mirror of
https://github.com/dashpay/dash.git
synced 2024-12-24 19:42:46 +01:00
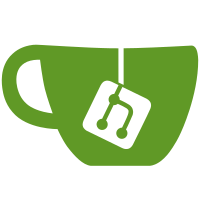
9329803969 wip: fix FromBytesUnchecked (#68) 767713de3d feat: js bindings in camel case (#66) 06df92693a chore(release): bump version (#64) 73593feefd fix: the JS bundle script and bindings (#47) 38a8f768c6 Merge pull request #61 from kittywhiskers/compat_support d9b375145e ci: ensure that CMakeFiles are compatible with LTS-bundled cmake 5ba1b520cc build: restore CMake 3.14.0 compatibility d1c1b66e5f backport: merge bls-signatures#332 (Python 3.11) git-subtree-dir: src/dashbls git-subtree-split: 9329803969fd325dc0d5c9029ab15669d658ed5d
38 lines
1.3 KiB
JavaScript
38 lines
1.3 KiB
JavaScript
// The code manipulation from this file needs to be done for Chrome, as
|
|
// it requires wasm to be loaded asynchronously, and it doesn't
|
|
// work when bundling complex projects. With this solution, wasm is
|
|
// included right into the bundle itself in a form of base64 string
|
|
// and compiled asynchronously, just as Chrome requires
|
|
|
|
const fs = require('fs');
|
|
|
|
const outputPath = './blsjs.js';
|
|
|
|
const wasm = fs.readFileSync('./blsjstmp.wasm');
|
|
const wasmBase = wasm.toString('base64');
|
|
|
|
const codeToPrepend = `
|
|
if (typeof window === "object") {
|
|
var buf = Buffer.from("${wasmBase}", "base64");
|
|
var blob = new Blob([buf], { type: "application/wasm" });
|
|
var wasmUrl = URL.createObjectURL(blob);
|
|
}
|
|
`;
|
|
|
|
const originalSourceCode = fs.readFileSync('./blsjstmp.js', 'utf-8');
|
|
const modifiedSourceCode = originalSourceCode
|
|
.replace(/fetch\(.,/g, "fetch(wasmUrl,");
|
|
|
|
const modifiedSourceBuffer = Buffer.from(modifiedSourceCode, 'utf-8');
|
|
|
|
const bundleFileDescriptor = fs.openSync(outputPath, 'w+');
|
|
|
|
const bufferToPrepend = Buffer.from(codeToPrepend);
|
|
|
|
fs.writeSync(bundleFileDescriptor, bufferToPrepend, 0, bufferToPrepend.length, 0);
|
|
fs.writeSync(bundleFileDescriptor, modifiedSourceBuffer, 0, modifiedSourceBuffer.length, bufferToPrepend.length);
|
|
|
|
fs.close(bundleFileDescriptor, (err) => {
|
|
if (err) throw err;
|
|
});
|