mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
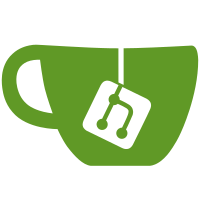
78e407ad0c26190a22de1bc8ed900164a44a36c3 GetKeyBirthTimes should return key ids, not destinations (Gregory Sanders)
70946e7fee54323ce6a5ea8aeb377e2c7c790bc6 Replace CScriptID and CKeyID in CTxDestination with dedicated types (Gregory Sanders)
Pull request description:
The current usage seems to be an overloading of meanings. `CScriptID` is used in the wallet as a lookup key, as well as a destination, and `CKeyID` likewise. Instead, have all destinations be dedicated types.
New types:
`CScriptID`->`ScriptHash`
`CKeyID`->`PKHash`
ACKs for commit 78e407:
ryanofsky:
utACK 78e407ad0c26190a22de1bc8ed900164a44a36c3. Only changes are removing extra CScriptID()s and fixing the test case.
Sjors:
utACK 78e407a
meshcollider:
utACK 78e407ad0c
Tree-SHA512: 437f59fc3afb83a40540da3351507aef5aed44e3a7f15b01ddad6226854edeee762ff0b0ef336fe3654c4cd99a205cef175211de8b639abe1130c8a6313337b9
165 lines
5.6 KiB
C++
165 lines
5.6 KiB
C++
// Copyright (c) 2014-2016 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <key_io.h>
|
|
|
|
#include <base58.h>
|
|
#include <bech32.h>
|
|
#include <chainparams.h>
|
|
|
|
#include <algorithm>
|
|
#include <assert.h>
|
|
#include <string.h>
|
|
|
|
namespace {
|
|
class DestinationEncoder
|
|
{
|
|
private:
|
|
const CChainParams& m_params;
|
|
|
|
public:
|
|
explicit DestinationEncoder(const CChainParams& params) : m_params(params) {}
|
|
|
|
std::string operator()(const PKHash& id) const
|
|
{
|
|
std::vector<unsigned char> data = m_params.Base58Prefix(CChainParams::PUBKEY_ADDRESS);
|
|
data.insert(data.end(), id.begin(), id.end());
|
|
return EncodeBase58Check(data);
|
|
}
|
|
|
|
std::string operator()(const ScriptHash& id) const
|
|
{
|
|
std::vector<unsigned char> data = m_params.Base58Prefix(CChainParams::SCRIPT_ADDRESS);
|
|
data.insert(data.end(), id.begin(), id.end());
|
|
return EncodeBase58Check(data);
|
|
}
|
|
|
|
std::string operator()(const CNoDestination& no) const { return {}; }
|
|
};
|
|
|
|
CTxDestination DecodeDestination(const std::string& str, const CChainParams& params)
|
|
{
|
|
std::vector<unsigned char> data;
|
|
uint160 hash;
|
|
if (DecodeBase58Check(str, data, 21)) {
|
|
// base58-encoded Dash addresses.
|
|
// Public-key-hash-addresses have version 76 (or 140 testnet).
|
|
// The data vector contains RIPEMD160(SHA256(pubkey)), where pubkey is the serialized public key.
|
|
const std::vector<unsigned char>& pubkey_prefix = params.Base58Prefix(CChainParams::PUBKEY_ADDRESS);
|
|
if (data.size() == hash.size() + pubkey_prefix.size() && std::equal(pubkey_prefix.begin(), pubkey_prefix.end(), data.begin())) {
|
|
std::copy(data.begin() + pubkey_prefix.size(), data.end(), hash.begin());
|
|
return PKHash(hash);
|
|
}
|
|
// Script-hash-addresses have version 16 (or 19 testnet).
|
|
// The data vector contains RIPEMD160(SHA256(cscript)), where cscript is the serialized redemption script.
|
|
const std::vector<unsigned char>& script_prefix = params.Base58Prefix(CChainParams::SCRIPT_ADDRESS);
|
|
if (data.size() == hash.size() + script_prefix.size() && std::equal(script_prefix.begin(), script_prefix.end(), data.begin())) {
|
|
std::copy(data.begin() + script_prefix.size(), data.end(), hash.begin());
|
|
return ScriptHash(hash);
|
|
}
|
|
}
|
|
return CNoDestination();
|
|
}
|
|
} // namespace
|
|
|
|
CKey DecodeSecret(const std::string& str)
|
|
{
|
|
CKey key;
|
|
std::vector<unsigned char> data;
|
|
if (DecodeBase58Check(str, data, 34)) {
|
|
const std::vector<unsigned char>& privkey_prefix = Params().Base58Prefix(CChainParams::SECRET_KEY);
|
|
if ((data.size() == 32 + privkey_prefix.size() || (data.size() == 33 + privkey_prefix.size() && data.back() == 1)) &&
|
|
std::equal(privkey_prefix.begin(), privkey_prefix.end(), data.begin())) {
|
|
bool compressed = data.size() == 33 + privkey_prefix.size();
|
|
key.Set(data.begin() + privkey_prefix.size(), data.begin() + privkey_prefix.size() + 32, compressed);
|
|
}
|
|
}
|
|
if (!data.empty()) {
|
|
memory_cleanse(data.data(), data.size());
|
|
}
|
|
return key;
|
|
}
|
|
|
|
std::string EncodeSecret(const CKey& key)
|
|
{
|
|
assert(key.IsValid());
|
|
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::SECRET_KEY);
|
|
data.insert(data.end(), key.begin(), key.end());
|
|
if (key.IsCompressed()) {
|
|
data.push_back(1);
|
|
}
|
|
std::string ret = EncodeBase58Check(data);
|
|
memory_cleanse(data.data(), data.size());
|
|
return ret;
|
|
}
|
|
|
|
CExtPubKey DecodeExtPubKey(const std::string& str)
|
|
{
|
|
CExtPubKey key;
|
|
std::vector<unsigned char> data;
|
|
if (DecodeBase58Check(str, data, 78)) {
|
|
const std::vector<unsigned char>& prefix = Params().Base58Prefix(CChainParams::EXT_PUBLIC_KEY);
|
|
if (data.size() == BIP32_EXTKEY_SIZE + prefix.size() && std::equal(prefix.begin(), prefix.end(), data.begin())) {
|
|
key.Decode(data.data() + prefix.size());
|
|
}
|
|
}
|
|
return key;
|
|
}
|
|
|
|
std::string EncodeExtPubKey(const CExtPubKey& key)
|
|
{
|
|
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::EXT_PUBLIC_KEY);
|
|
size_t size = data.size();
|
|
data.resize(size + BIP32_EXTKEY_SIZE);
|
|
key.Encode(data.data() + size);
|
|
std::string ret = EncodeBase58Check(data);
|
|
return ret;
|
|
}
|
|
|
|
CExtKey DecodeExtKey(const std::string& str)
|
|
{
|
|
CExtKey key;
|
|
std::vector<unsigned char> data;
|
|
if (DecodeBase58Check(str, data, 78)) {
|
|
const std::vector<unsigned char>& prefix = Params().Base58Prefix(CChainParams::EXT_SECRET_KEY);
|
|
if (data.size() == BIP32_EXTKEY_SIZE + prefix.size() && std::equal(prefix.begin(), prefix.end(), data.begin())) {
|
|
key.Decode(data.data() + prefix.size());
|
|
}
|
|
}
|
|
return key;
|
|
}
|
|
|
|
std::string EncodeExtKey(const CExtKey& key)
|
|
{
|
|
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::EXT_SECRET_KEY);
|
|
size_t size = data.size();
|
|
data.resize(size + BIP32_EXTKEY_SIZE);
|
|
key.Encode(data.data() + size);
|
|
std::string ret = EncodeBase58Check(data);
|
|
if (!data.empty()) {
|
|
memory_cleanse(data.data(), data.size());
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
std::string EncodeDestination(const CTxDestination& dest)
|
|
{
|
|
return std::visit(DestinationEncoder(Params()), dest);
|
|
}
|
|
|
|
CTxDestination DecodeDestination(const std::string& str)
|
|
{
|
|
return DecodeDestination(str, Params());
|
|
}
|
|
|
|
bool IsValidDestinationString(const std::string& str, const CChainParams& params)
|
|
{
|
|
return IsValidDestination(DecodeDestination(str, params));
|
|
}
|
|
|
|
bool IsValidDestinationString(const std::string& str)
|
|
{
|
|
return IsValidDestinationString(str, Params());
|
|
}
|