mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 04:22:55 +01:00
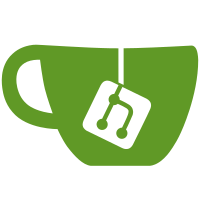
* Merge #11796: [tests] Functional test naming convention5fecd84
[tests] Remove redundant import in blocktools.py test (Anthony Towns)9b20bb4
[tests] Check tests conform to naming convention (Anthony Towns)7250b4e
[tests] README.md nit fixes (Anthony Towns)82b2712
[tests] move witness util functions to blocktools.py (John Newbery)1e10854
[tests] [docs] update README for new test naming scheme (John Newbery) Pull request description: Splitting #11774 into two parts -- this part updates the README with the proposed naming convention, and adds some checks to test_runner.py that the number of tests violating the naming convention doesn't increase too much. Idea is this part of the change should not introduce merge conflicts or require much rebasing, so reviews of the complicated bits won't become invalidated too often; while the second part will just be file renames, which will require regular rebasing and will introduce merge conflicts with pending PRs, but can be merged later, and should also be much easier to review, since it will only include relatively trivial changes. Tree-SHA512: b96557d41714addbbfe2aed62fb5a48639eaeb1eb3aba30ac1b3a86bb3cb8d796c6247f9c414c4695c4bf54c0ec9968ac88e2f88fb62483bc1a2f89368f7fc80 * update violation count Signed-off-by: pasta <pasta@dashboost.org> * Merge #11774: [tests] Rename functional tests6f881cc880
[tests] Remove EXPECTED_VIOLATION_COUNT (Anthony Towns)3150b3fea7
[tests] Rename misc functional tests. (Anthony Towns)81b79f2c39
[tests] Rename rpc_* functional tests. (Anthony Towns)61b8f7f273
[tests] Rename p2p_* functional tests. (Anthony Towns)90600bc7db
[tests] Rename wallet_* functional tests. (Anthony Towns)ca6523d0c8
[tests] Rename feature_* functional tests. (Anthony Towns) Pull request description: This PR changes the functional tests to have a consistent naming scheme: tests for individual RPC methods are named rpc_... tests for interfaces (REST, ZMQ, RPC features) are named interface_... tests that explicitly test the p2p interface are named p2p_... tests for wallet features are named wallet_... tests for mining features are named mining_... tests for mempool behaviour are named mempool_... tests for full features that aren't wallet/mining/mempool are named feature_... Rationale: it's sometimes difficult for new contributors to know what's already covered by existing tests and where new tests should be added. Naming in a consistent fashion makes it easier to see what's already covered at a glance. Tree-SHA512: 4246790552d42bbd95f6d5bdf67702b81b3b2c583ce7eaf1fe6d8e254721279b47315973c6e9ae82dad6e4c747f12188160764bf2624c0f8f3b4d39330ec8b16 * rename tests and edit associated strings to align test-suite with test name standards Signed-off-by: pasta <pasta@dashboost.org> * fix grammar in test/functional/test_runner.py Co-authored-by: dustinface <35775977+xdustinface@users.noreply.github.com> * ci: Fix excluded test names * rename feature_privatesend.py to rpc_privatesend.py Signed-off-by: pasta <pasta@dashboost.org> Co-authored-by: Wladimir J. van der Laan <laanwj@gmail.com> Co-authored-by: MarcoFalke <falke.marco@gmail.com> Co-authored-by: dustinface <35775977+xdustinface@users.noreply.github.com> Co-authored-by: xdustinface <xdustinfacex@gmail.com>
328 lines
13 KiB
Python
Executable File
328 lines
13 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2015-2020 The Dash Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
import time
|
|
from decimal import Decimal
|
|
|
|
from test_framework.blocktools import get_masternode_payment, create_coinbase, create_block
|
|
from test_framework.mininode import *
|
|
from test_framework.test_framework import DashTestFramework
|
|
from test_framework.util import assert_raises_rpc_error
|
|
|
|
'''
|
|
feature_llmq_is_cl_conflicts.py
|
|
|
|
Checks conflict handling between ChainLocks and InstantSend
|
|
|
|
'''
|
|
|
|
class TestP2PConn(P2PInterface):
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.clsigs = {}
|
|
self.islocks = {}
|
|
|
|
def send_clsig(self, clsig):
|
|
hash = uint256_from_str(hash256(clsig.serialize()))
|
|
self.clsigs[hash] = clsig
|
|
|
|
inv = msg_inv([CInv(29, hash)])
|
|
self.send_message(inv)
|
|
|
|
def send_islock(self, islock):
|
|
hash = uint256_from_str(hash256(islock.serialize()))
|
|
self.islocks[hash] = islock
|
|
|
|
inv = msg_inv([CInv(30, hash)])
|
|
self.send_message(inv)
|
|
|
|
def on_getdata(self, message):
|
|
for inv in message.inv:
|
|
if inv.hash in self.clsigs:
|
|
self.send_message(self.clsigs[inv.hash])
|
|
if inv.hash in self.islocks:
|
|
self.send_message(self.islocks[inv.hash])
|
|
|
|
|
|
class LLMQ_IS_CL_Conflicts(DashTestFramework):
|
|
def set_test_params(self):
|
|
self.set_dash_test_params(4, 3, fast_dip3_enforcement=True)
|
|
self.set_dash_dip8_activation(10)
|
|
#disable_mocktime()
|
|
|
|
def run_test(self):
|
|
|
|
while self.nodes[0].getblockchaininfo()["bip9_softforks"]["dip0008"]["status"] != "active":
|
|
self.nodes[0].generate(10)
|
|
self.sync_blocks(self.nodes, timeout=60*5)
|
|
|
|
self.test_node = self.nodes[0].add_p2p_connection(TestP2PConn())
|
|
network_thread_start()
|
|
self.nodes[0].p2p.wait_for_verack()
|
|
|
|
self.nodes[0].spork("SPORK_17_QUORUM_DKG_ENABLED", 0)
|
|
self.nodes[0].spork("SPORK_19_CHAINLOCKS_ENABLED", 0)
|
|
self.nodes[0].spork("SPORK_2_INSTANTSEND_ENABLED", 0)
|
|
self.nodes[0].spork("SPORK_3_INSTANTSEND_BLOCK_FILTERING", 0)
|
|
self.wait_for_sporks_same()
|
|
|
|
self.mine_quorum()
|
|
|
|
# mine single block, wait for chainlock
|
|
self.nodes[0].generate(1)
|
|
self.wait_for_chainlocked_block_all_nodes(self.nodes[0].getbestblockhash())
|
|
|
|
self.test_chainlock_overrides_islock(False)
|
|
self.test_chainlock_overrides_islock(True)
|
|
self.test_islock_overrides_nonchainlock()
|
|
|
|
def test_chainlock_overrides_islock(self, test_block_conflict):
|
|
# create three raw TXs, they will conflict with each other
|
|
rawtx1 = self.create_raw_tx(self.nodes[0], self.nodes[0], 1, 1, 100)['hex']
|
|
rawtx2 = self.create_raw_tx(self.nodes[0], self.nodes[0], 1, 1, 100)['hex']
|
|
rawtx3 = self.create_raw_tx(self.nodes[0], self.nodes[0], 1, 1, 100)['hex']
|
|
rawtx1_obj = FromHex(CTransaction(), rawtx1)
|
|
rawtx2_obj = FromHex(CTransaction(), rawtx2)
|
|
rawtx3_obj = FromHex(CTransaction(), rawtx3)
|
|
|
|
rawtx1_txid = self.nodes[0].sendrawtransaction(rawtx1)
|
|
rawtx2_txid = encode(hash256(hex_str_to_bytes(rawtx2))[::-1], 'hex_codec').decode('ascii')
|
|
rawtx3_txid = encode(hash256(hex_str_to_bytes(rawtx3))[::-1], 'hex_codec').decode('ascii')
|
|
|
|
# Create a chained TX on top of tx1
|
|
inputs = []
|
|
n = 0
|
|
for out in rawtx1_obj.vout:
|
|
if out.nValue == 100000000:
|
|
inputs.append({"txid": rawtx1_txid, "vout": n})
|
|
n += 1
|
|
rawtx4 = self.nodes[0].createrawtransaction(inputs, {self.nodes[0].getnewaddress(): 0.999})
|
|
rawtx4 = self.nodes[0].signrawtransaction(rawtx4)['hex']
|
|
rawtx4_txid = self.nodes[0].sendrawtransaction(rawtx4)
|
|
|
|
# wait for transactions to propagate
|
|
self.sync_mempools()
|
|
for node in self.nodes:
|
|
self.wait_for_instantlock(rawtx1_txid, node)
|
|
self.wait_for_instantlock(rawtx4_txid, node)
|
|
|
|
block = self.create_block(self.nodes[0], [rawtx2_obj])
|
|
if test_block_conflict:
|
|
# The block shouldn't be accepted/connected but it should be known to node 0 now
|
|
submit_result = self.nodes[0].submitblock(ToHex(block))
|
|
assert(submit_result == "conflict-tx-lock")
|
|
|
|
cl = self.create_chainlock(self.nodes[0].getblockcount() + 1, block.sha256)
|
|
self.test_node.send_clsig(cl)
|
|
|
|
for node in self.nodes:
|
|
self.wait_for_best_chainlock(node, "%064x" % block.sha256)
|
|
|
|
self.sync_blocks()
|
|
|
|
# At this point all nodes should be in sync and have the same "best chainlock"
|
|
|
|
submit_result = self.nodes[1].submitblock(ToHex(block))
|
|
if test_block_conflict:
|
|
# Node 1 should receive the block from node 0 and should not accept it again via submitblock
|
|
assert(submit_result == "duplicate")
|
|
else:
|
|
# The block should get accepted now, and at the same time prune the conflicting ISLOCKs
|
|
assert(submit_result is None)
|
|
|
|
for node in self.nodes:
|
|
self.wait_for_chainlocked_block(node, "%064x" % block.sha256)
|
|
|
|
# Create a chained TX on top of tx2
|
|
inputs = []
|
|
n = 0
|
|
for out in rawtx2_obj.vout:
|
|
if out.nValue == 100000000:
|
|
inputs.append({"txid": rawtx2_txid, "vout": n})
|
|
n += 1
|
|
rawtx5 = self.nodes[0].createrawtransaction(inputs, {self.nodes[0].getnewaddress(): 0.999})
|
|
rawtx5 = self.nodes[0].signrawtransaction(rawtx5)['hex']
|
|
rawtx5_txid = self.nodes[0].sendrawtransaction(rawtx5)
|
|
# wait for the transaction to propagate
|
|
self.sync_mempools()
|
|
for node in self.nodes:
|
|
self.wait_for_instantlock(rawtx5_txid, node)
|
|
|
|
# Lets verify that the ISLOCKs got pruned
|
|
for node in self.nodes:
|
|
assert_raises_rpc_error(-5, "No such mempool or blockchain transaction", node.getrawtransaction, rawtx1_txid, True)
|
|
assert_raises_rpc_error(-5, "No such mempool or blockchain transaction", node.getrawtransaction, rawtx4_txid, True)
|
|
rawtx = node.getrawtransaction(rawtx2_txid, True)
|
|
assert(rawtx['chainlock'])
|
|
assert(rawtx['instantlock'])
|
|
assert(not rawtx['instantlock_internal'])
|
|
|
|
def test_islock_overrides_nonchainlock(self):
|
|
# create two raw TXs, they will conflict with each other
|
|
rawtx1 = self.create_raw_tx(self.nodes[0], self.nodes[0], 1, 1, 100)['hex']
|
|
rawtx2 = self.create_raw_tx(self.nodes[0], self.nodes[0], 1, 1, 100)['hex']
|
|
|
|
rawtx1_txid = encode(hash256(hex_str_to_bytes(rawtx1))[::-1], 'hex_codec').decode('ascii')
|
|
rawtx2_txid = encode(hash256(hex_str_to_bytes(rawtx2))[::-1], 'hex_codec').decode('ascii')
|
|
|
|
# Create an ISLOCK but don't broadcast it yet
|
|
islock = self.create_islock(rawtx2)
|
|
|
|
# Stop enough MNs so that ChainLocks don't work anymore
|
|
for i in range(2):
|
|
self.stop_node(len(self.nodes) - 1)
|
|
self.nodes.pop(len(self.nodes) - 1)
|
|
self.mninfo.pop(len(self.mninfo) - 1)
|
|
|
|
# Send tx1, which will later conflict with the ISLOCK
|
|
self.nodes[0].sendrawtransaction(rawtx1)
|
|
|
|
# fast forward 11 minutes, so that the TX is considered safe and included in the next block
|
|
self.bump_mocktime(int(60 * 11))
|
|
|
|
# Mine the conflicting TX into a block
|
|
good_tip = self.nodes[0].getbestblockhash()
|
|
self.nodes[0].generate(2)
|
|
self.sync_all()
|
|
|
|
# Assert that the conflicting tx got mined and the locked TX is not valid
|
|
assert(self.nodes[0].getrawtransaction(rawtx1_txid, True)['confirmations'] > 0)
|
|
assert_raises_rpc_error(-25, "Missing inputs", self.nodes[0].sendrawtransaction, rawtx2)
|
|
|
|
# Send the ISLOCK, which should result in the last 2 blocks to be invalidated, even though the nodes don't know
|
|
# the locked transaction yet
|
|
self.test_node.send_islock(islock)
|
|
time.sleep(5)
|
|
|
|
assert(self.nodes[0].getbestblockhash() == good_tip)
|
|
assert(self.nodes[1].getbestblockhash() == good_tip)
|
|
|
|
# Send the actual transaction and mine it
|
|
self.nodes[0].sendrawtransaction(rawtx2)
|
|
self.nodes[0].generate(1)
|
|
self.sync_all()
|
|
|
|
assert(self.nodes[0].getrawtransaction(rawtx2_txid, True)['confirmations'] > 0)
|
|
assert(self.nodes[1].getrawtransaction(rawtx2_txid, True)['confirmations'] > 0)
|
|
assert(self.nodes[0].getrawtransaction(rawtx2_txid, True)['instantlock'])
|
|
assert(self.nodes[1].getrawtransaction(rawtx2_txid, True)['instantlock'])
|
|
assert(self.nodes[0].getbestblockhash() != good_tip)
|
|
assert(self.nodes[1].getbestblockhash() != good_tip)
|
|
|
|
def create_block(self, node, vtx=[]):
|
|
bt = node.getblocktemplate()
|
|
height = bt['height']
|
|
tip_hash = bt['previousblockhash']
|
|
|
|
coinbasevalue = bt['coinbasevalue']
|
|
miner_address = node.getnewaddress()
|
|
mn_payee = bt['masternode'][0]['payee']
|
|
|
|
# calculate fees that the block template included (we'll have to remove it from the coinbase as we won't
|
|
# include the template's transactions
|
|
bt_fees = 0
|
|
for tx in bt['transactions']:
|
|
bt_fees += tx['fee']
|
|
|
|
new_fees = 0
|
|
for tx in vtx:
|
|
in_value = 0
|
|
out_value = 0
|
|
for txin in tx.vin:
|
|
txout = node.gettxout("%064x" % txin.prevout.hash, txin.prevout.n, False)
|
|
in_value += int(txout['value'] * COIN)
|
|
for txout in tx.vout:
|
|
out_value += txout.nValue
|
|
new_fees += in_value - out_value
|
|
|
|
# fix fees
|
|
coinbasevalue -= bt_fees
|
|
coinbasevalue += new_fees
|
|
|
|
mn_amount = get_masternode_payment(height, coinbasevalue)
|
|
miner_amount = coinbasevalue - mn_amount
|
|
|
|
outputs = {miner_address: str(Decimal(miner_amount) / COIN)}
|
|
if mn_amount > 0:
|
|
outputs[mn_payee] = str(Decimal(mn_amount) / COIN)
|
|
|
|
coinbase = FromHex(CTransaction(), node.createrawtransaction([], outputs))
|
|
coinbase.vin = create_coinbase(height).vin
|
|
|
|
# We can't really use this one as it would result in invalid merkle roots for masternode lists
|
|
if len(bt['coinbase_payload']) != 0:
|
|
cbtx = FromHex(CCbTx(version=1), bt['coinbase_payload'])
|
|
coinbase.nVersion = 3
|
|
coinbase.nType = 5 # CbTx
|
|
coinbase.vExtraPayload = cbtx.serialize()
|
|
|
|
coinbase.calc_sha256()
|
|
|
|
block = create_block(int(tip_hash, 16), coinbase, nTime=bt['curtime'])
|
|
block.vtx += vtx
|
|
|
|
# Add quorum commitments from template
|
|
for tx in bt['transactions']:
|
|
tx2 = FromHex(CTransaction(), tx['data'])
|
|
if tx2.nType == 6:
|
|
block.vtx.append(tx2)
|
|
|
|
block.hashMerkleRoot = block.calc_merkle_root()
|
|
block.solve()
|
|
return block
|
|
|
|
def create_chainlock(self, height, blockHash):
|
|
request_id = "%064x" % uint256_from_str(hash256(ser_string(b"clsig") + struct.pack("<I", height)))
|
|
message_hash = "%064x" % blockHash
|
|
|
|
for mn in self.mninfo:
|
|
mn.node.quorum('sign', 100, request_id, message_hash)
|
|
|
|
recSig = None
|
|
|
|
t = time.time()
|
|
while time.time() - t < 10:
|
|
try:
|
|
recSig = self.nodes[0].quorum('getrecsig', 100, request_id, message_hash)
|
|
break
|
|
except:
|
|
time.sleep(0.1)
|
|
assert(recSig is not None)
|
|
|
|
clsig = msg_clsig(height, blockHash, hex_str_to_bytes(recSig['sig']))
|
|
return clsig
|
|
|
|
def create_islock(self, hextx):
|
|
tx = FromHex(CTransaction(), hextx)
|
|
tx.rehash()
|
|
|
|
request_id_buf = ser_string(b"islock") + ser_compact_size(len(tx.vin))
|
|
inputs = []
|
|
for txin in tx.vin:
|
|
request_id_buf += txin.prevout.serialize()
|
|
inputs.append(txin.prevout)
|
|
request_id = "%064x" % uint256_from_str(hash256(request_id_buf))
|
|
message_hash = "%064x" % tx.sha256
|
|
|
|
for mn in self.mninfo:
|
|
mn.node.quorum('sign', 100, request_id, message_hash)
|
|
|
|
recSig = None
|
|
|
|
t = time.time()
|
|
while time.time() - t < 10:
|
|
try:
|
|
recSig = self.nodes[0].quorum('getrecsig', 100, request_id, message_hash)
|
|
break
|
|
except:
|
|
time.sleep(0.1)
|
|
assert(recSig is not None)
|
|
|
|
islock = msg_islock(inputs, tx.sha256, hex_str_to_bytes(recSig['sig']))
|
|
return islock
|
|
|
|
|
|
if __name__ == '__main__':
|
|
LLMQ_IS_CL_Conflicts().main()
|