mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
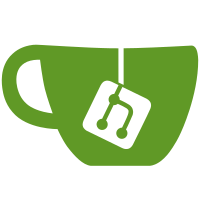
ab9aca2bdf [rpc] add 'getnewaddress' hint to 'generatetoaddress' help text. (John Newbery) c9f02955b2 [wallet] Deprecate the generate RPC method (John Newbery) aab81720de [tests] Add generate method to TestNode (John Newbery) c269209336 [tests] Small fixups before deprecating generate (John Newbery) Pull request description: Deprecates the `generate` RPC method. For concept discussion, see #14299. Fixes #14299. Tree-SHA512: 16a3b8b742932e4f0476c06b23de07a34d9d215b41d9272c1c9d1e39966b0c2406f17c5ab3cc568947620c08171ebe5eb74fd7ed4b62151363e305ee2937cc80
106 lines
3.8 KiB
Python
Executable File
106 lines
3.8 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2014-2015 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
# Exercise the wallet keypool, and interaction with wallet encryption/locking
|
|
|
|
# Add python-bitcoinrpc to module search path:
|
|
|
|
import time
|
|
|
|
from test_framework.authproxy import JSONRPCException
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import assert_equal, assert_raises_rpc_error
|
|
|
|
class KeyPoolTest(BitcoinTestFramework):
|
|
|
|
def set_test_params(self):
|
|
self.setup_clean_chain = True
|
|
self.num_nodes = 1
|
|
self.extra_args = [['-usehd=1']]
|
|
|
|
def skip_test_if_missing_module(self):
|
|
self.skip_if_no_wallet()
|
|
|
|
def run_test(self):
|
|
nodes = self.nodes
|
|
addr_before_encrypting = nodes[0].getnewaddress()
|
|
addr_before_encrypting_data = nodes[0].getaddressinfo(addr_before_encrypting)
|
|
wallet_info_old = nodes[0].getwalletinfo()
|
|
assert addr_before_encrypting_data['hdchainid'] == wallet_info_old['hdchainid']
|
|
|
|
# Encrypt wallet and wait to terminate
|
|
nodes[0].encryptwallet('test')
|
|
# Keep creating keys
|
|
addr = nodes[0].getnewaddress()
|
|
addr_data = nodes[0].getaddressinfo(addr)
|
|
wallet_info = nodes[0].getwalletinfo()
|
|
assert addr_before_encrypting_data['hdchainid'] == wallet_info['hdchainid']
|
|
assert addr_data['hdchainid'] == wallet_info['hdchainid']
|
|
|
|
try:
|
|
addr = nodes[0].getnewaddress()
|
|
raise AssertionError('Keypool should be exhausted after one address')
|
|
except JSONRPCException as e:
|
|
assert e.error['code']==-12
|
|
|
|
# put six (plus 2) new keys in the keypool (100% external-, +100% internal-keys, 1 in min)
|
|
nodes[0].walletpassphrase('test', 12000)
|
|
nodes[0].keypoolrefill(6)
|
|
nodes[0].walletlock()
|
|
wi = nodes[0].getwalletinfo()
|
|
assert_equal(wi['keypoolsize_hd_internal'], 6)
|
|
assert_equal(wi['keypoolsize'], 6)
|
|
|
|
# drain the internal keys
|
|
nodes[0].getrawchangeaddress()
|
|
nodes[0].getrawchangeaddress()
|
|
nodes[0].getrawchangeaddress()
|
|
nodes[0].getrawchangeaddress()
|
|
nodes[0].getrawchangeaddress()
|
|
nodes[0].getrawchangeaddress()
|
|
# the next one should fail
|
|
try:
|
|
nodes[0].getrawchangeaddress()
|
|
raise AssertionError('Keypool should be exhausted after six addresses')
|
|
except JSONRPCException as e:
|
|
assert e.error['code']==-12
|
|
|
|
addr = set()
|
|
# drain the external keys
|
|
addr.add(nodes[0].getnewaddress())
|
|
addr.add(nodes[0].getnewaddress())
|
|
addr.add(nodes[0].getnewaddress())
|
|
addr.add(nodes[0].getnewaddress())
|
|
addr.add(nodes[0].getnewaddress())
|
|
addr.add(nodes[0].getnewaddress())
|
|
assert len(addr) == 6
|
|
# the next one should fail
|
|
try:
|
|
addr = nodes[0].getnewaddress()
|
|
raise AssertionError('Keypool should be exhausted after six addresses')
|
|
except JSONRPCException as e:
|
|
assert e.error['code']==-12
|
|
|
|
# refill keypool with three new addresses
|
|
nodes[0].walletpassphrase('test', 1)
|
|
nodes[0].keypoolrefill(3)
|
|
# test walletpassphrase timeout
|
|
time.sleep(1.1)
|
|
assert_equal(nodes[0].getwalletinfo()["unlocked_until"], 0)
|
|
|
|
# drain the keypool
|
|
for _ in range(3):
|
|
nodes[0].getnewaddress()
|
|
assert_raises_rpc_error(-12, "Keypool ran out", nodes[0].getnewaddress)
|
|
|
|
nodes[0].walletpassphrase('test', 100)
|
|
nodes[0].keypoolrefill(100)
|
|
wi = nodes[0].getwalletinfo()
|
|
assert_equal(wi['keypoolsize_hd_internal'], 100)
|
|
assert_equal(wi['keypoolsize'], 100)
|
|
|
|
if __name__ == '__main__':
|
|
KeyPoolTest().main()
|