mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
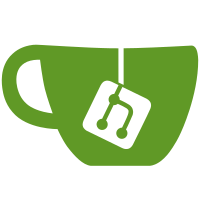
* tests: extend "age" period in `feature_llmq_connections.py` see `NOTE` * tests: sleep more in `wait_until` by default Avoid overloading rpc with 20+ requests per second, 2 should be enough. * tests: various fixes in `activate_dip0024` - lower batch size - no fast mode - disable spork17 while mining - bump mocktime on every generate call * tests: bump mocktime on generate in `activate_dip8` * tests: fix `reindex` option in `restart_mn` Make sure nodes actually finished reindexing before moving any further. * tests: trigger recovery threads and wait on mn restarts * tests: sync blocks in `wait_for_quorum_data` * tests: bump disconnect timeouts in `p2p_invalid_messages.py` 1 is too low for busy nodes * tests: Wait for addrv2 processing before bumping mocktime in p2p_addrv2_relay.py * tests: use `timeout_scale` option in `get_recovered_sig` and `isolate_node` * tests: fix `wait_for...`s * tests: fix `close_mn_port` banning test * Bump MASTERNODE_SYNC_RESET_SECONDS to 900 This helps to avoid issues with 10m+ bump_mocktime on isolated nodes in feature_llmq_is_retroactive.py and feature_llmq_simplepose.py. * style: fix extra whitespace Co-authored-by: PastaPastaPasta <6443210+PastaPastaPasta@users.noreply.github.com>
88 lines
2.7 KiB
Python
Executable File
88 lines
2.7 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2020 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""
|
|
Test addrv2 relay
|
|
"""
|
|
|
|
from test_framework.messages import (
|
|
CAddress,
|
|
msg_addrv2,
|
|
NODE_NETWORK,
|
|
)
|
|
from test_framework.mininode import P2PInterface
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal, wait_until
|
|
|
|
|
|
class AddrReceiver(P2PInterface):
|
|
addrv2_received_and_checked = False
|
|
|
|
def __init__(self):
|
|
super().__init__(support_addrv2 = True)
|
|
|
|
def on_addrv2(self, message):
|
|
for addr in message.addrs:
|
|
assert_equal(addr.nServices, 1)
|
|
assert addr.ip.startswith('123.123.123.')
|
|
assert 8333 <= addr.port < 8343
|
|
self.addrv2_received_and_checked = True
|
|
|
|
def wait_for_addrv2(self):
|
|
wait_until(lambda: "addrv2" in self.last_message)
|
|
|
|
|
|
class AddrTest(BitcoinTestFramework):
|
|
def set_test_params(self):
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 1
|
|
|
|
def run_test(self):
|
|
ADDRS = []
|
|
for i in range(10):
|
|
addr = CAddress()
|
|
addr.time = int(self.mocktime) + i
|
|
addr.nServices = NODE_NETWORK
|
|
addr.ip = "123.123.123.{}".format(i % 256)
|
|
addr.port = 8333 + i
|
|
ADDRS.append(addr)
|
|
|
|
self.log.info('Create connection that sends addrv2 messages')
|
|
addr_source = self.nodes[0].add_p2p_connection(P2PInterface())
|
|
|
|
msg = msg_addrv2()
|
|
|
|
self.log.info('Send too-large addrv2 message')
|
|
msg.addrs = ADDRS * 101
|
|
with self.nodes[0].assert_debug_log(['addrv2 message size = 1010']):
|
|
addr_source.send_and_ping(msg)
|
|
|
|
self.nodes[0].disconnect_p2ps()
|
|
|
|
self.log.info('Check that addrv2 message content is relayed and added to addrman')
|
|
addr_source = self.nodes[0].add_p2p_connection(P2PInterface())
|
|
addr_receiver = self.nodes[0].add_p2p_connection(AddrReceiver())
|
|
|
|
msg.addrs = ADDRS
|
|
with self.nodes[0].assert_debug_log([
|
|
'Added 10 addresses from 127.0.0.1: 0 tried',
|
|
'received: addrv2 (131 bytes) peer=1',
|
|
]):
|
|
addr_source.send_and_ping(msg)
|
|
|
|
# Wait until "Added ..." before bumping mocktime to make sure addv2 is (almost) fully processed
|
|
with self.nodes[0].assert_debug_log([
|
|
'sending addrv2 (131 bytes) peer=2',
|
|
]):
|
|
self.bump_mocktime(30 * 60)
|
|
addr_receiver.wait_for_addrv2()
|
|
|
|
assert addr_receiver.addrv2_received_and_checked
|
|
|
|
self.nodes[0].disconnect_p2ps()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
AddrTest().main()
|