mirror of
https://github.com/dashpay/dash.git
synced 2024-12-29 13:59:06 +01:00
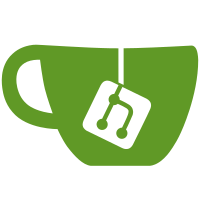
* evo: resolve suggestions given in dash#4696 * evo: add known-good mainnet vectors for IsTriviallyValid() * evo: add artificially malformed vectors for IsTriviallyValid() * evo: add IsTriviallyValid() tests Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com> * Make sure we deserialize the right type of a special tx in GetTxPayload, and debug assert Co-authored-by: UdjinM6 <UdjinM6@users.noreply.github.com>
59 lines
1.8 KiB
C++
59 lines
1.8 KiB
C++
// Copyright (c) 2017-2021 The Dash Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_EVO_CBTX_H
|
|
#define BITCOIN_EVO_CBTX_H
|
|
|
|
#include <primitives/transaction.h>
|
|
#include <univalue.h>
|
|
|
|
class CBlock;
|
|
class CBlockIndex;
|
|
class CCoinsViewCache;
|
|
class CValidationState;
|
|
|
|
// coinbase transaction
|
|
class CCbTx
|
|
{
|
|
public:
|
|
static constexpr auto SPECIALTX_TYPE = TRANSACTION_COINBASE;
|
|
static constexpr uint16_t CURRENT_VERSION = 2;
|
|
|
|
uint16_t nVersion{CURRENT_VERSION};
|
|
int32_t nHeight{0};
|
|
uint256 merkleRootMNList;
|
|
uint256 merkleRootQuorums;
|
|
|
|
SERIALIZE_METHODS(CCbTx, obj)
|
|
{
|
|
READWRITE(obj.nVersion, obj.nHeight, obj.merkleRootMNList);
|
|
|
|
if (obj.nVersion >= 2) {
|
|
READWRITE(obj.merkleRootQuorums);
|
|
}
|
|
}
|
|
|
|
std::string ToString() const;
|
|
|
|
void ToJson(UniValue& obj) const
|
|
{
|
|
obj.clear();
|
|
obj.setObject();
|
|
obj.pushKV("version", (int)nVersion);
|
|
obj.pushKV("height", nHeight);
|
|
obj.pushKV("merkleRootMNList", merkleRootMNList.ToString());
|
|
if (nVersion >= 2) {
|
|
obj.pushKV("merkleRootQuorums", merkleRootQuorums.ToString());
|
|
}
|
|
}
|
|
};
|
|
|
|
bool CheckCbTx(const CTransaction& tx, const CBlockIndex* pindexPrev, CValidationState& state);
|
|
|
|
bool CheckCbTxMerkleRoots(const CBlock& block, const CBlockIndex* pindex, CValidationState& state, const CCoinsViewCache& view);
|
|
bool CalcCbTxMerkleRootMNList(const CBlock& block, const CBlockIndex* pindexPrev, uint256& merkleRootRet, CValidationState& state, const CCoinsViewCache& view);
|
|
bool CalcCbTxMerkleRootQuorums(const CBlock& block, const CBlockIndex* pindexPrev, uint256& merkleRootRet, CValidationState& state);
|
|
|
|
#endif // BITCOIN_EVO_CBTX_H
|