connection manager for that node.
|
|
* @param[in] interrupt Interrupt condition for processing threads
|
|
* @return True if there is more work to be done
|
|
*/
|
|
bool SendMessages(CNode* pto, CConnman& connman, std::atomic<bool>& interrupt);
|
|
|
|
#endif // BITCOIN_NET_PROCESSING_H
|