mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 20:42:59 +01:00
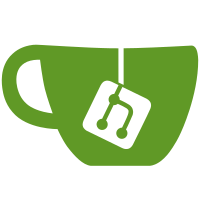
adaa281
Update release notes to include RPC error code changes. (John Newbery)338bf06
Add commenting around JSON error codes (John Newbery)dab804c
Return correct error codes in fundrawtransaction(). (John Newbery)a012087
Return correct error codes in setban(). (John Newbery)960bc7f
Return correct error codes in removeprunedfunds(). (John Newbery)c119096
Return correct error codes in blockchain.cpp. (John Newbery)6d07c62
Return correct error codes in bumpfee(). (John Newbery) Tree-SHA512: 4bb39ad221cd8c83d98ac5d7ad642f3a8c265522720dc86b2eebc70e74439a85b06d6ddcd6a874e879d986511de3ab0878bb7fe58b50cb0546b78913632ea809
82 lines
3.9 KiB
Python
Executable File
82 lines
3.9 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2014-2016 The Bitcoin Core developers
|
|
# Distributed under the MIT software license, see the accompanying
|
|
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
"""Test node handling."""
|
|
|
|
from test_framework.test_framework import BitcoinTestFramework
|
|
from test_framework.util import *
|
|
|
|
import urllib.parse
|
|
|
|
class NodeHandlingTest (BitcoinTestFramework):
|
|
|
|
def __init__(self):
|
|
super().__init__()
|
|
self.num_nodes = 4
|
|
self.setup_clean_chain = False
|
|
|
|
def run_test(self):
|
|
###########################
|
|
# setban/listbanned tests #
|
|
###########################
|
|
assert_equal(len(self.nodes[2].getpeerinfo()), 4) #we should have 4 nodes at this point
|
|
self.nodes[2].setban("127.0.0.1", "add")
|
|
time.sleep(3) #wait till the nodes are disconected
|
|
assert_equal(len(self.nodes[2].getpeerinfo()), 0) #all nodes must be disconnected at this point
|
|
assert_equal(len(self.nodes[2].listbanned()), 1)
|
|
self.nodes[2].clearbanned()
|
|
assert_equal(len(self.nodes[2].listbanned()), 0)
|
|
self.nodes[2].setban("127.0.0.0/24", "add")
|
|
assert_equal(len(self.nodes[2].listbanned()), 1)
|
|
# This will throw an exception because 127.0.0.1 is within range 127.0.0.0/24
|
|
assert_raises_jsonrpc(-23, "IP/Subnet already banned", self.nodes[2].setban, "127.0.0.1", "add")
|
|
# This will throw an exception because 127.0.0.1/42 is not a real subnet
|
|
assert_raises_jsonrpc(-30, "Error: Invalid IP/Subnet", self.nodes[2].setban, "127.0.0.1/42", "add")
|
|
assert_equal(len(self.nodes[2].listbanned()), 1) #still only one banned ip because 127.0.0.1 is within the range of 127.0.0.0/24
|
|
# This will throw an exception because 127.0.0.1 was not added above
|
|
assert_raises_jsonrpc(-30, "Error: Unban failed", self.nodes[2].setban, "127.0.0.1", "remove")
|
|
assert_equal(len(self.nodes[2].listbanned()), 1)
|
|
self.nodes[2].setban("127.0.0.0/24", "remove")
|
|
assert_equal(len(self.nodes[2].listbanned()), 0)
|
|
self.nodes[2].clearbanned()
|
|
assert_equal(len(self.nodes[2].listbanned()), 0)
|
|
|
|
##test persisted banlist
|
|
self.nodes[2].setban("127.0.0.0/32", "add")
|
|
self.nodes[2].setban("127.0.0.0/24", "add")
|
|
self.nodes[2].setban("192.168.0.1", "add", 1) #ban for 1 seconds
|
|
self.nodes[2].setban("2001:4d48:ac57:400:cacf:e9ff:fe1d:9c63/19", "add", 1000) #ban for 1000 seconds
|
|
listBeforeShutdown = self.nodes[2].listbanned()
|
|
assert_equal("192.168.0.1/32", listBeforeShutdown[2]['address']) #must be here
|
|
set_mocktime(get_mocktime() + 2) #make 100% sure we expired 192.168.0.1 node time
|
|
set_node_times(self.nodes, get_mocktime()) #make 100% sure we expired 192.168.0.1 node time
|
|
|
|
#stop node
|
|
stop_node(self.nodes[2], 2)
|
|
|
|
self.nodes[2] = start_node(2, self.options.tmpdir)
|
|
listAfterShutdown = self.nodes[2].listbanned()
|
|
assert_equal("127.0.0.0/24", listAfterShutdown[0]['address'])
|
|
assert_equal("127.0.0.0/32", listAfterShutdown[1]['address'])
|
|
assert_equal("/19" in listAfterShutdown[2]['address'], True)
|
|
|
|
###########################
|
|
# RPC disconnectnode test #
|
|
###########################
|
|
url = urllib.parse.urlparse(self.nodes[1].url)
|
|
self.nodes[0].disconnectnode(url.hostname+":"+str(p2p_port(1)))
|
|
time.sleep(2) #disconnecting a node needs a little bit of time
|
|
for node in self.nodes[0].getpeerinfo():
|
|
assert(node['addr'] != url.hostname+":"+str(p2p_port(1)))
|
|
|
|
connect_nodes_bi(self.nodes,0,1) #reconnect the node
|
|
found = False
|
|
for node in self.nodes[0].getpeerinfo():
|
|
if node['addr'] == url.hostname+":"+str(p2p_port(1)):
|
|
found = True
|
|
assert(found)
|
|
|
|
if __name__ == '__main__':
|
|
NodeHandlingTest ().main ()
|