2016-05-06 11:23:48 +02:00
#!/usr/bin/env python3
2020-12-31 18:50:11 +01:00
# Copyright (c) 2014-2020 The Bitcoin Core developers
2023-12-31 01:00:00 +01:00
# Copyright (c) 2014-2024 The Dash Core developers
2014-10-23 03:48:19 +02:00
# Distributed under the MIT software license, see the accompanying
2014-07-08 18:07:23 +02:00
# file COPYING or http://www.opensource.org/licenses/mit-license.php.
2019-01-07 10:55:35 +01:00
""" Base class for RPC testing. """
2017-11-29 19:21:51 +01:00
2018-04-25 15:54:36 +02:00
import configparser
2020-01-04 12:22:41 +01:00
import copy
2020-10-17 17:57:07 +02:00
from _decimal import Decimal , ROUND_DOWN
2017-06-02 11:32:55 +02:00
from enum import Enum
2021-07-04 00:41:23 +02:00
import argparse
2020-05-01 20:57:45 +02:00
import logging
2014-07-08 18:07:23 +02:00
import os
2024-08-25 16:17:32 +02:00
import platform
2017-08-11 18:09:51 +02:00
import pdb
2019-05-14 15:00:55 +02:00
import random
2020-05-01 20:57:45 +02:00
import re
2014-07-08 18:07:23 +02:00
import shutil
2019-12-04 19:22:23 +01:00
import subprocess
2017-05-07 15:13:29 +02:00
import sys
2014-07-08 18:07:23 +02:00
import tempfile
2017-03-22 13:03:26 +01:00
import time
2019-01-23 17:36:51 +01:00
from concurrent . futures import ThreadPoolExecutor
2014-07-08 18:07:23 +02:00
2021-01-31 09:28:17 +01:00
from typing import List
2021-02-25 09:48:28 +01:00
from . address import ADDRESS_BCRT1_P2SH_OP_TRUE
2017-06-29 17:37:19 +02:00
from . authproxy import JSONRPCException
2019-02-15 14:56:43 +01:00
from test_framework . blocktools import TIME_GENESIS_BLOCK
2017-06-29 17:37:19 +02:00
from . import coverage
2020-12-09 20:52:11 +01:00
from . messages import (
hash256 ,
2021-10-05 19:42:34 +02:00
msg_isdlock ,
2020-12-09 20:52:11 +01:00
ser_compact_size ,
ser_string ,
2021-06-24 12:47:04 +02:00
tx_from_hex ,
2020-12-09 20:52:11 +01:00
)
2023-02-14 19:48:33 +01:00
from . script import hash160
2024-01-15 20:35:29 +01:00
from . p2p import NetworkThread
2017-08-15 23:34:07 +02:00
from . test_node import TestNode
2015-10-11 07:41:19 +02:00
from . util import (
2017-05-07 15:13:29 +02:00
PortSeed ,
MAX_NODES ,
2019-06-20 18:37:09 +02:00
assert_equal ,
2017-05-07 15:13:29 +02:00
check_json_precision ,
2019-06-20 18:37:09 +02:00
copy_datadir ,
2019-10-09 18:48:12 +02:00
force_finish_mnsync ,
2018-03-14 14:18:44 +01:00
get_datadir_path ,
2017-05-07 15:13:29 +02:00
initialize_datadir ,
p2p_port ,
set_node_times ,
2019-06-20 18:37:09 +02:00
satoshi_round ,
2019-08-15 22:02:02 +02:00
softfork_active ,
2020-09-03 05:47:08 +02:00
wait_until_helper ,
2023-02-14 19:48:33 +01:00
get_chain_folder , rpc_port ,
2019-10-01 16:14:26 +02:00
)
2014-07-08 18:07:23 +02:00
2019-02-25 17:44:18 +01:00
2017-06-02 11:32:55 +02:00
class TestStatus ( Enum ) :
PASSED = 1
FAILED = 2
SKIPPED = 3
TEST_EXIT_PASSED = 0
TEST_EXIT_FAILED = 1
TEST_EXIT_SKIPPED = 77
2018-11-30 16:30:24 +01:00
TMPDIR_PREFIX = " dash_func_test_ "
2019-08-09 01:14:11 +02:00
2018-09-13 12:33:15 +02:00
class SkipTest ( Exception ) :
""" This exception is raised to skip a test """
def __init__ ( self , message ) :
self . message = message
2021-04-08 22:52:05 +02:00
class BitcoinTestMetaClass ( type ) :
""" Metaclass for BitcoinTestFramework.
Ensures that any attempt to register a subclass of ` BitcoinTestFramework `
adheres to a standard whereby the subclass overrides ` set_test_params ` and
` run_test ` but DOES NOT override either ` __init__ ` or ` main ` . If any of
those standards are violated , a ` ` TypeError ` ` is raised . """
def __new__ ( cls , clsname , bases , dct ) :
if not clsname == ' BitcoinTestFramework ' :
if not ( ' run_test ' in dct and ' set_test_params ' in dct ) :
raise TypeError ( " BitcoinTestFramework subclasses must override "
" ' run_test ' and ' set_test_params ' " )
if ' __init__ ' in dct or ' main ' in dct :
raise TypeError ( " BitcoinTestFramework subclasses may not override "
" ' __init__ ' or ' main ' " )
return super ( ) . __new__ ( cls , clsname , bases , dct )
class BitcoinTestFramework ( metaclass = BitcoinTestMetaClass ) :
2017-05-07 15:13:29 +02:00
""" Base class for a bitcoin test script.
2017-09-01 18:47:13 +02:00
Individual bitcoin test scripts should subclass this class and override the set_test_params ( ) and run_test ( ) methods .
Individual tests can also override the following methods to customize the test setup :
2017-05-07 15:13:29 +02:00
- add_options ( )
- setup_chain ( )
- setup_network ( )
2017-09-01 18:47:13 +02:00
- setup_nodes ( )
2017-05-07 15:13:29 +02:00
2017-09-01 18:47:13 +02:00
The __init__ ( ) and main ( ) methods should not be overridden .
2017-05-07 15:13:29 +02:00
This class also contains various public and private helper methods . """
2020-06-03 16:01:29 +02:00
chain = None # type: str
setup_clean_chain = None # type: bool
2016-05-20 15:16:51 +02:00
def __init__ ( self ) :
2017-09-01 18:47:13 +02:00
""" Sets test framework defaults. Do not override this method. Instead, override the set_test_params() method """
2021-01-31 09:28:17 +01:00
self . chain : str = ' regtest '
self . setup_clean_chain : bool = False
2024-08-30 09:47:15 +02:00
self . disable_mocktime : bool = False
2021-01-31 09:28:17 +01:00
self . nodes : List [ TestNode ] = [ ]
2018-06-29 18:04:25 +02:00
self . network_thread = None
2017-06-29 17:37:19 +02:00
self . mocktime = 0
2018-12-29 20:18:43 +01:00
self . rpc_timeout = 60 # Wait for up to 60 seconds for the RPC server to respond
2019-12-09 19:52:38 +01:00
self . supports_cli = True
2018-03-07 14:51:58 +01:00
self . bind_to_localhost_only = True
2022-11-30 20:23:48 +01:00
self . parse_args ( )
Merge #19077: wallet: Add sqlite as an alternative wallet database and use it for new descriptor wallets
c4a29d0a90b821c443c10891d9326c534d15cf97 Update wallet_multiwallet.py for descriptor and sqlite wallets (Russell Yanofsky)
310b0fde04639b7446efd5c1d2701caa4b991b86 Run dumpwallet for legacy wallets only in wallet_backup.py (Andrew Chow)
6c6639ac9f6e1677da066cf809f9e3fa4d2e7c32 Include sqlite3 in documentation (Andrew Chow)
f023b7cac0eb16d3c1bf40f1f7898b290de4cc73 wallet: Enforce sqlite serialized threading mode (Andrew Chow)
6173269866306058fcb1cc825b9eb681838678ca Set and check the sqlite user version (Andrew Chow)
9d3d2d263c331e3c77b8f0d01ecc9fea0407dd17 Use network magic as sqlite wallet application ID (Andrew Chow)
9af5de3798c49f86f27bb79396e075fb8c1b2381 Use SQLite for descriptor wallets (Andrew Chow)
9b78f3ce8ed1867c37f6b9fff98f74582d44b789 walletutil: Wallets can also be sqlite (Andrew Chow)
ac38a87225be0f1103ff9629d63980550d2f372b Determine wallet file type based on file magic (Andrew Chow)
6045f77003f167bee9a85e2d53f8fc6ff2e297d8 Implement SQLiteDatabase::MakeBatch (Andrew Chow)
727e6b2a4ee5abb7f2dcbc9f7778291908dc28ad Implement SQLiteDatabase::Verify (Andrew Chow)
b4df8fdb19fcded7e6d491ecf0b705cac0ec76a1 Implement SQLiteDatabase::Rewrite (Andrew Chow)
010e3659069e6f97dd7b24483f50ed71042b84b0 Implement SQLiteDatabase::TxnBegin, TxnCommit, and TxnAbort (Andrew Chow)
ac5c1617e7f4273daf24c24da1f6bc5ef5ab2d2b Implement SQLiteDatabase::Backup (Andrew Chow)
f6f9cd6a64842ef23777312f2465e826ca04b886 Implement SQLiteBatch::StartCursor, ReadAtCursor, and CloseCursor (Andrew Chow)
bf90e033f4fe86cfb90492c7e0962278ea3a146d Implement SQLiteBatch::ReadKey, WriteKey, EraseKey, and HasKey (Andrew Chow)
7aa45620e2f2178145a2eca58ccbab3cecff08fb Add SetupSQLStatements (Andrew Chow)
6636a2608a4e5906ee8092d5731595542261e0ad Implement SQLiteBatch::Close (Andrew Chow)
93825352a36456283bf87e39b5888363ee242f21 Implement SQLiteDatabase::Close (Andrew Chow)
a0de83372be83f59015cd3d61af2303b74fb64b5 Implement SQLiteDatabase::Open (Andrew Chow)
3bfa0fe1259280f8c32b41a798c9453b73f89b02 Initialize and Shutdown sqlite3 globals (Andrew Chow)
5a488b3d77326a0d957c1233493061da1b6ec207 Constructors, destructors, and relevant private fields for SQLiteDatabase/Batch (Andrew Chow)
ca8b7e04ab89f99075b093fa248919fd10acbdf7 Implement SQLiteDatabaseVersion (Andrew Chow)
7577b6e1c88a1a7b45ecf5c7f1735bae6f5a82bf Add SQLiteDatabase and SQLiteBatch dummy classes (Andrew Chow)
e87df8258090138d5c22ac46b8602b618620e8a1 Add sqlite to travis and depends (Andrew Chow)
54729f3f4e6765dfded590af5fb28c88331685f8 Add libsqlite3 (Andrew Chow)
Pull request description:
This PR adds a new class `SQLiteDatabase` which is a subclass of `WalletDatabase`. This provides access to a SQLite database that is used to store the wallet records. To keep compatibility with BDB and to complexity of the change down, we don't make use of many SQLite's features. We use it strictly as a key-value store. We create a table `main` which has two columns, `key` and `value` both with the type `blob`.
For new descriptor wallets, we will create a `SQLiteDatabase` instead of a `BerkeleyDatabase`. There is no requirement that all SQLite wallets are descriptor wallets, nor is there a requirement that all descriptor wallets be SQLite wallets. This allows for existing descriptor wallets to work as well as keeping open the option to migrate existing wallets to SQLite.
We keep the name `wallet.dat` for SQLite wallets. We are able to determine which database type to use by searching for specific magic bytes in the `wallet.dat` file. SQLite begins it's files with a null terminated string `SQLite format 3`. BDB has `0x00053162` at byte 12 (note that the byte order of this integer depends on the system endianness). So when we see that there is a `wallet.dat` file that we want to open, we check for the magic bytes to determine which database system to use.
I decided to keep the `wallet.dat` naming to keep things like backup script to continue to function as they won't need to be modified to look for a different file name. It also simplifies a couple of things in the implementation and the tests as `wallet.dat` is something that is specifically being looked for. If we don't want this behavior, then I do have another branch which creates `wallet.sqlite` files instead, but I find that this direction is easier.
ACKs for top commit:
Sjors:
re-utACK c4a29d0a90b821c443c10891d9326c534d15cf97
promag:
Tested ACK c4a29d0a90b821c443c10891d9326c534d15cf97.
fjahr:
reACK c4a29d0a90b821c443c10891d9326c534d15cf97
S3RK:
Re-review ACK c4a29d0a90b821c443c10891d9326c534d15cf97
meshcollider:
re-utACK c4a29d0a90b821c443c10891d9326c534d15cf97
hebasto:
re-ACK c4a29d0a90b821c443c10891d9326c534d15cf97, only rebased since my [previous](https://github.com/bitcoin/bitcoin/pull/19077#pullrequestreview-507743699) review, verified with `git range-diff master d18892dcc c4a29d0a9`.
ryanofsky:
Code review ACK c4a29d0a90b821c443c10891d9326c534d15cf97. I am honestly confused about reasons for locking into `wallet.dat` again when it's so easy now to use a clean format. I assume I'm just very dense, or there's some unstated reason, because the only thing that's been brought up are unrealistic compatibility scenarios (all require actively creating a wallet with non-default descriptor+sqlite option, then trying to using the descriptor+sqlite wallets with old software or scripts and ignoring the results) that we didn't pay attention to with previous PRs like #11687, which did not require any active interfaction.
jonatack:
ACK c4a29d0a90b821c443c10891d9326c534d15cf97, debug builds and test runs after rebase to latest master @ c2c4dbaebd9, some manual testing creating, using, unloading and reloading a few different new sqlite descriptor wallets over several node restarts/shutdowns.
Tree-SHA512: 19145732e5001484947352d3175a660b5102bc6e833f227a55bd41b9b2f4d92737bbed7cead64b75b509decf9e1408cd81c185ab1fb4b90561aee427c4f9751c
2020-10-15 08:20:18 +02:00
self . default_wallet_name = " default_wallet " if self . options . descriptors else " "
2022-11-30 20:23:48 +01:00
self . wallet_data_filename = " wallet.dat "
2020-04-16 11:23:49 +02:00
self . extra_args_from_options = [ ]
2022-11-30 20:23:48 +01:00
# Optional list of wallet names that can be set in set_test_params to
# create and import keys to. If unset, default is len(nodes) *
# [default_wallet_name]. If wallet names are None, wallet creation is
# skipped. If list is truncated, wallet creation is skipped and keys
# are not imported.
self . wallet_names = None
2023-02-14 09:48:28 +01:00
# By default the wallet is not required. Set to true by skip_if_no_wallet().
# When False, we ignore wallet_names regardless of what it is.
self . requires_wallet = False
2017-09-01 18:47:13 +02:00
self . set_test_params ( )
2022-11-30 20:24:02 +01:00
assert self . wallet_names is None or len ( self . wallet_names ) < = self . num_nodes
2023-05-24 19:38:33 +02:00
if self . options . timeout_scale != 1 :
print ( " DEPRECATED: --timeoutscale option is no longer available, please use --timeout-factor instead " )
if self . options . timeout_factor == 1 :
self . options . timeout_factor = self . options . timeout_scale
2020-05-19 02:00:24 +02:00
if self . options . timeout_factor == 0 :
self . options . timeout_factor = 99999
self . rpc_timeout = int ( self . rpc_timeout * self . options . timeout_factor ) # optionally, increase timeout by a factor
2014-07-08 18:07:23 +02:00
def main ( self ) :
2017-09-01 18:47:13 +02:00
""" Main function. This should not be overridden by the subclass test scripts. """
2014-07-08 18:07:23 +02:00
2019-11-04 20:52:51 +01:00
assert hasattr ( self , " num_nodes " ) , " Test must set self.num_nodes in set_test_params() "
try :
self . setup ( )
self . run_test ( )
except JSONRPCException :
self . log . exception ( " JSONRPC error " )
self . success = TestStatus . FAILED
except SkipTest as e :
self . log . warning ( " Test Skipped: %s " % e . message )
self . success = TestStatus . SKIPPED
except AssertionError :
self . log . exception ( " Assertion failed " )
self . success = TestStatus . FAILED
except KeyError :
self . log . exception ( " Key error " )
self . success = TestStatus . FAILED
2019-12-04 19:22:23 +01:00
except subprocess . CalledProcessError as e :
self . log . exception ( " Called Process failed with ' {} ' " . format ( e . output ) )
self . success = TestStatus . FAILED
2019-11-04 20:52:51 +01:00
except Exception :
self . log . exception ( " Unexpected exception caught during testing " )
self . success = TestStatus . FAILED
except KeyboardInterrupt :
self . log . warning ( " Exiting after keyboard interrupt " )
self . success = TestStatus . FAILED
finally :
exit_code = self . shutdown ( )
sys . exit ( exit_code )
def parse_args ( self ) :
2020-05-22 12:29:09 +02:00
previous_releases_path = os . getenv ( " PREVIOUS_RELEASES_DIR " ) or os . getcwd ( ) + " /releases "
2021-07-04 00:41:23 +02:00
parser = argparse . ArgumentParser ( usage = " %(prog)s [options] " )
parser . add_argument ( " --nocleanup " , dest = " nocleanup " , default = False , action = " store_true " ,
help = " Leave dashds and test.* datadir on exit or error " )
parser . add_argument ( " --noshutdown " , dest = " noshutdown " , default = False , action = " store_true " ,
help = " Don ' t stop dashds after the test execution " )
parser . add_argument ( " --cachedir " , dest = " cachedir " , default = os . path . abspath ( os . path . dirname ( os . path . realpath ( __file__ ) ) + " /../../cache " ) ,
help = " Directory for caching pregenerated datadirs (default: %(default)s ) " )
parser . add_argument ( " --tmpdir " , dest = " tmpdir " , help = " Root directory for datadirs " )
parser . add_argument ( " -l " , " --loglevel " , dest = " loglevel " , default = " INFO " ,
help = " log events at this level and higher to the console. Can be set to DEBUG, INFO, WARNING, ERROR or CRITICAL. Passing --loglevel DEBUG will output all logs to console. Note that logs at all levels are always written to the test_framework.log file in the temporary test directory. " )
parser . add_argument ( " --tracerpc " , dest = " trace_rpc " , default = False , action = " store_true " ,
help = " Print out all RPC calls as they are made " )
parser . add_argument ( " --portseed " , dest = " port_seed " , default = os . getpid ( ) , type = int ,
help = " The seed to use for assigning port numbers (default: current process id) " )
2020-05-22 12:29:09 +02:00
parser . add_argument ( " --previous-releases " , dest = " prev_releases " , action = " store_true " ,
default = os . path . isdir ( previous_releases_path ) and bool ( os . listdir ( previous_releases_path ) ) ,
help = " Force test of previous releases (default: %(default)s ) " )
2021-07-04 00:41:23 +02:00
parser . add_argument ( " --coveragedir " , dest = " coveragedir " ,
help = " Write tested RPC commands into this directory " )
parser . add_argument ( " --configfile " , dest = " configfile " ,
default = os . path . abspath ( os . path . dirname ( os . path . realpath ( __file__ ) ) + " /../../config.ini " ) ,
help = " Location of the test framework config file (default: %(default)s ) " )
parser . add_argument ( " --pdbonfailure " , dest = " pdbonfailure " , default = False , action = " store_true " ,
help = " Attach a python debugger if test fails " )
parser . add_argument ( " --usecli " , dest = " usecli " , default = False , action = " store_true " ,
help = " use dash-cli instead of RPC for all commands " )
parser . add_argument ( " --dashd-arg " , dest = " dashd_extra_args " , default = [ ] , action = " append " ,
help = " Pass extra args to all dashd instances " )
parser . add_argument ( " --timeoutscale " , dest = " timeout_scale " , default = 1 , type = int ,
2023-05-24 19:38:33 +02:00
help = argparse . SUPPRESS )
Merge #14519: tests: add utility to easily profile node performance with perf
13782b8ba8 docs: add perf section to developer docs (James O'Beirne)
58180b5fd4 tests: add utility to easily profile node performance with perf (James O'Beirne)
Pull request description:
Adds a context manager to easily (and selectively) profile node performance during functional test execution using `perf`.
While writing some tests, I encountered some odd bitcoind slowness. I wrote up a utility (`TestNode.profile_with_perf`) that generates performance diagnostics for a node by running `perf` during the execution of a particular region of test code.
`perf` usage is detailed in the excellent (and sadly unmerged) https://github.com/bitcoin/bitcoin/pull/12649; all due props to @eklitzke.
### Example
```python
with node.profile_with_perf("large-msgs"):
for i in range(200):
node.p2p.send_message(some_large_msg)
node.p2p.sync_with_ping()
```
This generates a perf data file in the test node's datadir (`/tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data`).
Running `perf report` generates nice output about where the node spent most of its time while running that part of the test:
```bash
$ perf report -i /tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data --stdio \
| c++filt \
| less
# To display the perf.data header info, please use --header/--header-only options.
#
#
# Total Lost Samples: 0
#
# Samples: 135 of event 'cycles:pp'
# Event count (approx.): 1458205679493582
#
# Children Self Command Shared Object Symbol
# ........ ........ ............... ................... ........................................................................................................................................................................................................................................................................
#
70.14% 0.00% bitcoin-net bitcoind [.] CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
|
---CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
70.14% 0.00% bitcoin-net bitcoind [.] CNetMessage::readData(char const*, unsigned int)
|
---CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
35.52% 0.00% bitcoin-net bitcoind [.] std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
|
---std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
...
```
Tree-SHA512: 9ac4ceaa88818d5eca00994e8e3c8ad42ae019550d6583972a0a4f7b0c4f61032e3d0c476b4ae58756bc5eb8f8015a19a7fc26c095bd588f31d49a37ed0c6b3e
2019-02-05 23:40:11 +01:00
parser . add_argument ( " --perf " , dest = " perf " , default = False , action = " store_true " ,
help = " profile running nodes with perf for the duration of the test " )
Merge #17633: tests: Add option --valgrind to run the functional tests under Valgrind
5db506ba5943868cc2c845f717508739b7f05714 tests: Add option --valgrind to run nodes under valgrind in the functional tests (practicalswift)
Pull request description:
What is better than fixing bugs? Fixing entire bug classes of course! :)
Add option `--valgrind` to run the functional tests under Valgrind.
Regular functional testing under Valgrind would have caught many of the uninitialized reads we've seen historically.
Let's kill this bug class once and for all: let's never use an uninitialized value ever again. Or at least not one that would be triggered by running the functional tests! :)
My hope is that this addition will make it super-easy to run the functional tests under Valgrind and thus increase the probability of people making use of it :)
Hopefully `test/functional/test_runner.py --valgrind` will become a natural part of the pre-release QA process.
**Usage:**
```
$ test/functional/test_runner.py --help
…
--valgrind run nodes under the valgrind memory error detector:
expect at least a ~10x slowdown, valgrind 3.14 or
later required
```
**Live demo:**
First, let's re-introduce a memory bug by reverting the recent P2P uninitialized read bug fix from PR #17624 ("net: Fix an uninitialized read in ProcessMessage(…, "tx", …) when receiving a transaction we already have").
```
$ git diff
diff --git a/src/consensus/validation.h b/src/consensus/validation.h
index 3401eb64c..940adea33 100644
--- a/src/consensus/validation.h
+++ b/src/consensus/validation.h
@@ -114,7 +114,7 @@ inline ValidationState::~ValidationState() {};
class TxValidationState : public ValidationState {
private:
- TxValidationResult m_result = TxValidationResult::TX_RESULT_UNSET;
+ TxValidationResult m_result;
public:
bool Invalid(TxValidationResult result,
const std::string &reject_reason="",
```
Second, let's test as normal without Valgrind:
```
$ test/functional/p2p_segwit.py -l INFO
2019-11-28T09:30:42.810000Z TestFramework (INFO): Initializing test directory /tmp/bitcoin_func_test__fc8q3qo
…
2019-11-28T09:31:57.187000Z TestFramework (INFO): Subtest: test_non_standard_witness_blinding (Segwit active = True)
…
2019-11-28T09:32:08.265000Z TestFramework (INFO): Tests successful
```
Third, let's test with `--valgrind` and see if the test fail (as we expect) when the unitialized value is used:
```
$ test/functional/p2p_segwit.py -l INFO --valgrind
2019-11-28T09:32:33.018000Z TestFramework (INFO): Initializing test directory /tmp/bitcoin_func_test_gtjecx2l
…
2019-11-28T09:40:36.702000Z TestFramework (INFO): Subtest: test_non_standard_witness_blinding (Segwit active = True)
2019-11-28T09:40:37.813000Z TestFramework (ERROR): Assertion failed
ConnectionRefusedError: [Errno 111] Connection refused
```
ACKs for top commit:
MarcoFalke:
ACK 5db506ba5943868cc2c845f717508739b7f05714
jonatack:
ACK 5db506ba5943868cc2c845f717508739b7f05714
Tree-SHA512: 2eaecacf4da166febad88b2a8ee6d7ac2bcd38d4c1892ca39516b6343e8f8c8814edf5eaf14c90f11a069a0389d24f0713076112ac284de987e72fc5f6cc3795
2019-12-10 19:30:05 +01:00
parser . add_argument ( " --valgrind " , dest = " valgrind " , default = False , action = " store_true " ,
help = " run nodes under the valgrind memory error detector: expect at least a ~10x slowdown, valgrind 3.14 or later required " )
2019-05-14 15:00:55 +02:00
parser . add_argument ( " --randomseed " , type = int ,
help = " set a random seed for deterministically reproducing a previous test run " )
2020-05-19 02:00:24 +02:00
parser . add_argument ( ' --timeout-factor ' , dest = " timeout_factor " , type = float , default = 1.0 , help = ' adjust test timeouts by a factor. Setting it to 0 disables all timeouts ' )
2020-11-02 16:54:06 +01:00
group = parser . add_mutually_exclusive_group ( )
2021-02-05 14:17:04 +01:00
group . add_argument ( " --descriptors " , action = ' store_const ' , const = True ,
2020-11-02 16:54:06 +01:00
help = " Run test using a descriptor wallet " , dest = ' descriptors ' )
2021-02-05 14:17:04 +01:00
group . add_argument ( " --legacy-wallet " , action = ' store_const ' , const = False ,
2020-11-02 16:54:06 +01:00
help = " Run test using legacy wallets " , dest = ' descriptors ' )
2023-02-14 09:59:39 +01:00
2014-07-08 18:07:23 +02:00
self . add_options ( parser )
2023-02-14 09:59:39 +01:00
self . options = parser . parse_args ( )
2020-05-22 12:29:09 +02:00
self . options . previous_releases_path = previous_releases_path
2023-02-14 09:59:39 +01:00
config = configparser . ConfigParser ( )
config . read_file ( open ( self . options . configfile ) )
self . config = config
Merge bitcoin/bitcoin#22201: test: Fix TestShell to allow running in Jupyter Notebook
168b6c317ca054c1287c36be532964e861f44266 add dummy file param to fix jupyter (Josiah Baker)
Pull request description:
this fixes argparse to use `parse_known_args`. previously, if an unknown argument was passed, argparse would fail with an `unrecognized arguments: %s` error.
## why
the documentation mentions being able to run `TestShell` in a REPL interpreter or a jupyter notebook. when i tried to run inside a jupyter notebook, i got the following error:
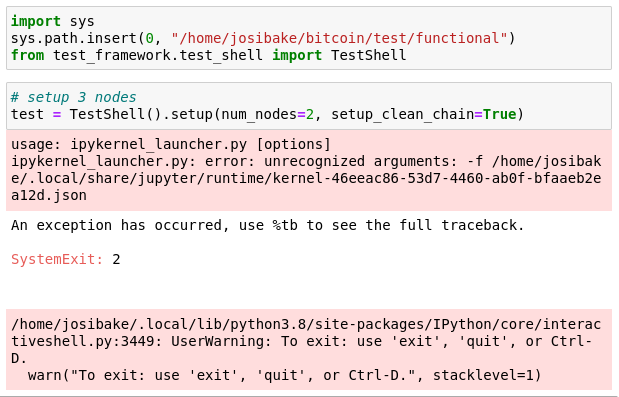
this was due to the notebook passing the filename of the notebook as an argument. this is a known problem with notebooks and argparse, documented here: https://stackoverflow.com/questions/48796169/how-to-fix-ipykernel-launcher-py-error-unrecognized-arguments-in-jupyter
## testing
to test, make sure you have jupyter notebooks installed. you can do this by running:
```
pip install notebook
```
or following instructions from [here](https://jupyterlab.readthedocs.io/en/stable/getting_started/installation.html).
once installed, start a notebook (`jupyter notebook`), launch a python3 kernel and run the following snippet:
```python
import sys
# make sure this is the path for your system
sys.path.insert(0, "/path/to/bitcoin/test/functional")
from test_framework.test_shell import TestShell
test = TestShell().setup(num_nodes=2, setup_clean_chain=True)
```
you should see the following output, without errors:
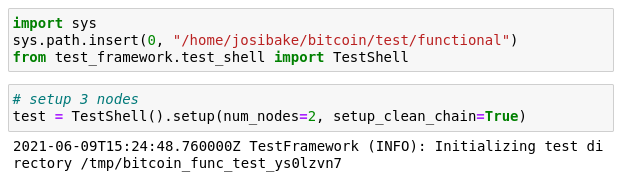
if you are unfamiliar with notebooks, here is a short guide on using them: https://jupyter.readthedocs.io/en/latest/running.html
ACKs for top commit:
MarcoFalke:
review ACK 168b6c317ca054c1287c36be532964e861f44266
jamesob:
crACK https://github.com/bitcoin/bitcoin/pull/22201/commits/168b6c317ca054c1287c36be532964e861f44266
practicalswift:
cr ACK 168b6c317ca054c1287c36be532964e861f44266
Tree-SHA512: 4fee1563bf64a1cf9009934182412446cde03badf2f19553b78ad2cb3ceb0e5e085a5db41ed440473494ac047f04641311ecbba3948761c6553d0ca4b54937b4
2021-06-22 08:11:14 +02:00
# Running TestShell in a Jupyter notebook causes an additional -f argument
# To keep TestShell from failing with an "unrecognized argument" error, we add a dummy "-f" argument
# source: https://stackoverflow.com/questions/48796169/how-to-fix-ipykernel-launcher-py-error-unrecognized-arguments-in-jupyter/56349168#56349168
parser . add_argument ( " -f " , " --fff " , help = " a dummy argument to fool ipython " , default = " 1 " )
2014-07-08 18:07:23 +02:00
2021-02-05 14:17:04 +01:00
if self . options . descriptors is None :
# Prefer BDB unless it isn't available
if self . is_bdb_compiled ( ) :
self . options . descriptors = False
elif self . is_sqlite_compiled ( ) :
self . options . descriptors = True
else :
# If neither are compiled, tests requiring a wallet will be skipped and the value of self.options.descriptors won't matter
# It still needs to exist and be None in order for tests to work however.
self . options . descriptors = None
2022-06-10 12:37:19 +02:00
PortSeed . n = self . options . port_seed
2019-11-04 20:52:51 +01:00
def setup ( self ) :
""" Call this method to start up the test framework object with options set. """
2014-07-08 18:07:23 +02:00
check_json_precision ( )
2017-10-18 16:52:44 +02:00
self . options . cachedir = os . path . abspath ( self . options . cachedir )
2023-02-14 09:59:39 +01:00
config = self . config
2020-05-16 12:16:45 +02:00
fname_bitcoind = os . path . join (
config [ " environment " ] [ " BUILDDIR " ] ,
" src " ,
2020-05-22 12:29:09 +02:00
" dashd " + config [ " environment " ] [ " EXEEXT " ] ,
2020-05-16 12:16:45 +02:00
)
fname_bitcoincli = os . path . join (
config [ " environment " ] [ " BUILDDIR " ] ,
" src " ,
2020-05-22 12:29:09 +02:00
" dash-cli " + config [ " environment " ] [ " EXEEXT " ] ,
2020-05-16 12:16:45 +02:00
)
self . options . bitcoind = os . getenv ( " BITCOIND " , default = fname_bitcoind )
self . options . bitcoincli = os . getenv ( " BITCOINCLI " , default = fname_bitcoincli )
2018-04-25 15:54:36 +02:00
2020-04-16 11:23:49 +02:00
self . extra_args_from_options = self . options . dashd_extra_args
2018-06-01 10:56:53 +02:00
os . environ [ ' PATH ' ] = os . pathsep . join ( [
os . path . join ( config [ ' environment ' ] [ ' BUILDDIR ' ] , ' src ' ) ,
2020-05-01 20:57:45 +02:00
os . path . join ( config [ ' environment ' ] [ ' BUILDDIR ' ] , ' src ' , ' qt ' ) , os . environ [ ' PATH ' ]
2018-06-01 10:56:53 +02:00
] )
2018-05-09 10:41:40 +02:00
2017-03-09 21:16:20 +01:00
# Set up temp directory and start logging
2017-05-22 08:59:11 +02:00
if self . options . tmpdir :
2017-10-18 16:52:44 +02:00
self . options . tmpdir = os . path . abspath ( self . options . tmpdir )
2017-05-22 08:59:11 +02:00
os . makedirs ( self . options . tmpdir , exist_ok = False )
else :
2018-11-30 16:30:24 +01:00
self . options . tmpdir = tempfile . mkdtemp ( prefix = TMPDIR_PREFIX )
2017-03-09 21:16:20 +01:00
self . _start_logging ( )
2019-05-14 15:00:55 +02:00
# Seed the PRNG. Note that test runs are reproducible if and only if
# a single thread accesses the PRNG. For more information, see
# https://docs.python.org/3/library/random.html#notes-on-reproducibility.
# The network thread shouldn't access random. If we need to change the
# network thread to access randomness, it should instantiate its own
# random.Random object.
seed = self . options . randomseed
if seed is None :
seed = random . randrange ( sys . maxsize )
else :
self . log . debug ( " User supplied random seed {} " . format ( seed ) )
random . seed ( seed )
self . log . debug ( " PRNG seed is: {} " . format ( seed ) )
2018-06-29 18:04:25 +02:00
self . log . debug ( ' Setting up network thread ' )
self . network_thread = NetworkThread ( )
self . network_thread . start ( )
2019-11-04 20:52:51 +01:00
if self . options . usecli :
if not self . supports_cli :
raise SkipTest ( " --usecli specified but test does not support using CLI " )
self . skip_if_no_cli ( )
self . skip_test_if_missing_module ( )
self . setup_chain ( )
self . setup_network ( )
2017-03-09 21:16:20 +01:00
2019-11-04 20:52:51 +01:00
self . success = TestStatus . PASSED
2014-07-08 18:07:23 +02:00
2019-11-04 20:52:51 +01:00
def shutdown ( self ) :
""" Call this method to shut down the test framework object. """
if self . success == TestStatus . FAILED and self . options . pdbonfailure :
2017-08-11 18:09:51 +02:00
print ( " Testcase failed. Attaching python debugger. Enter ? for help " )
pdb . set_trace ( )
2018-06-29 18:04:25 +02:00
self . log . debug ( ' Closing down network thread ' )
self . network_thread . close ( )
2015-04-23 14:19:00 +02:00
if not self . options . noshutdown :
2017-03-09 21:16:20 +01:00
self . log . info ( " Stopping nodes " )
2019-02-21 19:37:16 +01:00
try :
2017-06-02 11:32:55 +02:00
if self . nodes :
self . stop_nodes ( )
2020-09-27 07:43:00 +02:00
except BaseException :
2019-11-04 20:52:51 +01:00
self . success = TestStatus . FAILED
2019-03-08 09:05:00 +01:00
self . log . exception ( " Unexpected exception caught during shutdown " )
2015-04-23 14:19:00 +02:00
else :
2018-04-08 17:05:44 +02:00
for node in self . nodes :
node . cleanup_on_exit = False
2017-03-09 21:16:20 +01:00
self . log . info ( " Note: dashds were not stopped and may still be running " )
2015-04-20 11:50:33 +02:00
Merge #14519: tests: add utility to easily profile node performance with perf
13782b8ba8 docs: add perf section to developer docs (James O'Beirne)
58180b5fd4 tests: add utility to easily profile node performance with perf (James O'Beirne)
Pull request description:
Adds a context manager to easily (and selectively) profile node performance during functional test execution using `perf`.
While writing some tests, I encountered some odd bitcoind slowness. I wrote up a utility (`TestNode.profile_with_perf`) that generates performance diagnostics for a node by running `perf` during the execution of a particular region of test code.
`perf` usage is detailed in the excellent (and sadly unmerged) https://github.com/bitcoin/bitcoin/pull/12649; all due props to @eklitzke.
### Example
```python
with node.profile_with_perf("large-msgs"):
for i in range(200):
node.p2p.send_message(some_large_msg)
node.p2p.sync_with_ping()
```
This generates a perf data file in the test node's datadir (`/tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data`).
Running `perf report` generates nice output about where the node spent most of its time while running that part of the test:
```bash
$ perf report -i /tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data --stdio \
| c++filt \
| less
# To display the perf.data header info, please use --header/--header-only options.
#
#
# Total Lost Samples: 0
#
# Samples: 135 of event 'cycles:pp'
# Event count (approx.): 1458205679493582
#
# Children Self Command Shared Object Symbol
# ........ ........ ............... ................... ........................................................................................................................................................................................................................................................................
#
70.14% 0.00% bitcoin-net bitcoind [.] CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
|
---CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
70.14% 0.00% bitcoin-net bitcoind [.] CNetMessage::readData(char const*, unsigned int)
|
---CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
35.52% 0.00% bitcoin-net bitcoind [.] std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
|
---std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
...
```
Tree-SHA512: 9ac4ceaa88818d5eca00994e8e3c8ad42ae019550d6583972a0a4f7b0c4f61032e3d0c476b4ae58756bc5eb8f8015a19a7fc26c095bd588f31d49a37ed0c6b3e
2019-02-05 23:40:11 +01:00
should_clean_up = (
not self . options . nocleanup and
not self . options . noshutdown and
2019-11-04 20:52:51 +01:00
self . success != TestStatus . FAILED and
Merge #14519: tests: add utility to easily profile node performance with perf
13782b8ba8 docs: add perf section to developer docs (James O'Beirne)
58180b5fd4 tests: add utility to easily profile node performance with perf (James O'Beirne)
Pull request description:
Adds a context manager to easily (and selectively) profile node performance during functional test execution using `perf`.
While writing some tests, I encountered some odd bitcoind slowness. I wrote up a utility (`TestNode.profile_with_perf`) that generates performance diagnostics for a node by running `perf` during the execution of a particular region of test code.
`perf` usage is detailed in the excellent (and sadly unmerged) https://github.com/bitcoin/bitcoin/pull/12649; all due props to @eklitzke.
### Example
```python
with node.profile_with_perf("large-msgs"):
for i in range(200):
node.p2p.send_message(some_large_msg)
node.p2p.sync_with_ping()
```
This generates a perf data file in the test node's datadir (`/tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data`).
Running `perf report` generates nice output about where the node spent most of its time while running that part of the test:
```bash
$ perf report -i /tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data --stdio \
| c++filt \
| less
# To display the perf.data header info, please use --header/--header-only options.
#
#
# Total Lost Samples: 0
#
# Samples: 135 of event 'cycles:pp'
# Event count (approx.): 1458205679493582
#
# Children Self Command Shared Object Symbol
# ........ ........ ............... ................... ........................................................................................................................................................................................................................................................................
#
70.14% 0.00% bitcoin-net bitcoind [.] CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
|
---CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
70.14% 0.00% bitcoin-net bitcoind [.] CNetMessage::readData(char const*, unsigned int)
|
---CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
35.52% 0.00% bitcoin-net bitcoind [.] std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
|
---std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
...
```
Tree-SHA512: 9ac4ceaa88818d5eca00994e8e3c8ad42ae019550d6583972a0a4f7b0c4f61032e3d0c476b4ae58756bc5eb8f8015a19a7fc26c095bd588f31d49a37ed0c6b3e
2019-02-05 23:40:11 +01:00
not self . options . perf
)
if should_clean_up :
2018-02-12 11:30:17 +01:00
self . log . info ( " Cleaning up {} on exit " . format ( self . options . tmpdir ) )
cleanup_tree_on_exit = True
Merge #14519: tests: add utility to easily profile node performance with perf
13782b8ba8 docs: add perf section to developer docs (James O'Beirne)
58180b5fd4 tests: add utility to easily profile node performance with perf (James O'Beirne)
Pull request description:
Adds a context manager to easily (and selectively) profile node performance during functional test execution using `perf`.
While writing some tests, I encountered some odd bitcoind slowness. I wrote up a utility (`TestNode.profile_with_perf`) that generates performance diagnostics for a node by running `perf` during the execution of a particular region of test code.
`perf` usage is detailed in the excellent (and sadly unmerged) https://github.com/bitcoin/bitcoin/pull/12649; all due props to @eklitzke.
### Example
```python
with node.profile_with_perf("large-msgs"):
for i in range(200):
node.p2p.send_message(some_large_msg)
node.p2p.sync_with_ping()
```
This generates a perf data file in the test node's datadir (`/tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data`).
Running `perf report` generates nice output about where the node spent most of its time while running that part of the test:
```bash
$ perf report -i /tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data --stdio \
| c++filt \
| less
# To display the perf.data header info, please use --header/--header-only options.
#
#
# Total Lost Samples: 0
#
# Samples: 135 of event 'cycles:pp'
# Event count (approx.): 1458205679493582
#
# Children Self Command Shared Object Symbol
# ........ ........ ............... ................... ........................................................................................................................................................................................................................................................................
#
70.14% 0.00% bitcoin-net bitcoind [.] CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
|
---CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
70.14% 0.00% bitcoin-net bitcoind [.] CNetMessage::readData(char const*, unsigned int)
|
---CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
35.52% 0.00% bitcoin-net bitcoind [.] std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
|
---std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
...
```
Tree-SHA512: 9ac4ceaa88818d5eca00994e8e3c8ad42ae019550d6583972a0a4f7b0c4f61032e3d0c476b4ae58756bc5eb8f8015a19a7fc26c095bd588f31d49a37ed0c6b3e
2019-02-05 23:40:11 +01:00
elif self . options . perf :
self . log . warning ( " Not cleaning up dir {} due to perf data " . format ( self . options . tmpdir ) )
cleanup_tree_on_exit = False
2016-05-25 11:52:25 +02:00
else :
Merge #14519: tests: add utility to easily profile node performance with perf
13782b8ba8 docs: add perf section to developer docs (James O'Beirne)
58180b5fd4 tests: add utility to easily profile node performance with perf (James O'Beirne)
Pull request description:
Adds a context manager to easily (and selectively) profile node performance during functional test execution using `perf`.
While writing some tests, I encountered some odd bitcoind slowness. I wrote up a utility (`TestNode.profile_with_perf`) that generates performance diagnostics for a node by running `perf` during the execution of a particular region of test code.
`perf` usage is detailed in the excellent (and sadly unmerged) https://github.com/bitcoin/bitcoin/pull/12649; all due props to @eklitzke.
### Example
```python
with node.profile_with_perf("large-msgs"):
for i in range(200):
node.p2p.send_message(some_large_msg)
node.p2p.sync_with_ping()
```
This generates a perf data file in the test node's datadir (`/tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data`).
Running `perf report` generates nice output about where the node spent most of its time while running that part of the test:
```bash
$ perf report -i /tmp/testtxmpod0y/node0/node-0-TestName-large-msgs.perf.data --stdio \
| c++filt \
| less
# To display the perf.data header info, please use --header/--header-only options.
#
#
# Total Lost Samples: 0
#
# Samples: 135 of event 'cycles:pp'
# Event count (approx.): 1458205679493582
#
# Children Self Command Shared Object Symbol
# ........ ........ ............... ................... ........................................................................................................................................................................................................................................................................
#
70.14% 0.00% bitcoin-net bitcoind [.] CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
|
---CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
70.14% 0.00% bitcoin-net bitcoind [.] CNetMessage::readData(char const*, unsigned int)
|
---CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
35.52% 0.00% bitcoin-net bitcoind [.] std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
|
---std::vector<char, zero_after_free_allocator<char> >::_M_fill_insert(__gnu_cxx::__normal_iterator<char*, std::vector<char, zero_after_free_allocator<char> > >, unsigned long, char const&)
CNetMessage::readData(char const*, unsigned int)
CNode::ReceiveMsgBytes(char const*, unsigned int, bool&)
...
```
Tree-SHA512: 9ac4ceaa88818d5eca00994e8e3c8ad42ae019550d6583972a0a4f7b0c4f61032e3d0c476b4ae58756bc5eb8f8015a19a7fc26c095bd588f31d49a37ed0c6b3e
2019-02-05 23:40:11 +01:00
self . log . warning ( " Not cleaning up dir {} " . format ( self . options . tmpdir ) )
2018-02-12 11:30:17 +01:00
cleanup_tree_on_exit = False
2017-06-02 11:32:55 +02:00
2019-11-04 20:52:51 +01:00
if self . success == TestStatus . PASSED :
2017-03-09 21:16:20 +01:00
self . log . info ( " Tests successful " )
2017-11-29 19:21:51 +01:00
exit_code = TEST_EXIT_PASSED
2019-11-04 20:52:51 +01:00
elif self . success == TestStatus . SKIPPED :
2017-06-02 11:32:55 +02:00
self . log . info ( " Test skipped " )
2017-11-29 19:21:51 +01:00
exit_code = TEST_EXIT_SKIPPED
2014-07-08 18:07:23 +02:00
else :
2017-03-09 21:16:20 +01:00
self . log . error ( " Test failed. Test logging available at %s /test_framework.log " , self . options . tmpdir )
2020-05-30 12:49:24 +02:00
self . log . error ( " " )
2017-11-29 19:21:51 +01:00
self . log . error ( " Hint: Call {} ' {} ' to consolidate all logs " . format ( os . path . normpath ( os . path . dirname ( os . path . realpath ( __file__ ) ) + " /../combine_logs.py " ) , self . options . tmpdir ) )
2020-05-30 12:49:24 +02:00
self . log . error ( " " )
self . log . error ( " If this failure happened unexpectedly or intermittently, please file a bug and provide a link or upload of the combined log. " )
self . log . error ( self . config [ ' environment ' ] [ ' PACKAGE_BUGREPORT ' ] )
self . log . error ( " " )
2017-11-29 19:21:51 +01:00
exit_code = TEST_EXIT_FAILED
2019-11-04 20:52:51 +01:00
# Logging.shutdown will not remove stream- and filehandlers, so we must
# do it explicitly. Handlers are removed so the next test run can apply
# different log handler settings.
# See: https://docs.python.org/3/library/logging.html#logging.shutdown
for h in list ( self . log . handlers ) :
h . flush ( )
h . close ( )
self . log . removeHandler ( h )
rpc_logger = logging . getLogger ( " BitcoinRPC " )
for h in list ( rpc_logger . handlers ) :
h . flush ( )
rpc_logger . removeHandler ( h )
2018-02-12 11:30:17 +01:00
if cleanup_tree_on_exit :
shutil . rmtree ( self . options . tmpdir )
2019-11-04 20:52:51 +01:00
self . nodes . clear ( )
return exit_code
2015-04-28 18:39:47 +02:00
2017-09-01 18:47:13 +02:00
# Methods to override in subclass test scripts.
def set_test_params ( self ) :
2020-12-27 10:42:48 +01:00
""" Tests must override this method to change default values for number of nodes, topology, etc """
2017-09-01 18:47:13 +02:00
raise NotImplementedError
2017-05-07 15:13:29 +02:00
2017-09-01 18:47:13 +02:00
def add_options ( self , parser ) :
""" Override this method to add command-line options to the test """
pass
2017-06-29 17:37:19 +02:00
2018-09-13 12:33:15 +02:00
def skip_test_if_missing_module ( self ) :
""" Override this method to skip a test if a module is not compiled """
pass
2017-09-01 18:47:13 +02:00
def setup_chain ( self ) :
""" Override this method to customize blockchain setup """
self . log . info ( " Initializing test directory " + self . options . tmpdir )
if self . setup_clean_chain :
self . _initialize_chain_clean ( )
else :
self . _initialize_chain ( )
2024-08-30 09:47:15 +02:00
if not self . disable_mocktime :
self . _initialize_mocktime ( is_genesis = self . setup_clean_chain )
2017-06-29 17:37:19 +02:00
2017-09-01 18:47:13 +02:00
def setup_network ( self ) :
""" Override this method to customize test network topology """
self . setup_nodes ( )
# Connect the nodes as a "chain". This allows us
# to split the network between nodes 1 and 2 to get
# two halves that can work on competing chains.
2019-09-18 20:41:14 +02:00
#
# Topology looks like this:
# node0 <-- node1 <-- node2 <-- node3
#
# If all nodes are in IBD (clean chain from genesis), node0 is assumed to be the source of blocks (miner). To
# ensure block propagation, all nodes will establish outgoing connections toward node0.
# See fPreferredDownload in net_processing.
#
# If further outbound connections are needed, they can be added at the beginning of the test with e.g.
2020-06-07 15:54:56 +02:00
# self.connect_nodes(1, 2)
2017-09-01 18:47:13 +02:00
for i in range ( self . num_nodes - 1 ) :
2020-06-07 15:54:56 +02:00
self . connect_nodes ( i + 1 , i )
2017-09-01 18:47:13 +02:00
self . sync_all ( )
def setup_nodes ( self ) :
""" Override this method to customize test node setup """
2024-01-27 18:29:53 +01:00
""" If this method is updated - backport changes to DashTestFramework.setup_nodes """
Merge #16528: Native Descriptor Wallets using DescriptorScriptPubKeyMan
223588b1bbc63dc57098bbd0baa48635e0cc0b82 Add a --descriptors option to various tests (Andrew Chow)
869f7ab30aeb4d7fbd563c535b55467a8a0430cf tests: Add RPCOverloadWrapper which overloads some disabled RPCs (Andrew Chow)
cf060628590fab87d73f278e744d70ef2d5d81db Correctly check for default wallet (Andrew Chow)
886e0d75f5fea2421190aa4812777d89f68962cc Implement CWallet::IsSpentKey for non-LegacySPKMans (Andrew Chow)
3c19fdd2a2fd5394fcfa75b2ba84ab2277cbdabf Return error when no ScriptPubKeyMan is available for specified type (Andrew Chow)
388ba94231f2f10a0be751c562cdd4650510a90a Change wallet_encryption.py to use signmessage instead of dumpprivkey (Andrew Chow)
1346e14831489f9c8f53a08f9dfed61d55d53c6f Functional tests for descriptor wallets (Andrew Chow)
f193ea889ddb53d9a5c47647966681d525e38368 add importdescriptors RPC and tests for native descriptor wallets (Hugo Nguyen)
ce24a944940019185efebcc5d85eac458ed26016 Add IsLegacy to CWallet so that the GUI knows whether to show watchonly (Andrew Chow)
1cb42b22b11c27e64462afc25a94b2fc50bfa113 Generate new descriptors when encrypting (Andrew Chow)
82ae02b1656819f4bd5023b8955447e1d4ea8692 Be able to create new wallets with DescriptorScriptPubKeyMans as backing (Andrew Chow)
b713baa75a62335ab9c0eed9ef76a95bfec30668 Implement GetMetadata in DescriptorScriptPubKeyMan (Andrew Chow)
8b9603bd0b443e2f7984eb72bf2e21cf02af0bcb Change GetMetadata to use unique_ptr<CKeyMetadata> (Andrew Chow)
72a9540df96ffdb94f039b9c14eaacdc7d961196 Implement FillPSBT in DescriptorScriptPubKeyMan (Andrew Chow)
84b4978c02102171775c77a45f6ec198930f0a88 Implement SignMessage for descriptor wallets (Andrew Chow)
bde7c9fa38775a81d53ac0484fa9c98076a0c7d1 Implement SignTransaction in DescriptorScriptPubKeyMan (Andrew Chow)
d50c8ddd4190f20bf0debd410348b73408ec3143 Implement GetSolvingProvider for DescriptorScriptPubKeyMan (Andrew Chow)
f1ca5feb4ad668a3e1ae543d0addd5f483f1a88f Implement GetKeypoolOldestTime and only display it if greater than 0 (Andrew Chow)
586b57a9a6b4b12a78f792785b63a5a1743bce0c Implement ReturnDestination in DescriptorScriptPubKeyMan (Andrew Chow)
f866957979c23cefd41efa9dae9e53b9177818dc Implement GetReservedDestination in DescriptorScriptPubKeyMan (Andrew Chow)
a775f7c7fd0b9094fcbeee6ba92206d5bbb19164 Implement Unlock and Encrypt in DescriptorScriptPubKeyMan (Andrew Chow)
bfdd0734869a22217c15858d7a76d0dacc2ebc86 Implement GetNewDestination for DescriptorScriptPubKeyMan (Andrew Chow)
58c7651821b0eeff0a99dc61d78d2e9e07986580 Implement TopUp in DescriptorScriptPubKeyMan (Andrew Chow)
e014886a342508f7c8d80323eee9a5f314eaf94c Implement SetupGeneration for DescriptorScriptPubKeyMan (Andrew Chow)
46dfb99768e7d03a3cf552812d5b41ceaebc06be Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file (Andrew Chow)
4cb9b69be031e1dc65d8964794781b347fd948f5 Implement several simple functions in DescriptorScriptPubKeyMan (Andrew Chow)
d1ec3e4f19487b4b100f80ad02eac063c571777d Add IsSingleType to Descriptors (Andrew Chow)
953feb3d2724f5398dd48990c4957a19313d2c8c Implement loading of keys for DescriptorScriptPubKeyMan (Andrew Chow)
2363e9fcaa41b68bf11153f591b95f2d41ff9a1a Load the descriptor cache from the wallet file (Andrew Chow)
46c46aebb7943e1e2e96755e94dc6c197920bf75 Implement GetID for DescriptorScriptPubKeyMan (Andrew Chow)
ec2f9e1178c8e38c0a5ca063fe81adac8f916348 Implement IsHDEnabled in DescriptorScriptPubKeyMan (Andrew Chow)
741122d4c1a62ced3e96d16d67f4eeb3a6522d99 Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan (Andrew Chow)
2db7ca765c8fb2c71dd6f7c4f29ad70e68ff1720 Implement IsMine for DescriptorScriptPubKeyMan (Andrew Chow)
db7177af8c159abbcc209f2caafcd45d54c181c5 Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet (Andrew Chow)
78f8a92910d34247fa5d04368338c598d9908267 Implement SetType in DescriptorScriptPubKeyMan (Andrew Chow)
834de0300cde57ca3f662fb7aa5b1bdaed68bc8f Store WalletDescriptor in DescriptorScriptPubKeyMan (Andrew Chow)
d8132669e10c1db9ae0c2ea0d3f822d7d2f01345 Add a lock cs_desc_man for DescriptorScriptPubKeyMan (Andrew Chow)
3194a7f88ac1a32997b390b4f188c4b6a4af04a5 Introduce WalletDescriptor class (Andrew Chow)
6b13cd3fa854dfaeb9e269bff3d67cacc0e5b5dc Create LegacyScriptPubKeyMan when not a descriptor wallet (Andrew Chow)
aeac157c9dc141546b45e06ba9c2e641ad86083f Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet (Andrew Chow)
96accc73f067c7c95946e9932645dd821ef67f63 Add WALLET_FLAG_DESCRIPTORS (Andrew Chow)
6b8119af53ee2fdb4c4b5b24b4e650c0dc3bd27c Introduce DescriptorScriptPubKeyMan as a dummy class (Andrew Chow)
06620302c713cae65ee8e4ff9302e4c88e2a1285 Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it (Andrew Chow)
Pull request description:
Introducing the wallet of the glorious future (again): native descriptor wallets. With native descriptor wallets, addresses are generated from descriptors. Instead of generating keys and deriving addresses from keys, addresses come from the scriptPubKeys produced by a descriptor. Native descriptor wallets will be optional for now and can only be created by using `createwallet`.
Descriptor wallets will store descriptors, master keys from the descriptor, and descriptor cache entries. Keys are derived from descriptors on the fly. In order to allow choosing different address types, 6 descriptors are needed for normal use. There is a pair of primary and change descriptors for each of the 3 address types. With the default keypool size of 1000, each descriptor has 1000 scriptPubKeys and descriptor cache entries pregenerated. This has a side effect of making wallets large since 6000 pubkeys are written to the wallet by default, instead of the current 2000. scriptPubKeys are kept only in memory and are generated every time a descriptor is loaded. By default, we use the standard BIP 44, 49, 84 derivation paths with an external and internal derivation chain for each.
Descriptors can also be imported with a new `importdescriptors` RPC.
Native descriptor wallets use the `ScriptPubKeyMan` interface introduced in #16341 to add a `DescriptorScriptPubKeyMan`. This defines a different IsMine which uses the simpler model of "does this scriptPubKey exist in this wallet". Furthermore, `DescriptorScriptPubKeyMan` does not have watchonly, so with native descriptor wallets, it is not possible to have a wallet with both watchonly and non-watchonly things. Rather a wallet with `disable_private_keys` needs to be used for watchonly things.
A `--descriptor` option was added to some tests (`wallet_basic.py`, `wallet_encryption.py`, `wallet_keypool.py`, `wallet_keypool_topup.py`, and `wallet_labels.py`) to allow for these tests to use descriptor wallets. Additionally, several RPCs are disabled for descriptor wallets (`importprivkey`, `importpubkey`, `importaddress`, `importmulti`, `addmultisigaddress`, `dumpprivkey`, `dumpwallet`, `importwallet`, and `sethdseed`).
ACKs for top commit:
Sjors:
utACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82 (rebased, nits addressed)
jonatack:
Code review re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82.
fjahr:
re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
instagibbs:
light re-ACK 223588b
meshcollider:
Code review ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
Tree-SHA512: 59bc52aeddbb769ed5f420d5d240d8137847ac821b588eb616b34461253510c1717d6a70bab8765631738747336ae06f45ba39603ccd17f483843e5ed9a90986
Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it
Introduce DescriptorScriptPubKeyMan as a dummy class
Add WALLET_FLAG_DESCRIPTORS
Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet
Create LegacyScriptPubKeyMan when not a descriptor wallet
Introduce WalletDescriptor class
WalletDescriptor is a Descriptor with other wallet metadata
Add a lock cs_desc_man for DescriptorScriptPubKeyMan
Store WalletDescriptor in DescriptorScriptPubKeyMan
Implement SetType in DescriptorScriptPubKeyMan
Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet
Implement IsMine for DescriptorScriptPubKeyMan
Adds a set of scriptPubKeys that DescriptorScriptPubKeyMan tracks.
If the given script is in that set, it is considered ISMINE_SPENDABLE
Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan
Implement IsHDEnabled in DescriptorScriptPubKeyMan
Implement GetID for DescriptorScriptPubKeyMan
Load the descriptor cache from the wallet file
Implement loading of keys for DescriptorScriptPubKeyMan
Add IsSingleType to Descriptors
IsSingleType will return whether the descriptor will give one or multiple scriptPubKeys
Implement several simple functions in DescriptorScriptPubKeyMan
Implements a bunch of one liners: UpgradeKeyMetadata, IsFirstRun, HavePrivateKeys,
KeypoolCountExternalKeys, GetKeypoolSize, GetTimeFirstKey, CanGetAddresses,
RewriteDB
Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file
Implement SetupGeneration for DescriptorScriptPubKeyMan
Implement TopUp in DescriptorScriptPubKeyMan
Implement GetNewDestination for DescriptorScriptPubKeyMan
Implement Unlock and Encrypt in DescriptorScriptPubKeyMan
Implement GetReservedDestination in DescriptorScriptPubKeyMan
Implement ReturnDestination in DescriptorScriptPubKeyMan
Implement GetKeypoolOldestTime and only display it if greater than 0
Implement GetSolvingProvider for DescriptorScriptPubKeyMan
Internally, a GetSigningProvider function is introduced which allows for
some private keys to be optionally included. This can be called with a
script as the argument (i.e. a scriptPubKey from our wallet when we are
signing) or with a pubkey. In order to know what index to expand the
private keys for that pubkey, we need to also cache all of the pubkeys
involved when we expand the descriptor. So SetCache and TopUp are
updated to do this too.
Implement SignTransaction in DescriptorScriptPubKeyMan
Implement SignMessage for descriptor wallets
Implement FillPSBT in DescriptorScriptPubKeyMan
FillPSBT will add our own scripts to the PSBT if those inputs are ours.
If an input also lists pubkeys that we happen to know the private keys
for, we will sign those inputs too.
Change GetMetadata to use unique_ptr<CKeyMetadata>
Implement GetMetadata in DescriptorScriptPubKeyMan
Be able to create new wallets with DescriptorScriptPubKeyMans as backing
Generate new descriptors when encrypting
Add IsLegacy to CWallet so that the GUI knows whether to show watchonly
add importdescriptors RPC and tests for native descriptor wallets
Co-authored-by: Andrew Chow <achow101-github@achow101.com>
Functional tests for descriptor wallets
Change wallet_encryption.py to use signmessage instead of dumpprivkey
Return error when no ScriptPubKeyMan is available for specified type
When a CWallet doesn't have a ScriptPubKeyMan for the requested type
in GetNewDestination, give a meaningful error. Also handle this in
Qt which did not do anything with errors.
Implement CWallet::IsSpentKey for non-LegacySPKMans
tests: Add RPCOverloadWrapper which overloads some disabled RPCs
RPCOverloadWrapper overloads some deprecated or disabled RPCs with
an implementation using other RPCs to avoid having a ton of code churn
around replacing those RPCs.
Add a --descriptors option to various tests
Adds a --descriptors option globally to the test framework. This will
make the test create and use descriptor wallets. However some tests may
not work with this.
Some tests are modified to work with --descriptors and run with that
option in test_runer:
* wallet_basic.py
* wallet_encryption.py
* wallet_keypool.py <---- wallet_keypool_hd.py actually
* wallet_keypool_topup.py
* wallet_labels.py
* wallet_avoidreuse.py
2019-07-16 19:34:35 +02:00
extra_args = [ [ ] ] * self . num_nodes
2017-09-01 18:47:13 +02:00
if hasattr ( self , " extra_args " ) :
extra_args = self . extra_args
2021-06-17 19:05:11 +02:00
self . add_nodes ( self . num_nodes , extra_args )
2017-09-01 18:47:13 +02:00
self . start_nodes ( )
2023-02-14 09:48:28 +01:00
if self . requires_wallet :
2022-11-30 20:23:48 +01:00
self . import_deterministic_coinbase_privkeys ( )
2019-02-25 17:44:18 +01:00
if not self . setup_clean_chain :
for n in self . nodes :
assert_equal ( n . getblockchaininfo ( ) [ " blocks " ] , 199 )
# To ensure that all nodes are out of IBD, the most recent block
# must have a timestamp not too old (see IsInitialBlockDownload()).
2024-08-30 09:47:15 +02:00
if not self . disable_mocktime :
self . log . debug ( ' Generate a block with current mocktime ' )
2024-08-30 14:33:15 +02:00
self . bump_mocktime ( 156 * 200 , update_schedulers = False )
2024-09-26 21:17:04 +02:00
block_hash = self . generate ( self . nodes [ 0 ] , 1 , sync_fun = self . no_op ) [ 0 ]
2019-02-25 17:44:18 +01:00
block = self . nodes [ 0 ] . getblock ( blockhash = block_hash , verbosity = 0 )
for n in self . nodes :
n . submitblock ( block )
chain_info = n . getblockchaininfo ( )
assert_equal ( chain_info [ " blocks " ] , 200 )
assert_equal ( chain_info [ " initialblockdownload " ] , False )
2017-09-01 18:47:13 +02:00
2018-09-10 22:58:15 +02:00
def import_deterministic_coinbase_privkeys ( self ) :
2022-11-30 20:24:02 +01:00
for i in range ( len ( self . nodes ) ) :
self . init_wallet ( i )
def init_wallet ( self , i ) :
wallet_name = self . default_wallet_name if self . wallet_names is None else self . wallet_names [ i ] if i < len ( self . wallet_names ) else False
if wallet_name is not False :
n = self . nodes [ i ]
2022-11-30 20:23:48 +01:00
if wallet_name is not None :
Merge #16528: Native Descriptor Wallets using DescriptorScriptPubKeyMan
223588b1bbc63dc57098bbd0baa48635e0cc0b82 Add a --descriptors option to various tests (Andrew Chow)
869f7ab30aeb4d7fbd563c535b55467a8a0430cf tests: Add RPCOverloadWrapper which overloads some disabled RPCs (Andrew Chow)
cf060628590fab87d73f278e744d70ef2d5d81db Correctly check for default wallet (Andrew Chow)
886e0d75f5fea2421190aa4812777d89f68962cc Implement CWallet::IsSpentKey for non-LegacySPKMans (Andrew Chow)
3c19fdd2a2fd5394fcfa75b2ba84ab2277cbdabf Return error when no ScriptPubKeyMan is available for specified type (Andrew Chow)
388ba94231f2f10a0be751c562cdd4650510a90a Change wallet_encryption.py to use signmessage instead of dumpprivkey (Andrew Chow)
1346e14831489f9c8f53a08f9dfed61d55d53c6f Functional tests for descriptor wallets (Andrew Chow)
f193ea889ddb53d9a5c47647966681d525e38368 add importdescriptors RPC and tests for native descriptor wallets (Hugo Nguyen)
ce24a944940019185efebcc5d85eac458ed26016 Add IsLegacy to CWallet so that the GUI knows whether to show watchonly (Andrew Chow)
1cb42b22b11c27e64462afc25a94b2fc50bfa113 Generate new descriptors when encrypting (Andrew Chow)
82ae02b1656819f4bd5023b8955447e1d4ea8692 Be able to create new wallets with DescriptorScriptPubKeyMans as backing (Andrew Chow)
b713baa75a62335ab9c0eed9ef76a95bfec30668 Implement GetMetadata in DescriptorScriptPubKeyMan (Andrew Chow)
8b9603bd0b443e2f7984eb72bf2e21cf02af0bcb Change GetMetadata to use unique_ptr<CKeyMetadata> (Andrew Chow)
72a9540df96ffdb94f039b9c14eaacdc7d961196 Implement FillPSBT in DescriptorScriptPubKeyMan (Andrew Chow)
84b4978c02102171775c77a45f6ec198930f0a88 Implement SignMessage for descriptor wallets (Andrew Chow)
bde7c9fa38775a81d53ac0484fa9c98076a0c7d1 Implement SignTransaction in DescriptorScriptPubKeyMan (Andrew Chow)
d50c8ddd4190f20bf0debd410348b73408ec3143 Implement GetSolvingProvider for DescriptorScriptPubKeyMan (Andrew Chow)
f1ca5feb4ad668a3e1ae543d0addd5f483f1a88f Implement GetKeypoolOldestTime and only display it if greater than 0 (Andrew Chow)
586b57a9a6b4b12a78f792785b63a5a1743bce0c Implement ReturnDestination in DescriptorScriptPubKeyMan (Andrew Chow)
f866957979c23cefd41efa9dae9e53b9177818dc Implement GetReservedDestination in DescriptorScriptPubKeyMan (Andrew Chow)
a775f7c7fd0b9094fcbeee6ba92206d5bbb19164 Implement Unlock and Encrypt in DescriptorScriptPubKeyMan (Andrew Chow)
bfdd0734869a22217c15858d7a76d0dacc2ebc86 Implement GetNewDestination for DescriptorScriptPubKeyMan (Andrew Chow)
58c7651821b0eeff0a99dc61d78d2e9e07986580 Implement TopUp in DescriptorScriptPubKeyMan (Andrew Chow)
e014886a342508f7c8d80323eee9a5f314eaf94c Implement SetupGeneration for DescriptorScriptPubKeyMan (Andrew Chow)
46dfb99768e7d03a3cf552812d5b41ceaebc06be Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file (Andrew Chow)
4cb9b69be031e1dc65d8964794781b347fd948f5 Implement several simple functions in DescriptorScriptPubKeyMan (Andrew Chow)
d1ec3e4f19487b4b100f80ad02eac063c571777d Add IsSingleType to Descriptors (Andrew Chow)
953feb3d2724f5398dd48990c4957a19313d2c8c Implement loading of keys for DescriptorScriptPubKeyMan (Andrew Chow)
2363e9fcaa41b68bf11153f591b95f2d41ff9a1a Load the descriptor cache from the wallet file (Andrew Chow)
46c46aebb7943e1e2e96755e94dc6c197920bf75 Implement GetID for DescriptorScriptPubKeyMan (Andrew Chow)
ec2f9e1178c8e38c0a5ca063fe81adac8f916348 Implement IsHDEnabled in DescriptorScriptPubKeyMan (Andrew Chow)
741122d4c1a62ced3e96d16d67f4eeb3a6522d99 Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan (Andrew Chow)
2db7ca765c8fb2c71dd6f7c4f29ad70e68ff1720 Implement IsMine for DescriptorScriptPubKeyMan (Andrew Chow)
db7177af8c159abbcc209f2caafcd45d54c181c5 Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet (Andrew Chow)
78f8a92910d34247fa5d04368338c598d9908267 Implement SetType in DescriptorScriptPubKeyMan (Andrew Chow)
834de0300cde57ca3f662fb7aa5b1bdaed68bc8f Store WalletDescriptor in DescriptorScriptPubKeyMan (Andrew Chow)
d8132669e10c1db9ae0c2ea0d3f822d7d2f01345 Add a lock cs_desc_man for DescriptorScriptPubKeyMan (Andrew Chow)
3194a7f88ac1a32997b390b4f188c4b6a4af04a5 Introduce WalletDescriptor class (Andrew Chow)
6b13cd3fa854dfaeb9e269bff3d67cacc0e5b5dc Create LegacyScriptPubKeyMan when not a descriptor wallet (Andrew Chow)
aeac157c9dc141546b45e06ba9c2e641ad86083f Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet (Andrew Chow)
96accc73f067c7c95946e9932645dd821ef67f63 Add WALLET_FLAG_DESCRIPTORS (Andrew Chow)
6b8119af53ee2fdb4c4b5b24b4e650c0dc3bd27c Introduce DescriptorScriptPubKeyMan as a dummy class (Andrew Chow)
06620302c713cae65ee8e4ff9302e4c88e2a1285 Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it (Andrew Chow)
Pull request description:
Introducing the wallet of the glorious future (again): native descriptor wallets. With native descriptor wallets, addresses are generated from descriptors. Instead of generating keys and deriving addresses from keys, addresses come from the scriptPubKeys produced by a descriptor. Native descriptor wallets will be optional for now and can only be created by using `createwallet`.
Descriptor wallets will store descriptors, master keys from the descriptor, and descriptor cache entries. Keys are derived from descriptors on the fly. In order to allow choosing different address types, 6 descriptors are needed for normal use. There is a pair of primary and change descriptors for each of the 3 address types. With the default keypool size of 1000, each descriptor has 1000 scriptPubKeys and descriptor cache entries pregenerated. This has a side effect of making wallets large since 6000 pubkeys are written to the wallet by default, instead of the current 2000. scriptPubKeys are kept only in memory and are generated every time a descriptor is loaded. By default, we use the standard BIP 44, 49, 84 derivation paths with an external and internal derivation chain for each.
Descriptors can also be imported with a new `importdescriptors` RPC.
Native descriptor wallets use the `ScriptPubKeyMan` interface introduced in #16341 to add a `DescriptorScriptPubKeyMan`. This defines a different IsMine which uses the simpler model of "does this scriptPubKey exist in this wallet". Furthermore, `DescriptorScriptPubKeyMan` does not have watchonly, so with native descriptor wallets, it is not possible to have a wallet with both watchonly and non-watchonly things. Rather a wallet with `disable_private_keys` needs to be used for watchonly things.
A `--descriptor` option was added to some tests (`wallet_basic.py`, `wallet_encryption.py`, `wallet_keypool.py`, `wallet_keypool_topup.py`, and `wallet_labels.py`) to allow for these tests to use descriptor wallets. Additionally, several RPCs are disabled for descriptor wallets (`importprivkey`, `importpubkey`, `importaddress`, `importmulti`, `addmultisigaddress`, `dumpprivkey`, `dumpwallet`, `importwallet`, and `sethdseed`).
ACKs for top commit:
Sjors:
utACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82 (rebased, nits addressed)
jonatack:
Code review re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82.
fjahr:
re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
instagibbs:
light re-ACK 223588b
meshcollider:
Code review ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
Tree-SHA512: 59bc52aeddbb769ed5f420d5d240d8137847ac821b588eb616b34461253510c1717d6a70bab8765631738747336ae06f45ba39603ccd17f483843e5ed9a90986
Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it
Introduce DescriptorScriptPubKeyMan as a dummy class
Add WALLET_FLAG_DESCRIPTORS
Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet
Create LegacyScriptPubKeyMan when not a descriptor wallet
Introduce WalletDescriptor class
WalletDescriptor is a Descriptor with other wallet metadata
Add a lock cs_desc_man for DescriptorScriptPubKeyMan
Store WalletDescriptor in DescriptorScriptPubKeyMan
Implement SetType in DescriptorScriptPubKeyMan
Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet
Implement IsMine for DescriptorScriptPubKeyMan
Adds a set of scriptPubKeys that DescriptorScriptPubKeyMan tracks.
If the given script is in that set, it is considered ISMINE_SPENDABLE
Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan
Implement IsHDEnabled in DescriptorScriptPubKeyMan
Implement GetID for DescriptorScriptPubKeyMan
Load the descriptor cache from the wallet file
Implement loading of keys for DescriptorScriptPubKeyMan
Add IsSingleType to Descriptors
IsSingleType will return whether the descriptor will give one or multiple scriptPubKeys
Implement several simple functions in DescriptorScriptPubKeyMan
Implements a bunch of one liners: UpgradeKeyMetadata, IsFirstRun, HavePrivateKeys,
KeypoolCountExternalKeys, GetKeypoolSize, GetTimeFirstKey, CanGetAddresses,
RewriteDB
Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file
Implement SetupGeneration for DescriptorScriptPubKeyMan
Implement TopUp in DescriptorScriptPubKeyMan
Implement GetNewDestination for DescriptorScriptPubKeyMan
Implement Unlock and Encrypt in DescriptorScriptPubKeyMan
Implement GetReservedDestination in DescriptorScriptPubKeyMan
Implement ReturnDestination in DescriptorScriptPubKeyMan
Implement GetKeypoolOldestTime and only display it if greater than 0
Implement GetSolvingProvider for DescriptorScriptPubKeyMan
Internally, a GetSigningProvider function is introduced which allows for
some private keys to be optionally included. This can be called with a
script as the argument (i.e. a scriptPubKey from our wallet when we are
signing) or with a pubkey. In order to know what index to expand the
private keys for that pubkey, we need to also cache all of the pubkeys
involved when we expand the descriptor. So SetCache and TopUp are
updated to do this too.
Implement SignTransaction in DescriptorScriptPubKeyMan
Implement SignMessage for descriptor wallets
Implement FillPSBT in DescriptorScriptPubKeyMan
FillPSBT will add our own scripts to the PSBT if those inputs are ours.
If an input also lists pubkeys that we happen to know the private keys
for, we will sign those inputs too.
Change GetMetadata to use unique_ptr<CKeyMetadata>
Implement GetMetadata in DescriptorScriptPubKeyMan
Be able to create new wallets with DescriptorScriptPubKeyMans as backing
Generate new descriptors when encrypting
Add IsLegacy to CWallet so that the GUI knows whether to show watchonly
add importdescriptors RPC and tests for native descriptor wallets
Co-authored-by: Andrew Chow <achow101-github@achow101.com>
Functional tests for descriptor wallets
Change wallet_encryption.py to use signmessage instead of dumpprivkey
Return error when no ScriptPubKeyMan is available for specified type
When a CWallet doesn't have a ScriptPubKeyMan for the requested type
in GetNewDestination, give a meaningful error. Also handle this in
Qt which did not do anything with errors.
Implement CWallet::IsSpentKey for non-LegacySPKMans
tests: Add RPCOverloadWrapper which overloads some disabled RPCs
RPCOverloadWrapper overloads some deprecated or disabled RPCs with
an implementation using other RPCs to avoid having a ton of code churn
around replacing those RPCs.
Add a --descriptors option to various tests
Adds a --descriptors option globally to the test framework. This will
make the test create and use descriptor wallets. However some tests may
not work with this.
Some tests are modified to work with --descriptors and run with that
option in test_runer:
* wallet_basic.py
* wallet_encryption.py
* wallet_keypool.py <---- wallet_keypool_hd.py actually
* wallet_keypool_topup.py
* wallet_labels.py
* wallet_avoidreuse.py
2019-07-16 19:34:35 +02:00
n . createwallet ( wallet_name = wallet_name , descriptors = self . options . descriptors , load_on_startup = True )
2021-11-28 02:50:11 +01:00
n . importprivkey ( privkey = n . get_deterministic_priv_key ( ) . key , label = ' coinbase ' , rescan = True )
2018-09-10 22:58:15 +02:00
2017-09-01 18:47:13 +02:00
def run_test ( self ) :
""" Tests must override this method to define test logic """
raise NotImplementedError
2017-06-29 17:37:19 +02:00
2017-09-01 18:47:13 +02:00
# Public helper methods. These can be accessed by the subclass test scripts.
2017-06-29 17:37:19 +02:00
2020-06-03 16:01:29 +02:00
def add_nodes ( self , num_nodes : int , extra_args = None , * , rpchost = None , binary = None , binary_cli = None , versions = None ) :
2018-12-14 19:02:07 +01:00
""" Instantiate TestNode objects.
Should only be called once after the nodes have been specified in
set_test_params ( ) . """
2020-05-01 20:57:45 +02:00
def get_bin_from_version ( version , bin_name , bin_default ) :
if not version :
return bin_default
return os . path . join (
self . options . previous_releases_path ,
re . sub (
r ' \ .0$ ' if version != 150000 else r ' ^$ ' ,
' ' , # remove trailing .0 for point releases
' v {} . {} . {} . {} ' . format (
( version % 100000000 ) / / 1000000 ,
( version % 1000000 ) / / 10000 ,
( version % 10000 ) / / 100 ,
( version % 100 ) / / 1 ,
) ,
) ,
' bin ' ,
bin_name ,
)
2018-03-07 14:51:58 +01:00
if self . bind_to_localhost_only :
extra_confs = [ [ " bind=127.0.0.1 " ] ] * num_nodes
else :
extra_confs = [ [ ] ] * num_nodes
2017-06-29 17:37:19 +02:00
if extra_args is None :
2017-08-15 23:34:07 +02:00
extra_args = [ [ ] ] * num_nodes
2020-02-12 15:19:50 +01:00
if versions is None :
versions = [ None ] * num_nodes
2017-06-29 17:37:19 +02:00
if binary is None :
2020-05-01 20:57:45 +02:00
binary = [ get_bin_from_version ( v , ' dashd ' , self . options . bitcoind ) for v in versions ]
2020-02-12 15:19:50 +01:00
if binary_cli is None :
2020-05-01 20:57:45 +02:00
binary_cli = [ get_bin_from_version ( v , ' dash-cli ' , self . options . bitcoincli ) for v in versions ]
2018-03-07 14:51:58 +01:00
assert_equal ( len ( extra_confs ) , num_nodes )
2017-06-29 17:37:19 +02:00
assert_equal ( len ( extra_args ) , num_nodes )
2020-02-12 15:19:50 +01:00
assert_equal ( len ( versions ) , num_nodes )
2017-06-29 17:37:19 +02:00
assert_equal ( len ( binary ) , num_nodes )
2020-02-12 15:19:50 +01:00
assert_equal ( len ( binary_cli ) , num_nodes )
2018-12-14 19:02:07 +01:00
old_num_nodes = len ( self . nodes )
2017-09-01 18:47:13 +02:00
for i in range ( num_nodes ) :
2020-06-16 22:28:04 +02:00
test_node_i = TestNode (
2018-12-14 19:02:07 +01:00
old_num_nodes + i ,
get_datadir_path ( self . options . tmpdir , old_num_nodes + i ) ,
self . extra_args_from_options ,
chain = self . chain ,
rpchost = rpchost ,
timewait = self . rpc_timeout ,
2020-05-19 02:00:24 +02:00
timeout_factor = self . options . timeout_factor ,
2018-12-14 19:02:07 +01:00
bitcoind = binary [ i ] ,
2020-02-12 15:19:50 +01:00
bitcoin_cli = binary_cli [ i ] ,
version = versions [ i ] ,
2018-12-14 19:02:07 +01:00
mocktime = self . mocktime ,
coverage_dir = self . options . coveragedir ,
2019-02-19 16:12:07 +01:00
cwd = self . options . tmpdir ,
2018-12-14 19:02:07 +01:00
extra_conf = extra_confs [ i ] ,
extra_args = extra_args [ i ] ,
use_cli = self . options . usecli ,
start_perf = self . options . perf ,
Merge #17633: tests: Add option --valgrind to run the functional tests under Valgrind
5db506ba5943868cc2c845f717508739b7f05714 tests: Add option --valgrind to run nodes under valgrind in the functional tests (practicalswift)
Pull request description:
What is better than fixing bugs? Fixing entire bug classes of course! :)
Add option `--valgrind` to run the functional tests under Valgrind.
Regular functional testing under Valgrind would have caught many of the uninitialized reads we've seen historically.
Let's kill this bug class once and for all: let's never use an uninitialized value ever again. Or at least not one that would be triggered by running the functional tests! :)
My hope is that this addition will make it super-easy to run the functional tests under Valgrind and thus increase the probability of people making use of it :)
Hopefully `test/functional/test_runner.py --valgrind` will become a natural part of the pre-release QA process.
**Usage:**
```
$ test/functional/test_runner.py --help
…
--valgrind run nodes under the valgrind memory error detector:
expect at least a ~10x slowdown, valgrind 3.14 or
later required
```
**Live demo:**
First, let's re-introduce a memory bug by reverting the recent P2P uninitialized read bug fix from PR #17624 ("net: Fix an uninitialized read in ProcessMessage(…, "tx", …) when receiving a transaction we already have").
```
$ git diff
diff --git a/src/consensus/validation.h b/src/consensus/validation.h
index 3401eb64c..940adea33 100644
--- a/src/consensus/validation.h
+++ b/src/consensus/validation.h
@@ -114,7 +114,7 @@ inline ValidationState::~ValidationState() {};
class TxValidationState : public ValidationState {
private:
- TxValidationResult m_result = TxValidationResult::TX_RESULT_UNSET;
+ TxValidationResult m_result;
public:
bool Invalid(TxValidationResult result,
const std::string &reject_reason="",
```
Second, let's test as normal without Valgrind:
```
$ test/functional/p2p_segwit.py -l INFO
2019-11-28T09:30:42.810000Z TestFramework (INFO): Initializing test directory /tmp/bitcoin_func_test__fc8q3qo
…
2019-11-28T09:31:57.187000Z TestFramework (INFO): Subtest: test_non_standard_witness_blinding (Segwit active = True)
…
2019-11-28T09:32:08.265000Z TestFramework (INFO): Tests successful
```
Third, let's test with `--valgrind` and see if the test fail (as we expect) when the unitialized value is used:
```
$ test/functional/p2p_segwit.py -l INFO --valgrind
2019-11-28T09:32:33.018000Z TestFramework (INFO): Initializing test directory /tmp/bitcoin_func_test_gtjecx2l
…
2019-11-28T09:40:36.702000Z TestFramework (INFO): Subtest: test_non_standard_witness_blinding (Segwit active = True)
2019-11-28T09:40:37.813000Z TestFramework (ERROR): Assertion failed
ConnectionRefusedError: [Errno 111] Connection refused
```
ACKs for top commit:
MarcoFalke:
ACK 5db506ba5943868cc2c845f717508739b7f05714
jonatack:
ACK 5db506ba5943868cc2c845f717508739b7f05714
Tree-SHA512: 2eaecacf4da166febad88b2a8ee6d7ac2bcd38d4c1892ca39516b6343e8f8c8814edf5eaf14c90f11a069a0389d24f0713076112ac284de987e72fc5f6cc3795
2019-12-10 19:30:05 +01:00
use_valgrind = self . options . valgrind ,
Merge #16528: Native Descriptor Wallets using DescriptorScriptPubKeyMan
223588b1bbc63dc57098bbd0baa48635e0cc0b82 Add a --descriptors option to various tests (Andrew Chow)
869f7ab30aeb4d7fbd563c535b55467a8a0430cf tests: Add RPCOverloadWrapper which overloads some disabled RPCs (Andrew Chow)
cf060628590fab87d73f278e744d70ef2d5d81db Correctly check for default wallet (Andrew Chow)
886e0d75f5fea2421190aa4812777d89f68962cc Implement CWallet::IsSpentKey for non-LegacySPKMans (Andrew Chow)
3c19fdd2a2fd5394fcfa75b2ba84ab2277cbdabf Return error when no ScriptPubKeyMan is available for specified type (Andrew Chow)
388ba94231f2f10a0be751c562cdd4650510a90a Change wallet_encryption.py to use signmessage instead of dumpprivkey (Andrew Chow)
1346e14831489f9c8f53a08f9dfed61d55d53c6f Functional tests for descriptor wallets (Andrew Chow)
f193ea889ddb53d9a5c47647966681d525e38368 add importdescriptors RPC and tests for native descriptor wallets (Hugo Nguyen)
ce24a944940019185efebcc5d85eac458ed26016 Add IsLegacy to CWallet so that the GUI knows whether to show watchonly (Andrew Chow)
1cb42b22b11c27e64462afc25a94b2fc50bfa113 Generate new descriptors when encrypting (Andrew Chow)
82ae02b1656819f4bd5023b8955447e1d4ea8692 Be able to create new wallets with DescriptorScriptPubKeyMans as backing (Andrew Chow)
b713baa75a62335ab9c0eed9ef76a95bfec30668 Implement GetMetadata in DescriptorScriptPubKeyMan (Andrew Chow)
8b9603bd0b443e2f7984eb72bf2e21cf02af0bcb Change GetMetadata to use unique_ptr<CKeyMetadata> (Andrew Chow)
72a9540df96ffdb94f039b9c14eaacdc7d961196 Implement FillPSBT in DescriptorScriptPubKeyMan (Andrew Chow)
84b4978c02102171775c77a45f6ec198930f0a88 Implement SignMessage for descriptor wallets (Andrew Chow)
bde7c9fa38775a81d53ac0484fa9c98076a0c7d1 Implement SignTransaction in DescriptorScriptPubKeyMan (Andrew Chow)
d50c8ddd4190f20bf0debd410348b73408ec3143 Implement GetSolvingProvider for DescriptorScriptPubKeyMan (Andrew Chow)
f1ca5feb4ad668a3e1ae543d0addd5f483f1a88f Implement GetKeypoolOldestTime and only display it if greater than 0 (Andrew Chow)
586b57a9a6b4b12a78f792785b63a5a1743bce0c Implement ReturnDestination in DescriptorScriptPubKeyMan (Andrew Chow)
f866957979c23cefd41efa9dae9e53b9177818dc Implement GetReservedDestination in DescriptorScriptPubKeyMan (Andrew Chow)
a775f7c7fd0b9094fcbeee6ba92206d5bbb19164 Implement Unlock and Encrypt in DescriptorScriptPubKeyMan (Andrew Chow)
bfdd0734869a22217c15858d7a76d0dacc2ebc86 Implement GetNewDestination for DescriptorScriptPubKeyMan (Andrew Chow)
58c7651821b0eeff0a99dc61d78d2e9e07986580 Implement TopUp in DescriptorScriptPubKeyMan (Andrew Chow)
e014886a342508f7c8d80323eee9a5f314eaf94c Implement SetupGeneration for DescriptorScriptPubKeyMan (Andrew Chow)
46dfb99768e7d03a3cf552812d5b41ceaebc06be Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file (Andrew Chow)
4cb9b69be031e1dc65d8964794781b347fd948f5 Implement several simple functions in DescriptorScriptPubKeyMan (Andrew Chow)
d1ec3e4f19487b4b100f80ad02eac063c571777d Add IsSingleType to Descriptors (Andrew Chow)
953feb3d2724f5398dd48990c4957a19313d2c8c Implement loading of keys for DescriptorScriptPubKeyMan (Andrew Chow)
2363e9fcaa41b68bf11153f591b95f2d41ff9a1a Load the descriptor cache from the wallet file (Andrew Chow)
46c46aebb7943e1e2e96755e94dc6c197920bf75 Implement GetID for DescriptorScriptPubKeyMan (Andrew Chow)
ec2f9e1178c8e38c0a5ca063fe81adac8f916348 Implement IsHDEnabled in DescriptorScriptPubKeyMan (Andrew Chow)
741122d4c1a62ced3e96d16d67f4eeb3a6522d99 Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan (Andrew Chow)
2db7ca765c8fb2c71dd6f7c4f29ad70e68ff1720 Implement IsMine for DescriptorScriptPubKeyMan (Andrew Chow)
db7177af8c159abbcc209f2caafcd45d54c181c5 Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet (Andrew Chow)
78f8a92910d34247fa5d04368338c598d9908267 Implement SetType in DescriptorScriptPubKeyMan (Andrew Chow)
834de0300cde57ca3f662fb7aa5b1bdaed68bc8f Store WalletDescriptor in DescriptorScriptPubKeyMan (Andrew Chow)
d8132669e10c1db9ae0c2ea0d3f822d7d2f01345 Add a lock cs_desc_man for DescriptorScriptPubKeyMan (Andrew Chow)
3194a7f88ac1a32997b390b4f188c4b6a4af04a5 Introduce WalletDescriptor class (Andrew Chow)
6b13cd3fa854dfaeb9e269bff3d67cacc0e5b5dc Create LegacyScriptPubKeyMan when not a descriptor wallet (Andrew Chow)
aeac157c9dc141546b45e06ba9c2e641ad86083f Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet (Andrew Chow)
96accc73f067c7c95946e9932645dd821ef67f63 Add WALLET_FLAG_DESCRIPTORS (Andrew Chow)
6b8119af53ee2fdb4c4b5b24b4e650c0dc3bd27c Introduce DescriptorScriptPubKeyMan as a dummy class (Andrew Chow)
06620302c713cae65ee8e4ff9302e4c88e2a1285 Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it (Andrew Chow)
Pull request description:
Introducing the wallet of the glorious future (again): native descriptor wallets. With native descriptor wallets, addresses are generated from descriptors. Instead of generating keys and deriving addresses from keys, addresses come from the scriptPubKeys produced by a descriptor. Native descriptor wallets will be optional for now and can only be created by using `createwallet`.
Descriptor wallets will store descriptors, master keys from the descriptor, and descriptor cache entries. Keys are derived from descriptors on the fly. In order to allow choosing different address types, 6 descriptors are needed for normal use. There is a pair of primary and change descriptors for each of the 3 address types. With the default keypool size of 1000, each descriptor has 1000 scriptPubKeys and descriptor cache entries pregenerated. This has a side effect of making wallets large since 6000 pubkeys are written to the wallet by default, instead of the current 2000. scriptPubKeys are kept only in memory and are generated every time a descriptor is loaded. By default, we use the standard BIP 44, 49, 84 derivation paths with an external and internal derivation chain for each.
Descriptors can also be imported with a new `importdescriptors` RPC.
Native descriptor wallets use the `ScriptPubKeyMan` interface introduced in #16341 to add a `DescriptorScriptPubKeyMan`. This defines a different IsMine which uses the simpler model of "does this scriptPubKey exist in this wallet". Furthermore, `DescriptorScriptPubKeyMan` does not have watchonly, so with native descriptor wallets, it is not possible to have a wallet with both watchonly and non-watchonly things. Rather a wallet with `disable_private_keys` needs to be used for watchonly things.
A `--descriptor` option was added to some tests (`wallet_basic.py`, `wallet_encryption.py`, `wallet_keypool.py`, `wallet_keypool_topup.py`, and `wallet_labels.py`) to allow for these tests to use descriptor wallets. Additionally, several RPCs are disabled for descriptor wallets (`importprivkey`, `importpubkey`, `importaddress`, `importmulti`, `addmultisigaddress`, `dumpprivkey`, `dumpwallet`, `importwallet`, and `sethdseed`).
ACKs for top commit:
Sjors:
utACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82 (rebased, nits addressed)
jonatack:
Code review re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82.
fjahr:
re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
instagibbs:
light re-ACK 223588b
meshcollider:
Code review ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
Tree-SHA512: 59bc52aeddbb769ed5f420d5d240d8137847ac821b588eb616b34461253510c1717d6a70bab8765631738747336ae06f45ba39603ccd17f483843e5ed9a90986
Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it
Introduce DescriptorScriptPubKeyMan as a dummy class
Add WALLET_FLAG_DESCRIPTORS
Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet
Create LegacyScriptPubKeyMan when not a descriptor wallet
Introduce WalletDescriptor class
WalletDescriptor is a Descriptor with other wallet metadata
Add a lock cs_desc_man for DescriptorScriptPubKeyMan
Store WalletDescriptor in DescriptorScriptPubKeyMan
Implement SetType in DescriptorScriptPubKeyMan
Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet
Implement IsMine for DescriptorScriptPubKeyMan
Adds a set of scriptPubKeys that DescriptorScriptPubKeyMan tracks.
If the given script is in that set, it is considered ISMINE_SPENDABLE
Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan
Implement IsHDEnabled in DescriptorScriptPubKeyMan
Implement GetID for DescriptorScriptPubKeyMan
Load the descriptor cache from the wallet file
Implement loading of keys for DescriptorScriptPubKeyMan
Add IsSingleType to Descriptors
IsSingleType will return whether the descriptor will give one or multiple scriptPubKeys
Implement several simple functions in DescriptorScriptPubKeyMan
Implements a bunch of one liners: UpgradeKeyMetadata, IsFirstRun, HavePrivateKeys,
KeypoolCountExternalKeys, GetKeypoolSize, GetTimeFirstKey, CanGetAddresses,
RewriteDB
Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file
Implement SetupGeneration for DescriptorScriptPubKeyMan
Implement TopUp in DescriptorScriptPubKeyMan
Implement GetNewDestination for DescriptorScriptPubKeyMan
Implement Unlock and Encrypt in DescriptorScriptPubKeyMan
Implement GetReservedDestination in DescriptorScriptPubKeyMan
Implement ReturnDestination in DescriptorScriptPubKeyMan
Implement GetKeypoolOldestTime and only display it if greater than 0
Implement GetSolvingProvider for DescriptorScriptPubKeyMan
Internally, a GetSigningProvider function is introduced which allows for
some private keys to be optionally included. This can be called with a
script as the argument (i.e. a scriptPubKey from our wallet when we are
signing) or with a pubkey. In order to know what index to expand the
private keys for that pubkey, we need to also cache all of the pubkeys
involved when we expand the descriptor. So SetCache and TopUp are
updated to do this too.
Implement SignTransaction in DescriptorScriptPubKeyMan
Implement SignMessage for descriptor wallets
Implement FillPSBT in DescriptorScriptPubKeyMan
FillPSBT will add our own scripts to the PSBT if those inputs are ours.
If an input also lists pubkeys that we happen to know the private keys
for, we will sign those inputs too.
Change GetMetadata to use unique_ptr<CKeyMetadata>
Implement GetMetadata in DescriptorScriptPubKeyMan
Be able to create new wallets with DescriptorScriptPubKeyMans as backing
Generate new descriptors when encrypting
Add IsLegacy to CWallet so that the GUI knows whether to show watchonly
add importdescriptors RPC and tests for native descriptor wallets
Co-authored-by: Andrew Chow <achow101-github@achow101.com>
Functional tests for descriptor wallets
Change wallet_encryption.py to use signmessage instead of dumpprivkey
Return error when no ScriptPubKeyMan is available for specified type
When a CWallet doesn't have a ScriptPubKeyMan for the requested type
in GetNewDestination, give a meaningful error. Also handle this in
Qt which did not do anything with errors.
Implement CWallet::IsSpentKey for non-LegacySPKMans
tests: Add RPCOverloadWrapper which overloads some disabled RPCs
RPCOverloadWrapper overloads some deprecated or disabled RPCs with
an implementation using other RPCs to avoid having a ton of code churn
around replacing those RPCs.
Add a --descriptors option to various tests
Adds a --descriptors option globally to the test framework. This will
make the test create and use descriptor wallets. However some tests may
not work with this.
Some tests are modified to work with --descriptors and run with that
option in test_runer:
* wallet_basic.py
* wallet_encryption.py
* wallet_keypool.py <---- wallet_keypool_hd.py actually
* wallet_keypool_topup.py
* wallet_labels.py
* wallet_avoidreuse.py
2019-07-16 19:34:35 +02:00
descriptors = self . options . descriptors ,
2020-06-16 22:28:04 +02:00
)
self . nodes . append ( test_node_i )
if not test_node_i . version_is_at_least ( 160000 ) :
# adjust conf for pre 16
conf_file = test_node_i . bitcoinconf
with open ( conf_file , ' r ' , encoding = ' utf8 ' ) as conf :
conf_data = conf . read ( )
with open ( conf_file , ' w ' , encoding = ' utf8 ' ) as conf :
conf . write ( conf_data . replace ( ' [regtest] ' , ' ' ) )
2017-09-01 18:47:13 +02:00
2023-02-14 19:48:33 +01:00
def add_dynamically_node ( self , extra_args = None , * , rpchost = None , binary = None ) :
if self . bind_to_localhost_only :
extra_confs = [ [ " bind=127.0.0.1 " ] ]
else :
extra_confs = [ [ ] ]
if extra_args is None :
extra_args = [ [ ] ]
if binary is None :
binary = [ self . options . bitcoind ]
assert_equal ( len ( extra_confs ) , 1 )
assert_equal ( len ( binary ) , 1 )
old_num_nodes = len ( self . nodes )
p0 = old_num_nodes
p1 = get_datadir_path ( self . options . tmpdir , old_num_nodes )
p2 = self . extra_args_from_options
p3 = self . chain
p4 = rpchost
p5 = self . rpc_timeout
p6 = self . options . timeout_factor
p7 = binary [ 0 ]
p8 = self . options . bitcoincli
p9 = self . mocktime
p10 = self . options . coveragedir
p11 = self . options . tmpdir
p12 = extra_confs [ 0 ]
p13 = extra_args
p14 = self . options . usecli
p15 = self . options . perf
p16 = self . options . valgrind
t_node = TestNode ( p0 , p1 , p2 , chain = p3 , rpchost = p4 , timewait = p5 , timeout_factor = p6 , bitcoind = p7 , bitcoin_cli = p8 , mocktime = p9 , coverage_dir = p10 , cwd = p11 , extra_conf = p12 , extra_args = p13 , use_cli = p14 , start_perf = p15 , use_valgrind = p16 )
self . nodes . append ( t_node )
return t_node
2023-05-24 19:38:33 +02:00
def dynamically_initialize_datadir ( self , node_p2p_port , node_rpc_port ) :
source_data_dir = get_datadir_path ( self . options . tmpdir , 0 ) # use node0 as a source
new_data_dir = get_datadir_path ( self . options . tmpdir , len ( self . nodes ) )
# In general, it's a pretty bad idea to copy datadir folder on the fly...
# But we flush all state changes to disk via gettxoutsetinfo call and
# we don't care about wallets, so it works
self . nodes [ 0 ] . gettxoutsetinfo ( )
shutil . copytree ( source_data_dir , new_data_dir )
shutil . rmtree ( os . path . join ( new_data_dir , self . chain , ' wallets ' ) )
shutil . rmtree ( os . path . join ( new_data_dir , self . chain , ' llmq ' ) )
for entry in os . listdir ( os . path . join ( new_data_dir , self . chain ) ) :
if entry not in [ ' chainstate ' , ' blocks ' , ' indexes ' , ' evodb ' ] :
os . remove ( os . path . join ( new_data_dir , self . chain , entry ) )
2023-02-14 19:48:33 +01:00
# Translate chain name to config name
2023-05-24 19:38:33 +02:00
if self . chain == ' testnet3 ' :
2023-02-14 19:48:33 +01:00
chain_name_conf_arg = ' testnet '
chain_name_conf_section = ' test '
chain_name_conf_arg_value = ' 1 '
2023-05-24 19:38:33 +02:00
elif self . chain == ' devnet ' :
2023-02-14 19:48:33 +01:00
chain_name_conf_arg = ' devnet '
chain_name_conf_section = ' devnet '
chain_name_conf_arg_value = ' devnet1 '
else :
2023-05-24 19:38:33 +02:00
chain_name_conf_arg = self . chain
chain_name_conf_section = self . chain
2023-02-14 19:48:33 +01:00
chain_name_conf_arg_value = ' 1 '
2023-05-24 19:38:33 +02:00
with open ( os . path . join ( new_data_dir , " dash.conf " ) , ' w ' , encoding = ' utf8 ' ) as f :
2023-02-14 19:48:33 +01:00
f . write ( " {} = {} \n " . format ( chain_name_conf_arg , chain_name_conf_arg_value ) )
f . write ( " [ {} ] \n " . format ( chain_name_conf_section ) )
f . write ( " port= " + str ( node_p2p_port ) + " \n " )
f . write ( " rpcport= " + str ( node_rpc_port ) + " \n " )
f . write ( " server=1 \n " )
f . write ( " debug=1 \n " )
f . write ( " keypool=1 \n " )
f . write ( " discover=0 \n " )
f . write ( " listenonion=0 \n " )
f . write ( " printtoconsole=0 \n " )
f . write ( " upnp=0 \n " )
f . write ( " natpmp=0 \n " )
f . write ( " shrinkdebugfile=0 \n " )
2023-05-24 19:38:33 +02:00
os . makedirs ( os . path . join ( new_data_dir , ' stderr ' ) , exist_ok = True )
os . makedirs ( os . path . join ( new_data_dir , ' stdout ' ) , exist_ok = True )
2023-02-14 19:48:33 +01:00
2018-01-19 17:44:27 +01:00
def start_node ( self , i , * args , * * kwargs ) :
2019-09-26 15:50:24 +02:00
""" Start a dashd """
2017-09-01 18:47:13 +02:00
node = self . nodes [ i ]
2018-01-19 17:44:27 +01:00
node . start ( * args , * * kwargs )
2017-09-01 18:47:13 +02:00
node . wait_for_rpc_connection ( )
if self . options . coveragedir is not None :
coverage . write_all_rpc_commands ( self . options . coveragedir , node . rpc )
2021-06-17 19:05:11 +02:00
def start_nodes ( self , extra_args = None , * args , * * kwargs ) :
2017-09-01 18:47:13 +02:00
""" Start multiple dashds """
if extra_args is None :
extra_args = [ None ] * self . num_nodes
assert_equal ( len ( extra_args ) , self . num_nodes )
2017-06-29 17:37:19 +02:00
try :
2017-09-01 18:47:13 +02:00
for i , node in enumerate ( self . nodes ) :
2021-06-17 19:05:11 +02:00
node . start ( extra_args [ i ] , * args , * * kwargs )
2017-09-01 18:47:13 +02:00
for node in self . nodes :
2017-08-15 23:34:07 +02:00
node . wait_for_rpc_connection ( )
2017-06-29 17:37:19 +02:00
except :
# If one node failed to start, stop the others
self . stop_nodes ( )
raise
2017-08-15 23:34:07 +02:00
if self . options . coveragedir is not None :
2017-09-01 18:47:13 +02:00
for node in self . nodes :
2017-08-15 23:34:07 +02:00
coverage . write_all_rpc_commands ( self . options . coveragedir , node . rpc )
2021-06-17 19:05:11 +02:00
def stop_node ( self , i , expected_stderr = ' ' , wait = 0 ) :
2019-08-09 01:21:57 +02:00
""" Stop a dashd test node """
2021-06-17 19:05:11 +02:00
self . nodes [ i ] . stop_node ( expected_stderr = expected_stderr , wait = wait )
2019-08-09 01:19:22 +02:00
2021-06-17 19:05:11 +02:00
def stop_nodes ( self , expected_stderr = ' ' , wait = 0 ) :
2019-08-09 01:21:57 +02:00
""" Stop multiple dashd test nodes """
2017-08-15 23:34:07 +02:00
for node in self . nodes :
# Issue RPC to stop nodes
2020-12-21 20:20:18 +01:00
node . stop_node ( expected_stderr = expected_stderr , wait = wait , wait_until_stopped = False )
2017-06-29 17:37:19 +02:00
2017-08-15 23:34:07 +02:00
for node in self . nodes :
# Wait for nodes to stop
2017-09-06 20:02:08 +02:00
node . wait_until_stopped ( )
2017-06-29 17:37:19 +02:00
2021-06-17 19:05:11 +02:00
def restart_node ( self , i , extra_args = None , expected_stderr = ' ' ) :
2017-09-25 13:16:08 +02:00
""" Stop and start a test node """
2021-06-17 19:05:11 +02:00
self . stop_node ( i , expected_stderr )
2017-09-25 13:16:08 +02:00
self . start_node ( i , extra_args )
2017-06-29 17:37:19 +02:00
def wait_for_node_exit ( self , i , timeout ) :
2017-08-15 23:34:07 +02:00
self . nodes [ i ] . process . wait ( timeout )
2017-05-07 15:13:29 +02:00
2020-06-07 15:54:56 +02:00
def connect_nodes ( self , a , b ) :
2024-09-19 13:21:16 +02:00
# A node cannot connect to itself, bail out early
if ( a == b ) :
return
2024-09-22 15:58:07 +02:00
from_connection = self . nodes [ a ]
to_connection = self . nodes [ b ]
ip_port = " 127.0.0.1: " + str ( p2p_port ( b ) )
from_connection . addnode ( ip_port , " onetry " )
2024-05-23 16:00:00 +02:00
# Use subversion as peer id. Test nodes have their node number appended to the user agent string
from_connection_subver = from_connection . getnetworkinfo ( ) [ ' subversion ' ]
to_connection_subver = to_connection . getnetworkinfo ( ) [ ' subversion ' ]
def find_conn ( node , peer_subversion , inbound ) :
return next ( filter ( lambda peer : peer [ ' subver ' ] == peer_subversion and peer [ ' inbound ' ] == inbound , node . getpeerinfo ( ) ) , None )
2024-09-22 15:58:07 +02:00
# poll until version handshake complete to avoid race conditions
# with transaction relaying
# See comments in net_processing:
# * Must have a version message before anything else
# * Must have a verack message before anything else
2024-05-23 16:00:00 +02:00
self . wait_until ( lambda : find_conn ( from_connection , to_connection_subver , inbound = False ) is not None )
self . wait_until ( lambda : find_conn ( to_connection , from_connection_subver , inbound = True ) is not None )
def check_bytesrecv ( peer , msg_type , min_bytes_recv ) :
assert peer is not None , " Error: peer disconnected "
return peer [ ' bytesrecv_per_msg ' ] . pop ( msg_type , 0 ) > = min_bytes_recv
self . wait_until ( lambda : check_bytesrecv ( find_conn ( from_connection , to_connection_subver , inbound = False ) , ' verack ' , 24 ) )
self . wait_until ( lambda : check_bytesrecv ( find_conn ( to_connection , from_connection_subver , inbound = True ) , ' verack ' , 24 ) )
2024-09-19 09:48:07 +02:00
# The message bytes are counted before processing the message, so make
# sure it was fully processed by waiting for a ping.
2024-05-23 16:00:00 +02:00
self . wait_until ( lambda : check_bytesrecv ( find_conn ( from_connection , to_connection_subver , inbound = False ) , ' pong ' , 32 ) )
self . wait_until ( lambda : check_bytesrecv ( find_conn ( to_connection , from_connection_subver , inbound = True ) , ' pong ' , 32 ) )
2020-06-07 15:54:56 +02:00
def disconnect_nodes ( self , a , b ) :
2024-09-19 13:21:16 +02:00
# A node cannot disconnect from itself, bail out early
if ( a == b ) :
return
2022-09-20 16:00:43 +02:00
def disconnect_nodes_helper ( node_a , node_b ) :
def get_peer_ids ( from_connection , node_num ) :
2022-09-24 14:36:35 +02:00
result = [ ]
for peer in from_connection . getpeerinfo ( ) :
if " testnode {} " . format ( node_num ) in peer [ ' subver ' ] :
result . append ( peer [ ' id ' ] )
return result
2022-09-20 16:00:43 +02:00
peer_ids = get_peer_ids ( node_a , node_b . index )
2022-09-24 14:36:35 +02:00
if not peer_ids :
self . log . warning ( " disconnect_nodes: {} and {} were not connected " . format (
2022-09-20 16:00:43 +02:00
node_a . index ,
node_b . index ,
2022-09-24 14:36:35 +02:00
) )
return
for peer_id in peer_ids :
try :
2022-09-20 16:00:43 +02:00
node_a . disconnectnode ( nodeid = peer_id )
2022-09-24 14:36:35 +02:00
except JSONRPCException as e :
# If this node is disconnected between calculating the peer id
# and issuing the disconnect, don't worry about it.
# This avoids a race condition if we're mass-disconnecting peers.
if e . error [ ' code ' ] != - 29 : # RPC_CLIENT_NODE_NOT_CONNECTED
raise
# wait to disconnect
2022-09-20 16:00:43 +02:00
self . wait_until ( lambda : not get_peer_ids ( node_a , node_b . index ) , timeout = 5 )
self . wait_until ( lambda : not get_peer_ids ( node_b , node_a . index ) , timeout = 5 )
2022-09-24 14:36:35 +02:00
2022-09-20 16:00:43 +02:00
disconnect_nodes_helper ( self . nodes [ a ] , self . nodes [ b ] )
2022-09-24 14:36:35 +02:00
def isolate_node ( self , node_num , timeout = 5 ) :
self . nodes [ node_num ] . setnetworkactive ( False )
2020-09-03 05:47:08 +02:00
wait_until_helper ( lambda : self . nodes [ node_num ] . getconnectioncount ( ) == 0 , timeout = timeout )
2022-09-24 14:36:35 +02:00
def reconnect_isolated_node ( self , a , b ) :
self . nodes [ a ] . setnetworkactive ( True )
self . connect_nodes ( a , b )
2020-06-07 15:54:56 +02:00
2017-05-07 15:13:29 +02:00
def split_network ( self ) :
"""
Split the network of four nodes into nodes 0 / 1 and 2 / 3.
"""
2020-06-07 15:54:56 +02:00
self . disconnect_nodes ( 1 , 2 )
2020-04-14 12:00:16 +02:00
self . sync_all ( self . nodes [ : 2 ] )
self . sync_all ( self . nodes [ 2 : ] )
2017-05-07 15:13:29 +02:00
def join_network ( self ) :
"""
Join the ( previously split ) network halves together .
"""
2020-06-07 15:54:56 +02:00
self . connect_nodes ( 1 , 2 )
2017-05-07 15:13:29 +02:00
self . sync_all ( )
2024-09-26 21:17:04 +02:00
def no_op ( self ) :
pass
def generate ( self , generator , * args , sync_fun = None , * * kwargs ) :
2024-10-01 17:56:48 +02:00
blocks = generator . generate ( * args , invalid_call = False , * * kwargs )
2024-09-26 21:17:04 +02:00
sync_fun ( ) if sync_fun else self . sync_all ( )
2024-09-26 21:13:22 +02:00
return blocks
2024-09-26 21:17:04 +02:00
def generateblock ( self , generator , * args , sync_fun = None , * * kwargs ) :
2024-10-01 17:56:48 +02:00
blocks = generator . generateblock ( * args , invalid_call = False , * * kwargs )
2024-09-26 21:17:04 +02:00
sync_fun ( ) if sync_fun else self . sync_all ( )
2024-09-26 21:13:22 +02:00
return blocks
2024-09-26 21:17:04 +02:00
def generatetoaddress ( self , generator , * args , sync_fun = None , * * kwargs ) :
2024-10-01 17:56:48 +02:00
blocks = generator . generatetoaddress ( * args , invalid_call = False , * * kwargs )
2024-09-26 21:17:04 +02:00
sync_fun ( ) if sync_fun else self . sync_all ( )
2024-09-26 21:13:22 +02:00
return blocks
2024-09-26 21:17:04 +02:00
def generatetodescriptor ( self , generator , * args , sync_fun = None , * * kwargs ) :
2024-10-01 17:56:48 +02:00
blocks = generator . generatetodescriptor ( * args , invalid_call = False , * * kwargs )
2024-09-26 21:17:04 +02:00
sync_fun ( ) if sync_fun else self . sync_all ( )
2024-09-26 21:13:22 +02:00
return blocks
2020-06-21 15:17:31 +02:00
def sync_blocks ( self , nodes = None , wait = 1 , timeout = 60 ) :
"""
Wait until everybody has the same tip .
sync_blocks needs to be called with an rpc_connections set that has least
one node already synced to the latest , stable tip , otherwise there ' s a
chance it might return before all nodes are stably synced .
"""
rpc_connections = nodes or self . nodes
timeout = int ( timeout * self . options . timeout_factor )
stop_time = time . time ( ) + timeout
while time . time ( ) < = stop_time :
best_hash = [ x . getbestblockhash ( ) for x in rpc_connections ]
if best_hash . count ( best_hash [ 0 ] ) == len ( rpc_connections ) :
return
# Check that each peer has at least one connection
assert ( all ( [ len ( x . getpeerinfo ( ) ) for x in rpc_connections ] ) )
time . sleep ( wait )
2020-04-21 16:57:52 +02:00
raise AssertionError ( " Block sync timed out after {} s: {} " . format (
timeout ,
" " . join ( " \n {!r} " . format ( b ) for b in best_hash ) ,
) )
2020-06-21 15:17:31 +02:00
def sync_mempools ( self , nodes = None , wait = 1 , timeout = 60 , flush_scheduler = True , wait_func = None ) :
"""
Wait until everybody has the same transactions in their memory
pools
"""
rpc_connections = nodes or self . nodes
timeout = int ( timeout * self . options . timeout_factor )
stop_time = time . time ( ) + timeout
if self . mocktime != 0 and wait_func is None :
wait_func = lambda : self . bump_mocktime ( 3 , nodes = nodes )
while time . time ( ) < = stop_time :
pool = [ set ( r . getrawmempool ( ) ) for r in rpc_connections ]
if pool . count ( pool [ 0 ] ) == len ( rpc_connections ) :
if flush_scheduler :
for r in rpc_connections :
2023-08-11 08:17:20 +02:00
if r . version_is_at_least ( 170000 ) :
r . syncwithvalidationinterfacequeue ( )
2020-06-21 15:17:31 +02:00
return
# Check that each peer has at least one connection
assert ( all ( [ len ( x . getpeerinfo ( ) ) for x in rpc_connections ] ) )
if wait_func is not None :
wait_func ( )
time . sleep ( wait )
2020-04-21 16:57:52 +02:00
raise AssertionError ( " Mempool sync timed out after {} s: {} " . format (
timeout ,
" " . join ( " \n {!r} " . format ( m ) for m in pool ) ,
) )
2020-06-21 15:17:31 +02:00
def sync_all ( self , nodes = None ) :
self . sync_blocks ( nodes )
self . sync_mempools ( nodes )
2017-05-07 15:13:29 +02:00
2024-08-30 14:33:15 +02:00
def bump_mocktime ( self , t , update_nodes = True , nodes = None , update_schedulers = True ) :
2024-08-30 09:47:15 +02:00
if self . mocktime == 0 :
return
2017-06-29 17:37:19 +02:00
2024-08-30 09:47:15 +02:00
self . mocktime + = t
2024-08-30 14:33:15 +02:00
if not update_nodes :
return
nodes_to_update = nodes or self . nodes
set_node_times ( nodes_to_update , self . mocktime )
if not update_schedulers :
return
for node in nodes_to_update :
if node . version_is_at_least ( 180100 ) :
node . mockscheduler ( t )
2017-06-29 17:37:19 +02:00
2024-08-30 09:47:15 +02:00
def _initialize_mocktime ( self , is_genesis ) :
if is_genesis :
self . mocktime = TIME_GENESIS_BLOCK
else :
self . mocktime = TIME_GENESIS_BLOCK + ( 199 * 156 )
2020-04-17 07:52:06 +02:00
for node in self . nodes :
node . mocktime = self . mocktime
2017-06-29 17:37:19 +02:00
Merge #19552: test: fix intermittent failure in p2p_ibd_txrelay
12410b1feb80189061eb4a2b43421e53cbb758a8 test: fix intermittent p2p_ibd_txrelay race, add test_framework.py#wait_until (Jon Atack)
Pull request description:
To fix these intermittent failures in Travis CI.
```
162/163 - p2p_ibd_txrelay.py failed, Duration: 2 s
stdout:
2020-07-19T05:44:17.213000Z TestFramework (INFO):
Check that nodes set minfilter to MAX_MONEY while still in IBD
2020-07-19T05:44:17.216000Z TestFramework (ERROR): Assertion failed
Traceback (most recent call last):
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/test_framework/test_framework.py", line 117, in main
self.run_test()
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/p2p_ibd_txrelay.py", line 30, in run_test
assert_equal(conn_info['minfeefilter'], MAX_FEE_FILTER)
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/test_framework/util.py", line 49, in assert_equal
raise AssertionError("not(%s)" % " == ".join(str(arg) for arg in (thing1, thing2) + args))
AssertionError: not(0E-8 == 0.09170997)
2020-07-19T05:44:17.293000Z TestFramework (INFO): Stopping nodes
```
At Marco's suggestion, cherry-picked part of #19134 to nicely simplify using `wait_until`.
ACKs for top commit:
vasild:
ACK 12410b1fe
Tree-SHA512: 615f509883682fd693e578b259cba35a9fa0bc519f1394e88c857e8b0650bfec5397bfa856cfa9e6d5ef81d0ee6ad02e4ad2b0eb0bd530b4c281cbe3e663790b
2020-07-21 16:01:52 +02:00
def wait_until ( self , test_function , timeout = 60 , lock = None ) :
2020-09-03 05:47:08 +02:00
return wait_until_helper ( test_function , timeout = timeout , lock = lock , timeout_factor = self . options . timeout_factor )
Merge #19552: test: fix intermittent failure in p2p_ibd_txrelay
12410b1feb80189061eb4a2b43421e53cbb758a8 test: fix intermittent p2p_ibd_txrelay race, add test_framework.py#wait_until (Jon Atack)
Pull request description:
To fix these intermittent failures in Travis CI.
```
162/163 - p2p_ibd_txrelay.py failed, Duration: 2 s
stdout:
2020-07-19T05:44:17.213000Z TestFramework (INFO):
Check that nodes set minfilter to MAX_MONEY while still in IBD
2020-07-19T05:44:17.216000Z TestFramework (ERROR): Assertion failed
Traceback (most recent call last):
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/test_framework/test_framework.py", line 117, in main
self.run_test()
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/p2p_ibd_txrelay.py", line 30, in run_test
assert_equal(conn_info['minfeefilter'], MAX_FEE_FILTER)
File "/Users/travis/build/bitcoin/bitcoin/ci/scratch/build/bitcoin-x86_64-apple-darwin16/test/functional/test_framework/util.py", line 49, in assert_equal
raise AssertionError("not(%s)" % " == ".join(str(arg) for arg in (thing1, thing2) + args))
AssertionError: not(0E-8 == 0.09170997)
2020-07-19T05:44:17.293000Z TestFramework (INFO): Stopping nodes
```
At Marco's suggestion, cherry-picked part of #19134 to nicely simplify using `wait_until`.
ACKs for top commit:
vasild:
ACK 12410b1fe
Tree-SHA512: 615f509883682fd693e578b259cba35a9fa0bc519f1394e88c857e8b0650bfec5397bfa856cfa9e6d5ef81d0ee6ad02e4ad2b0eb0bd530b4c281cbe3e663790b
2020-07-21 16:01:52 +02:00
2017-05-07 15:13:29 +02:00
# Private helper methods. These should not be accessed by the subclass test scripts.
2019-02-26 23:04:56 +01:00
def _start_logging ( self ) :
# Add logger and logging handlers
self . log = logging . getLogger ( ' TestFramework ' )
self . log . setLevel ( logging . DEBUG )
# Create file handler to log all messages
2018-06-12 14:52:26 +02:00
fh = logging . FileHandler ( self . options . tmpdir + ' /test_framework.log ' , encoding = ' utf-8 ' )
2019-02-26 23:04:56 +01:00
fh . setLevel ( logging . DEBUG )
# Create console handler to log messages to stderr. By default this logs only error messages, but can be configured with --loglevel.
ch = logging . StreamHandler ( sys . stdout )
# User can provide log level as a number or string (eg DEBUG). loglevel was caught as a string, so try to convert it to an int
ll = int ( self . options . loglevel ) if self . options . loglevel . isdigit ( ) else self . options . loglevel . upper ( )
ch . setLevel ( ll )
2019-08-09 01:21:57 +02:00
# Format logs the same as dashd's debug.log with microprecision (so log files can be concatenated and sorted)
2018-03-10 14:08:15 +01:00
formatter = logging . Formatter ( fmt = ' %(asctime)s . %(msecs)03d 000Z %(name)s ( %(levelname)s ): %(message)s ' , datefmt = ' % Y- % m- %d T % H: % M: % S ' )
2017-03-22 13:03:26 +01:00
formatter . converter = time . gmtime
2019-02-26 23:04:56 +01:00
fh . setFormatter ( formatter )
ch . setFormatter ( formatter )
# add the handlers to the logger
self . log . addHandler ( fh )
self . log . addHandler ( ch )
if self . options . trace_rpc :
rpc_logger = logging . getLogger ( " BitcoinRPC " )
rpc_logger . setLevel ( logging . DEBUG )
rpc_handler = logging . StreamHandler ( sys . stdout )
rpc_handler . setLevel ( logging . DEBUG )
rpc_logger . addHandler ( rpc_handler )
2019-05-24 13:00:54 +02:00
def _initialize_chain ( self ) :
2017-05-07 15:13:29 +02:00
""" Initialize a pre-mined blockchain for use by the test.
2019-05-24 13:00:54 +02:00
Create a cache of a 199 - block - long chain
2017-05-07 15:13:29 +02:00
Afterward , create num_nodes copies from the cache . """
2019-05-24 13:00:54 +02:00
CACHE_NODE_ID = 0 # Use node 0 to create the cache for all other nodes
cache_node_dir = get_datadir_path ( self . options . cachedir , CACHE_NODE_ID )
2017-09-01 18:47:13 +02:00
assert self . num_nodes < = MAX_NODES
2017-05-07 15:13:29 +02:00
2019-05-24 13:00:54 +02:00
if not os . path . isdir ( cache_node_dir ) :
self . log . debug ( " Creating cache directory {} " . format ( cache_node_dir ) )
initialize_datadir ( self . options . cachedir , CACHE_NODE_ID , self . chain )
self . nodes . append (
TestNode (
CACHE_NODE_ID ,
cache_node_dir ,
chain = self . chain ,
extra_conf = [ " bind=127.0.0.1 " ] ,
2024-08-30 09:47:15 +02:00
extra_args = [ ' -disablewallet ' , f " -mocktime= { TIME_GENESIS_BLOCK } " ] ,
2019-05-24 13:00:54 +02:00
extra_args_from_options = self . extra_args_from_options ,
rpchost = None ,
2018-12-29 20:18:43 +01:00
timewait = self . rpc_timeout ,
2020-05-19 02:00:24 +02:00
timeout_factor = self . options . timeout_factor ,
2018-12-29 20:18:43 +01:00
bitcoind = self . options . bitcoind ,
bitcoin_cli = self . options . bitcoincli ,
mocktime = self . mocktime ,
coverage_dir = None ,
2019-02-19 16:12:07 +01:00
cwd = self . options . tmpdir ,
Merge #16528: Native Descriptor Wallets using DescriptorScriptPubKeyMan
223588b1bbc63dc57098bbd0baa48635e0cc0b82 Add a --descriptors option to various tests (Andrew Chow)
869f7ab30aeb4d7fbd563c535b55467a8a0430cf tests: Add RPCOverloadWrapper which overloads some disabled RPCs (Andrew Chow)
cf060628590fab87d73f278e744d70ef2d5d81db Correctly check for default wallet (Andrew Chow)
886e0d75f5fea2421190aa4812777d89f68962cc Implement CWallet::IsSpentKey for non-LegacySPKMans (Andrew Chow)
3c19fdd2a2fd5394fcfa75b2ba84ab2277cbdabf Return error when no ScriptPubKeyMan is available for specified type (Andrew Chow)
388ba94231f2f10a0be751c562cdd4650510a90a Change wallet_encryption.py to use signmessage instead of dumpprivkey (Andrew Chow)
1346e14831489f9c8f53a08f9dfed61d55d53c6f Functional tests for descriptor wallets (Andrew Chow)
f193ea889ddb53d9a5c47647966681d525e38368 add importdescriptors RPC and tests for native descriptor wallets (Hugo Nguyen)
ce24a944940019185efebcc5d85eac458ed26016 Add IsLegacy to CWallet so that the GUI knows whether to show watchonly (Andrew Chow)
1cb42b22b11c27e64462afc25a94b2fc50bfa113 Generate new descriptors when encrypting (Andrew Chow)
82ae02b1656819f4bd5023b8955447e1d4ea8692 Be able to create new wallets with DescriptorScriptPubKeyMans as backing (Andrew Chow)
b713baa75a62335ab9c0eed9ef76a95bfec30668 Implement GetMetadata in DescriptorScriptPubKeyMan (Andrew Chow)
8b9603bd0b443e2f7984eb72bf2e21cf02af0bcb Change GetMetadata to use unique_ptr<CKeyMetadata> (Andrew Chow)
72a9540df96ffdb94f039b9c14eaacdc7d961196 Implement FillPSBT in DescriptorScriptPubKeyMan (Andrew Chow)
84b4978c02102171775c77a45f6ec198930f0a88 Implement SignMessage for descriptor wallets (Andrew Chow)
bde7c9fa38775a81d53ac0484fa9c98076a0c7d1 Implement SignTransaction in DescriptorScriptPubKeyMan (Andrew Chow)
d50c8ddd4190f20bf0debd410348b73408ec3143 Implement GetSolvingProvider for DescriptorScriptPubKeyMan (Andrew Chow)
f1ca5feb4ad668a3e1ae543d0addd5f483f1a88f Implement GetKeypoolOldestTime and only display it if greater than 0 (Andrew Chow)
586b57a9a6b4b12a78f792785b63a5a1743bce0c Implement ReturnDestination in DescriptorScriptPubKeyMan (Andrew Chow)
f866957979c23cefd41efa9dae9e53b9177818dc Implement GetReservedDestination in DescriptorScriptPubKeyMan (Andrew Chow)
a775f7c7fd0b9094fcbeee6ba92206d5bbb19164 Implement Unlock and Encrypt in DescriptorScriptPubKeyMan (Andrew Chow)
bfdd0734869a22217c15858d7a76d0dacc2ebc86 Implement GetNewDestination for DescriptorScriptPubKeyMan (Andrew Chow)
58c7651821b0eeff0a99dc61d78d2e9e07986580 Implement TopUp in DescriptorScriptPubKeyMan (Andrew Chow)
e014886a342508f7c8d80323eee9a5f314eaf94c Implement SetupGeneration for DescriptorScriptPubKeyMan (Andrew Chow)
46dfb99768e7d03a3cf552812d5b41ceaebc06be Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file (Andrew Chow)
4cb9b69be031e1dc65d8964794781b347fd948f5 Implement several simple functions in DescriptorScriptPubKeyMan (Andrew Chow)
d1ec3e4f19487b4b100f80ad02eac063c571777d Add IsSingleType to Descriptors (Andrew Chow)
953feb3d2724f5398dd48990c4957a19313d2c8c Implement loading of keys for DescriptorScriptPubKeyMan (Andrew Chow)
2363e9fcaa41b68bf11153f591b95f2d41ff9a1a Load the descriptor cache from the wallet file (Andrew Chow)
46c46aebb7943e1e2e96755e94dc6c197920bf75 Implement GetID for DescriptorScriptPubKeyMan (Andrew Chow)
ec2f9e1178c8e38c0a5ca063fe81adac8f916348 Implement IsHDEnabled in DescriptorScriptPubKeyMan (Andrew Chow)
741122d4c1a62ced3e96d16d67f4eeb3a6522d99 Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan (Andrew Chow)
2db7ca765c8fb2c71dd6f7c4f29ad70e68ff1720 Implement IsMine for DescriptorScriptPubKeyMan (Andrew Chow)
db7177af8c159abbcc209f2caafcd45d54c181c5 Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet (Andrew Chow)
78f8a92910d34247fa5d04368338c598d9908267 Implement SetType in DescriptorScriptPubKeyMan (Andrew Chow)
834de0300cde57ca3f662fb7aa5b1bdaed68bc8f Store WalletDescriptor in DescriptorScriptPubKeyMan (Andrew Chow)
d8132669e10c1db9ae0c2ea0d3f822d7d2f01345 Add a lock cs_desc_man for DescriptorScriptPubKeyMan (Andrew Chow)
3194a7f88ac1a32997b390b4f188c4b6a4af04a5 Introduce WalletDescriptor class (Andrew Chow)
6b13cd3fa854dfaeb9e269bff3d67cacc0e5b5dc Create LegacyScriptPubKeyMan when not a descriptor wallet (Andrew Chow)
aeac157c9dc141546b45e06ba9c2e641ad86083f Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet (Andrew Chow)
96accc73f067c7c95946e9932645dd821ef67f63 Add WALLET_FLAG_DESCRIPTORS (Andrew Chow)
6b8119af53ee2fdb4c4b5b24b4e650c0dc3bd27c Introduce DescriptorScriptPubKeyMan as a dummy class (Andrew Chow)
06620302c713cae65ee8e4ff9302e4c88e2a1285 Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it (Andrew Chow)
Pull request description:
Introducing the wallet of the glorious future (again): native descriptor wallets. With native descriptor wallets, addresses are generated from descriptors. Instead of generating keys and deriving addresses from keys, addresses come from the scriptPubKeys produced by a descriptor. Native descriptor wallets will be optional for now and can only be created by using `createwallet`.
Descriptor wallets will store descriptors, master keys from the descriptor, and descriptor cache entries. Keys are derived from descriptors on the fly. In order to allow choosing different address types, 6 descriptors are needed for normal use. There is a pair of primary and change descriptors for each of the 3 address types. With the default keypool size of 1000, each descriptor has 1000 scriptPubKeys and descriptor cache entries pregenerated. This has a side effect of making wallets large since 6000 pubkeys are written to the wallet by default, instead of the current 2000. scriptPubKeys are kept only in memory and are generated every time a descriptor is loaded. By default, we use the standard BIP 44, 49, 84 derivation paths with an external and internal derivation chain for each.
Descriptors can also be imported with a new `importdescriptors` RPC.
Native descriptor wallets use the `ScriptPubKeyMan` interface introduced in #16341 to add a `DescriptorScriptPubKeyMan`. This defines a different IsMine which uses the simpler model of "does this scriptPubKey exist in this wallet". Furthermore, `DescriptorScriptPubKeyMan` does not have watchonly, so with native descriptor wallets, it is not possible to have a wallet with both watchonly and non-watchonly things. Rather a wallet with `disable_private_keys` needs to be used for watchonly things.
A `--descriptor` option was added to some tests (`wallet_basic.py`, `wallet_encryption.py`, `wallet_keypool.py`, `wallet_keypool_topup.py`, and `wallet_labels.py`) to allow for these tests to use descriptor wallets. Additionally, several RPCs are disabled for descriptor wallets (`importprivkey`, `importpubkey`, `importaddress`, `importmulti`, `addmultisigaddress`, `dumpprivkey`, `dumpwallet`, `importwallet`, and `sethdseed`).
ACKs for top commit:
Sjors:
utACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82 (rebased, nits addressed)
jonatack:
Code review re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82.
fjahr:
re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
instagibbs:
light re-ACK 223588b
meshcollider:
Code review ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
Tree-SHA512: 59bc52aeddbb769ed5f420d5d240d8137847ac821b588eb616b34461253510c1717d6a70bab8765631738747336ae06f45ba39603ccd17f483843e5ed9a90986
Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it
Introduce DescriptorScriptPubKeyMan as a dummy class
Add WALLET_FLAG_DESCRIPTORS
Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet
Create LegacyScriptPubKeyMan when not a descriptor wallet
Introduce WalletDescriptor class
WalletDescriptor is a Descriptor with other wallet metadata
Add a lock cs_desc_man for DescriptorScriptPubKeyMan
Store WalletDescriptor in DescriptorScriptPubKeyMan
Implement SetType in DescriptorScriptPubKeyMan
Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet
Implement IsMine for DescriptorScriptPubKeyMan
Adds a set of scriptPubKeys that DescriptorScriptPubKeyMan tracks.
If the given script is in that set, it is considered ISMINE_SPENDABLE
Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan
Implement IsHDEnabled in DescriptorScriptPubKeyMan
Implement GetID for DescriptorScriptPubKeyMan
Load the descriptor cache from the wallet file
Implement loading of keys for DescriptorScriptPubKeyMan
Add IsSingleType to Descriptors
IsSingleType will return whether the descriptor will give one or multiple scriptPubKeys
Implement several simple functions in DescriptorScriptPubKeyMan
Implements a bunch of one liners: UpgradeKeyMetadata, IsFirstRun, HavePrivateKeys,
KeypoolCountExternalKeys, GetKeypoolSize, GetTimeFirstKey, CanGetAddresses,
RewriteDB
Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file
Implement SetupGeneration for DescriptorScriptPubKeyMan
Implement TopUp in DescriptorScriptPubKeyMan
Implement GetNewDestination for DescriptorScriptPubKeyMan
Implement Unlock and Encrypt in DescriptorScriptPubKeyMan
Implement GetReservedDestination in DescriptorScriptPubKeyMan
Implement ReturnDestination in DescriptorScriptPubKeyMan
Implement GetKeypoolOldestTime and only display it if greater than 0
Implement GetSolvingProvider for DescriptorScriptPubKeyMan
Internally, a GetSigningProvider function is introduced which allows for
some private keys to be optionally included. This can be called with a
script as the argument (i.e. a scriptPubKey from our wallet when we are
signing) or with a pubkey. In order to know what index to expand the
private keys for that pubkey, we need to also cache all of the pubkeys
involved when we expand the descriptor. So SetCache and TopUp are
updated to do this too.
Implement SignTransaction in DescriptorScriptPubKeyMan
Implement SignMessage for descriptor wallets
Implement FillPSBT in DescriptorScriptPubKeyMan
FillPSBT will add our own scripts to the PSBT if those inputs are ours.
If an input also lists pubkeys that we happen to know the private keys
for, we will sign those inputs too.
Change GetMetadata to use unique_ptr<CKeyMetadata>
Implement GetMetadata in DescriptorScriptPubKeyMan
Be able to create new wallets with DescriptorScriptPubKeyMans as backing
Generate new descriptors when encrypting
Add IsLegacy to CWallet so that the GUI knows whether to show watchonly
add importdescriptors RPC and tests for native descriptor wallets
Co-authored-by: Andrew Chow <achow101-github@achow101.com>
Functional tests for descriptor wallets
Change wallet_encryption.py to use signmessage instead of dumpprivkey
Return error when no ScriptPubKeyMan is available for specified type
When a CWallet doesn't have a ScriptPubKeyMan for the requested type
in GetNewDestination, give a meaningful error. Also handle this in
Qt which did not do anything with errors.
Implement CWallet::IsSpentKey for non-LegacySPKMans
tests: Add RPCOverloadWrapper which overloads some disabled RPCs
RPCOverloadWrapper overloads some deprecated or disabled RPCs with
an implementation using other RPCs to avoid having a ton of code churn
around replacing those RPCs.
Add a --descriptors option to various tests
Adds a --descriptors option globally to the test framework. This will
make the test create and use descriptor wallets. However some tests may
not work with this.
Some tests are modified to work with --descriptors and run with that
option in test_runer:
* wallet_basic.py
* wallet_encryption.py
* wallet_keypool.py <---- wallet_keypool_hd.py actually
* wallet_keypool_topup.py
* wallet_labels.py
* wallet_avoidreuse.py
2019-07-16 19:34:35 +02:00
descriptors = self . options . descriptors ,
2018-12-29 20:18:43 +01:00
) )
2019-05-24 13:00:54 +02:00
self . start_node ( CACHE_NODE_ID )
2020-04-17 20:04:08 +02:00
cache_node = self . nodes [ CACHE_NODE_ID ]
2017-05-07 15:13:29 +02:00
2017-08-15 23:34:07 +02:00
# Wait for RPC connections to be ready
2020-04-17 20:04:08 +02:00
cache_node . wait_for_rpc_connection ( )
# Set a time in the past, so that blocks don't end up in the future
cache_node . setmocktime ( cache_node . getblockheader ( cache_node . getbestblockhash ( ) ) [ ' time ' ] )
2017-05-07 15:13:29 +02:00
2021-02-25 09:48:28 +01:00
# Create a 199-block-long chain; each of the 3 first nodes
2017-05-07 15:13:29 +02:00
# gets 25 mature blocks and 25 immature.
2021-02-25 09:48:28 +01:00
# The 4th address gets 25 mature and only 24 immature blocks so that the very last
2019-02-25 17:44:18 +01:00
# block in the cache does not age too much (have an old tip age).
# This is needed so that we are out of IBD when the test starts,
# see the tip age check in IsInitialBlockDownload().
2024-08-30 09:47:15 +02:00
self . _initialize_mocktime ( is_genesis = True )
2024-07-23 19:39:21 +02:00
gen_addresses = [ k . address for k in TestNode . PRIV_KEYS ] [ : 3 ] + [ ADDRESS_BCRT1_P2SH_OP_TRUE ]
assert_equal ( len ( gen_addresses ) , 4 )
2019-02-25 17:44:18 +01:00
for i in range ( 8 ) :
2024-08-30 14:33:15 +02:00
self . bump_mocktime ( ( 25 if i != 7 else 24 ) * 156 , update_schedulers = False )
2024-09-26 21:13:22 +02:00
self . generatetoaddress (
cache_node ,
2019-05-24 13:00:54 +02:00
nblocks = 25 if i != 7 else 24 ,
2024-07-23 19:39:21 +02:00
address = gen_addresses [ i % len ( gen_addresses ) ] ,
2019-05-24 13:00:54 +02:00
)
2020-04-17 20:04:08 +02:00
assert_equal ( cache_node . getblockchaininfo ( ) [ " blocks " ] , 199 )
2017-05-07 15:13:29 +02:00
2019-05-24 13:00:54 +02:00
# Shut it down, and clean up cache directories:
2017-05-07 15:13:29 +02:00
self . stop_nodes ( )
self . nodes = [ ]
2024-08-30 09:47:15 +02:00
self . mocktime = 0
2018-03-14 14:18:44 +01:00
2019-05-24 13:00:54 +02:00
def cache_path ( * paths ) :
chain = get_chain_folder ( cache_node_dir , self . chain )
return os . path . join ( cache_node_dir , chain , * paths )
2018-03-14 14:18:44 +01:00
2019-05-24 13:00:54 +02:00
os . rmdir ( cache_path ( ' wallets ' ) ) # Remove empty wallets dir
for entry in os . listdir ( cache_path ( ) ) :
if entry not in [ ' chainstate ' , ' blocks ' , ' indexes ' , ' evodb ' , ' llmq ' ] : # Keep some folders
os . remove ( cache_path ( entry ) )
2017-09-01 18:47:13 +02:00
for i in range ( self . num_nodes ) :
2019-05-24 13:00:54 +02:00
self . log . debug ( " Copy cache directory {} to node {} " . format ( cache_node_dir , i ) )
2018-03-14 14:18:44 +01:00
to_dir = get_datadir_path ( self . options . tmpdir , i )
2019-05-24 13:00:54 +02:00
shutil . copytree ( cache_node_dir , to_dir )
2021-01-22 15:58:07 +01:00
initialize_datadir ( self . options . tmpdir , i , self . chain ) # Overwrite port/rpcport in dash.conf
2017-05-07 15:13:29 +02:00
2017-09-01 18:47:13 +02:00
def _initialize_chain_clean ( self ) :
2017-05-07 15:13:29 +02:00
""" Initialize empty blockchain for use by the test.
Create an empty blockchain and num_nodes wallets .
Useful if a test case wants complete control over initialization . """
2017-09-01 18:47:13 +02:00
for i in range ( self . num_nodes ) :
2021-01-22 15:58:07 +01:00
initialize_datadir ( self . options . tmpdir , i , self . chain )
2017-05-07 15:13:29 +02:00
2018-09-13 12:33:15 +02:00
def skip_if_no_py3_zmq ( self ) :
""" Attempt to import the zmq package and skip the test if the import fails. """
try :
import zmq # noqa
except ImportError :
raise SkipTest ( " python3-zmq module not available. " )
2024-08-25 16:17:32 +02:00
def skip_if_no_python_bcc ( self ) :
""" Attempt to import the bcc package and skip the tests if the import fails. """
try :
import bcc # type: ignore[import] # noqa: F401
except ImportError :
raise SkipTest ( " bcc python module not available " )
def skip_if_no_bitcoind_tracepoints ( self ) :
""" Skip the running test if dashd has not been compiled with USDT tracepoint support. """
if not self . is_usdt_compiled ( ) :
raise SkipTest ( " dashd has not been built with USDT tracepoints enabled. " )
def skip_if_no_bpf_permissions ( self ) :
""" Skip the running test if we don ' t have permissions to do BPF syscalls and load BPF maps. """
# check for 'root' permissions
if os . geteuid ( ) != 0 :
raise SkipTest ( " no permissions to use BPF (please review the tests carefully before running them with higher privileges) " )
def skip_if_platform_not_linux ( self ) :
""" Skip the running test if we are not on a Linux platform """
if platform . system ( ) != " Linux " :
raise SkipTest ( " not on a Linux system " )
2018-09-13 12:33:15 +02:00
def skip_if_no_bitcoind_zmq ( self ) :
""" Skip the running test if dashd has not been compiled with zmq support. """
if not self . is_zmq_compiled ( ) :
raise SkipTest ( " dashd has not been built with zmq enabled. " )
def skip_if_no_wallet ( self ) :
""" Skip the running test if wallet has not been compiled. """
2023-02-14 09:48:28 +01:00
self . requires_wallet = True
2018-09-13 12:33:15 +02:00
if not self . is_wallet_compiled ( ) :
raise SkipTest ( " wallet has not been compiled. " )
2020-11-01 23:45:42 +01:00
if self . options . descriptors :
self . skip_if_no_sqlite ( )
2024-02-16 13:24:56 +01:00
else :
self . skip_if_no_bdb ( )
2018-09-13 12:33:15 +02:00
2020-10-29 17:28:07 +01:00
def skip_if_no_sqlite ( self ) :
""" Skip the running test if sqlite has not been compiled. """
if not self . is_sqlite_compiled ( ) :
raise SkipTest ( " sqlite has not been compiled. " )
2020-11-23 11:23:31 +01:00
def skip_if_no_bdb ( self ) :
""" Skip the running test if BDB has not been compiled. """
if not self . is_bdb_compiled ( ) :
raise SkipTest ( " BDB has not been compiled. " )
Merge #17497: test: skip tests when utils haven't been compiled
a67352161c68fea9764cc31aff199f112d8572c6 test: skip tool_wallet test when bitcoin-wallet isn't compiled (fanquake)
e9277baed64e1d4054a102e40b39a9aed7839c2f test: skip wallet_listreceivedby test when the cli isn't compiled (fanquake)
621d398750d9f5ce3e7ec75ccb160b3534dcc436 test: skip bitcoin_cli test when the cli isn't compiled (fanquake)
Pull request description:
Don't try and run the `interface_bitcoin_cli.py` test when `bitcoin-cli` isn't available.
```bash
stdout:
2019-11-17T01:51:41.623000Z TestFramework (INFO): Initializing test directory /var/folders/z2/cn877pxd3czdfh47mfkmbwgm0000gn/T/test_runner_₿_🏃_20191116_205141/interface_bitcoin_cli_0
2019-11-17T01:51:41.890000Z TestFramework (ERROR): Unexpected exception caught during testing
Traceback (most recent call last):
File "/Users/michael/github/bitcoin/test/functional/test_framework/test_framework.py", line 111, in main
self.run_test()
File "/Users/michael/github/bitcoin/test/functional/interface_bitcoin_cli.py", line 18, in run_test
cli_response = self.nodes[0].cli("-version").send_cli()
File "/Users/michael/github/bitcoin/test/functional/test_framework/test_node.py", line 528, in send_cli
process = subprocess.Popen(p_args, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, universal_newlines=True)
File "/Users/michael/.pyenv/versions/3.5.6/lib/python3.5/subprocess.py", line 676, in __init__
restore_signals, start_new_session)
File "/Users/michael/.pyenv/versions/3.5.6/lib/python3.5/subprocess.py", line 1289, in _execute_child
raise child_exception_type(errno_num, err_msg)
FileNotFoundError: [Errno 2] No such file or directory: '/Users/michael/github/bitcoin/src/bitcoin-cli'
```
Top commit has no ACKs.
Tree-SHA512: de27513a615d9d21271a0948e012c3209351e7374efd19bfa1bb9cda77e8fffe15d99e3424e4dbfa8cf826084f8af1670726f4703bd2b6093e7d37df4bea64f0
2019-11-19 16:11:27 +01:00
def skip_if_no_wallet_tool ( self ) :
""" Skip the running test if dash-wallet has not been compiled. """
if not self . is_wallet_tool_compiled ( ) :
raise SkipTest ( " dash-wallet has not been compiled " )
2018-09-13 12:33:15 +02:00
def skip_if_no_cli ( self ) :
""" Skip the running test if dash-cli has not been compiled. """
if not self . is_cli_compiled ( ) :
raise SkipTest ( " dash-cli has not been compiled. " )
2020-05-01 20:57:45 +02:00
def skip_if_no_previous_releases ( self ) :
""" Skip the running test if previous releases are not available. """
if not self . has_previous_releases ( ) :
raise SkipTest ( " previous releases not available or disabled " )
def has_previous_releases ( self ) :
""" Checks whether previous releases are present and enabled. """
if not os . path . isdir ( self . options . previous_releases_path ) :
2020-05-22 12:29:09 +02:00
if self . options . prev_releases :
raise AssertionError ( " Force test of previous releases but releases missing: {} " . format (
2020-05-01 20:57:45 +02:00
self . options . previous_releases_path ) )
2020-05-22 12:29:09 +02:00
return self . options . prev_releases
2020-05-01 20:57:45 +02:00
2018-09-13 12:33:15 +02:00
def is_cli_compiled ( self ) :
""" Checks whether dash-cli was compiled. """
2021-12-08 07:24:46 +01:00
return self . config [ " components " ] . getboolean ( " ENABLE_CLI " )
2018-09-13 12:33:15 +02:00
def is_wallet_compiled ( self ) :
""" Checks whether the wallet module was compiled. """
2021-12-08 07:24:46 +01:00
return self . config [ " components " ] . getboolean ( " ENABLE_WALLET " )
2018-09-13 12:33:15 +02:00
Merge #17497: test: skip tests when utils haven't been compiled
a67352161c68fea9764cc31aff199f112d8572c6 test: skip tool_wallet test when bitcoin-wallet isn't compiled (fanquake)
e9277baed64e1d4054a102e40b39a9aed7839c2f test: skip wallet_listreceivedby test when the cli isn't compiled (fanquake)
621d398750d9f5ce3e7ec75ccb160b3534dcc436 test: skip bitcoin_cli test when the cli isn't compiled (fanquake)
Pull request description:
Don't try and run the `interface_bitcoin_cli.py` test when `bitcoin-cli` isn't available.
```bash
stdout:
2019-11-17T01:51:41.623000Z TestFramework (INFO): Initializing test directory /var/folders/z2/cn877pxd3czdfh47mfkmbwgm0000gn/T/test_runner_₿_🏃_20191116_205141/interface_bitcoin_cli_0
2019-11-17T01:51:41.890000Z TestFramework (ERROR): Unexpected exception caught during testing
Traceback (most recent call last):
File "/Users/michael/github/bitcoin/test/functional/test_framework/test_framework.py", line 111, in main
self.run_test()
File "/Users/michael/github/bitcoin/test/functional/interface_bitcoin_cli.py", line 18, in run_test
cli_response = self.nodes[0].cli("-version").send_cli()
File "/Users/michael/github/bitcoin/test/functional/test_framework/test_node.py", line 528, in send_cli
process = subprocess.Popen(p_args, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, universal_newlines=True)
File "/Users/michael/.pyenv/versions/3.5.6/lib/python3.5/subprocess.py", line 676, in __init__
restore_signals, start_new_session)
File "/Users/michael/.pyenv/versions/3.5.6/lib/python3.5/subprocess.py", line 1289, in _execute_child
raise child_exception_type(errno_num, err_msg)
FileNotFoundError: [Errno 2] No such file or directory: '/Users/michael/github/bitcoin/src/bitcoin-cli'
```
Top commit has no ACKs.
Tree-SHA512: de27513a615d9d21271a0948e012c3209351e7374efd19bfa1bb9cda77e8fffe15d99e3424e4dbfa8cf826084f8af1670726f4703bd2b6093e7d37df4bea64f0
2019-11-19 16:11:27 +01:00
def is_wallet_tool_compiled ( self ) :
""" Checks whether dash-wallet was compiled. """
return self . config [ " components " ] . getboolean ( " ENABLE_WALLET_TOOL " )
2018-09-13 12:33:15 +02:00
def is_zmq_compiled ( self ) :
""" Checks whether the zmq module was compiled. """
2021-12-08 07:24:46 +01:00
return self . config [ " components " ] . getboolean ( " ENABLE_ZMQ " )
2018-09-13 12:33:15 +02:00
2024-08-25 16:17:32 +02:00
def is_usdt_compiled ( self ) :
""" Checks whether the USDT tracepoints were compiled. """
return self . config [ " components " ] . getboolean ( " ENABLE_USDT_TRACEPOINTS " )
2020-10-29 17:28:07 +01:00
def is_sqlite_compiled ( self ) :
2020-11-23 11:23:31 +01:00
""" Checks whether the wallet module was compiled with Sqlite support. """
2020-10-29 17:28:07 +01:00
return self . config [ " components " ] . getboolean ( " USE_SQLITE " )
2020-11-23 11:23:31 +01:00
def is_bdb_compiled ( self ) :
""" Checks whether the wallet module was compiled with BDB support. """
return self . config [ " components " ] . getboolean ( " USE_BDB " )
2018-09-13 12:33:15 +02:00
2018-10-20 16:08:02 +02:00
MASTERNODE_COLLATERAL = 1000
2023-08-17 21:01:12 +02:00
EVONODE_COLLATERAL = 4000
2018-10-20 16:08:02 +02:00
class MasternodeInfo :
2023-11-13 17:02:52 +01:00
def __init__ ( self , proTxHash , ownerAddr , votingAddr , rewards_address , operator_reward , pubKeyOperator , keyOperator , collateral_address , collateral_txid , collateral_vout , addr , evo = False ) :
2018-12-31 08:12:36 +01:00
self . proTxHash = proTxHash
self . ownerAddr = ownerAddr
self . votingAddr = votingAddr
2023-11-13 17:02:52 +01:00
self . rewards_address = rewards_address
self . operator_reward = operator_reward
2018-12-31 08:12:36 +01:00
self . pubKeyOperator = pubKeyOperator
self . keyOperator = keyOperator
self . collateral_address = collateral_address
self . collateral_txid = collateral_txid
self . collateral_vout = collateral_vout
2023-02-14 19:48:33 +01:00
self . addr = addr
2023-08-17 21:01:12 +02:00
self . evo = evo
2024-09-25 12:57:08 +02:00
self . node = None
self . nodeIdx = None
2018-10-20 16:08:02 +02:00
class DashTestFramework ( BitcoinTestFramework ) :
2021-04-08 22:52:05 +02:00
def set_test_params ( self ) :
""" Tests must this method to change default values for number of nodes, topology, etc """
raise NotImplementedError
2018-09-13 12:33:15 +02:00
def skip_test_if_missing_module ( self ) :
self . skip_if_no_wallet ( )
2021-04-08 22:52:05 +02:00
def run_test ( self ) :
""" Tests must override this method to define test logic """
raise NotImplementedError
2024-09-25 12:57:08 +02:00
def connect_nodes ( self , a , b ) :
for mn2 in self . mninfo :
if mn2 . node is not None :
mn2 . node . setmnthreadactive ( False )
super ( ) . connect_nodes ( a , b )
for mn2 in self . mninfo :
if mn2 . node is not None :
mn2 . node . setmnthreadactive ( True )
2024-08-27 12:30:39 +02:00
def set_dash_test_params ( self , num_nodes , masterodes_count , extra_args = None , evo_count = 0 ) :
2018-10-20 16:08:02 +02:00
self . mn_count = masterodes_count
2023-08-17 21:01:12 +02:00
self . evo_count = evo_count
2018-10-20 16:08:02 +02:00
self . num_nodes = num_nodes
self . mninfo = [ ]
self . setup_clean_chain = True
# additional args
2019-12-05 19:28:33 +01:00
if extra_args is None :
extra_args = [ [ ] ] * num_nodes
assert_equal ( len ( extra_args ) , num_nodes )
2020-01-04 12:22:41 +01:00
self . extra_args = [ copy . deepcopy ( a ) for a in extra_args ]
2019-12-05 19:28:33 +01:00
self . extra_args [ 0 ] + = [ " -sporkkey=cP4EKFyJsHT39LDqgdcB43Y3YXjNyjb5Fuas1GQSeAtjnZWmZEQK " ]
2024-08-27 12:30:39 +02:00
for i in range ( 0 , num_nodes ) :
self . extra_args [ i ] . append ( " -dip3params=2:2 " )
2019-01-23 17:36:51 +01:00
2020-01-07 13:49:51 +01:00
# LLMQ default test params (no need to pass -llmqtestparams)
self . llmq_size = 3
self . llmq_threshold = 2
2023-10-30 16:03:22 +01:00
self . llmq_size_dip0024 = 4
2020-01-07 13:49:51 +01:00
2021-02-01 17:10:19 +01:00
# This is nRequestTimeout in dash-q-recovery thread
self . quorum_data_thread_request_timeout_seconds = 10
2023-03-30 18:45:02 +02:00
# This is EXPIRATION_TIMEOUT + EXPIRATION_BIAS in CQuorumDataRequest
self . quorum_data_request_expiration_timeout = 360
2021-01-28 23:33:18 +01:00
2020-01-04 12:21:16 +01:00
2022-11-22 18:34:21 +01:00
def activate_by_name ( self , name , expected_activation_height = None ) :
2023-10-18 05:31:40 +02:00
assert not softfork_active ( self . nodes [ 0 ] , name )
2022-11-22 18:34:21 +01:00
self . log . info ( " Wait for " + name + " activation " )
2023-05-24 19:38:33 +02:00
# disable spork17 while mining blocks to activate "name" to prevent accidental quorum formation
spork17_value = self . nodes [ 0 ] . spork ( ' show ' ) [ ' SPORK_17_QUORUM_DKG_ENABLED ' ]
self . bump_mocktime ( 1 )
self . nodes [ 0 ] . sporkupdate ( " SPORK_17_QUORUM_DKG_ENABLED " , 4070908800 )
self . wait_for_sporks_same ( )
2022-10-12 19:36:17 +02:00
# mine blocks in batches
2024-09-13 07:58:27 +02:00
batch_size = 50
2022-04-16 16:46:04 +02:00
if expected_activation_height is not None :
height = self . nodes [ 0 ] . getblockcount ( )
2023-10-18 05:31:40 +02:00
assert height < expected_activation_height
2023-05-11 11:25:41 +02:00
# NOTE: getblockchaininfo shows softforks active at block (window * 3 - 1)
# since it's returning whether a softwork is active for the _next_ block.
# Hence the last block prior to the activation is (expected_activation_height - 2).
2024-08-30 14:33:15 +02:00
while expected_activation_height - height - 2 > batch_size :
2022-10-12 19:36:17 +02:00
self . bump_mocktime ( batch_size )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , batch_size , sync_fun = lambda : self . sync_blocks ( ) )
2022-04-16 16:46:04 +02:00
height + = batch_size
2023-05-11 11:25:41 +02:00
blocks_left = expected_activation_height - height - 2
2024-08-30 14:33:15 +02:00
assert blocks_left < = batch_size
2022-10-12 19:36:17 +02:00
self . bump_mocktime ( blocks_left )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , blocks_left , sync_fun = lambda : self . sync_blocks ( ) )
2019-08-15 22:02:02 +02:00
assert not softfork_active ( self . nodes [ 0 ] , name )
2023-05-11 11:25:41 +02:00
self . bump_mocktime ( 1 )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( ) )
2023-05-11 11:25:41 +02:00
else :
while not softfork_active ( self . nodes [ 0 ] , name ) :
self . bump_mocktime ( batch_size )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , batch_size , sync_fun = lambda : self . sync_blocks ( ) )
2023-05-11 11:25:41 +02:00
assert softfork_active ( self . nodes [ 0 ] , name )
2022-04-16 16:46:04 +02:00
2022-10-12 19:36:17 +02:00
# revert spork17 changes
self . bump_mocktime ( 1 )
self . nodes [ 0 ] . sporkupdate ( " SPORK_17_QUORUM_DKG_ENABLED " , spork17_value )
self . wait_for_sporks_same ( )
2022-11-22 18:34:21 +01:00
def activate_v19 ( self , expected_activation_height = None ) :
self . activate_by_name ( ' v19 ' , expected_activation_height )
2023-05-09 05:34:26 +02:00
def activate_v20 ( self , expected_activation_height = None ) :
self . activate_by_name ( ' v20 ' , expected_activation_height )
2022-12-08 10:10:26 +01:00
2024-01-14 13:46:23 +01:00
def activate_mn_rr ( self , expected_activation_height = None ) :
2024-08-21 14:55:28 +02:00
self . activate_by_name ( ' mn_rr ' , expected_activation_height )
2023-05-09 05:34:26 +02:00
2020-01-07 13:49:51 +01:00
def set_dash_llmq_test_params ( self , llmq_size , llmq_threshold ) :
self . llmq_size = llmq_size
self . llmq_threshold = llmq_threshold
for i in range ( 0 , self . num_nodes ) :
self . extra_args [ i ] . append ( " -llmqtestparams= %d : %d " % ( self . llmq_size , self . llmq_threshold ) )
2022-04-16 16:46:04 +02:00
self . extra_args [ i ] . append ( " -llmqtestinstantsendparams= %d : %d " % ( self . llmq_size , self . llmq_threshold ) )
2020-01-07 13:49:51 +01:00
2024-01-27 18:29:53 +01:00
def create_simple_node ( self , extra_args = None ) :
2018-10-20 16:08:02 +02:00
idx = len ( self . nodes )
2024-01-27 18:29:53 +01:00
self . add_nodes ( 1 , extra_args = [ extra_args [ idx ] ] )
2019-09-24 00:57:30 +02:00
self . start_node ( idx )
2018-10-20 16:08:02 +02:00
for i in range ( 0 , idx ) :
2022-09-24 14:36:35 +02:00
self . connect_nodes ( i , idx )
2018-10-20 16:08:02 +02:00
2024-01-07 02:28:47 +01:00
# TODO: to let creating Evo Nodes without instant-send available
2023-08-17 21:01:12 +02:00
def dynamically_add_masternode ( self , evo = False , rnd = None , should_be_rejected = False ) :
2023-02-14 19:48:33 +01:00
mn_idx = len ( self . nodes )
node_p2p_port = p2p_port ( mn_idx )
node_rpc_port = rpc_port ( mn_idx )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
protx_success = False
try :
2023-08-17 21:01:12 +02:00
created_mn_info = self . dynamically_prepare_masternode ( mn_idx , node_p2p_port , evo , rnd )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
protx_success = True
except :
2023-05-31 22:34:30 +02:00
self . log . info ( " dynamically_prepare_masternode failed " )
2023-04-09 07:09:18 +02:00
assert_equal ( protx_success , not should_be_rejected )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
if should_be_rejected :
2023-04-09 07:09:18 +02:00
# nothing to do
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
return
2023-02-14 19:48:33 +01:00
2023-05-24 19:38:33 +02:00
self . dynamically_initialize_datadir ( node_p2p_port , node_rpc_port )
2023-02-14 19:48:33 +01:00
node_info = self . add_dynamically_node ( self . extra_args [ 1 ] )
args = [ ' -masternodeblsprivkey= %s ' % created_mn_info . keyOperator ] + node_info . extra_args
self . start_node ( mn_idx , args )
for mn_info in self . mninfo :
if mn_info . proTxHash == created_mn_info . proTxHash :
2023-05-31 22:34:30 +02:00
mn_info . nodeIdx = mn_idx
2023-02-14 19:48:33 +01:00
mn_info . node = self . nodes [ mn_idx ]
self . connect_nodes ( mn_idx , 0 )
self . wait_for_sporks_same ( )
2024-10-01 18:06:39 +02:00
self . sync_blocks ( )
2023-02-14 19:48:33 +01:00
force_finish_mnsync ( self . nodes [ mn_idx ] )
self . log . info ( " Successfully started and synced proTx: " + str ( created_mn_info . proTxHash ) )
return created_mn_info
2023-08-17 21:01:12 +02:00
def dynamically_prepare_masternode ( self , idx , node_p2p_port , evo = False , rnd = None ) :
2023-02-14 19:48:33 +01:00
bls = self . nodes [ 0 ] . bls ( ' generate ' )
collateral_address = self . nodes [ 0 ] . getnewaddress ( )
funds_address = self . nodes [ 0 ] . getnewaddress ( )
owner_address = self . nodes [ 0 ] . getnewaddress ( )
voting_address = self . nodes [ 0 ] . getnewaddress ( )
reward_address = self . nodes [ 0 ] . getnewaddress ( )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
platform_node_id = hash160 ( b ' %d ' % rnd ) . hex ( ) if rnd is not None else hash160 ( b ' %d ' % node_p2p_port ) . hex ( )
2023-05-31 22:34:30 +02:00
platform_p2p_port = ' %d ' % ( node_p2p_port + 101 )
platform_http_port = ' %d ' % ( node_p2p_port + 102 )
2023-02-14 19:48:33 +01:00
2023-08-17 21:01:12 +02:00
collateral_amount = EVONODE_COLLATERAL if evo else MASTERNODE_COLLATERAL
2023-04-09 07:09:18 +02:00
outputs = { collateral_address : collateral_amount , funds_address : 1 }
collateral_txid = self . nodes [ 0 ] . sendmany ( " " , outputs )
self . wait_for_instantlock ( collateral_txid , self . nodes [ 0 ] )
2024-09-26 21:13:22 +02:00
tip = self . generate ( self . nodes [ 0 ] , 1 ) [ 0 ]
2023-02-14 19:48:33 +01:00
2023-04-09 07:09:18 +02:00
rawtx = self . nodes [ 0 ] . getrawtransaction ( collateral_txid , 1 , tip )
assert_equal ( rawtx [ ' confirmations ' ] , 1 )
collateral_vout = 0
2023-02-14 19:48:33 +01:00
for txout in rawtx [ ' vout ' ] :
if txout [ ' value ' ] == Decimal ( collateral_amount ) :
collateral_vout = txout [ ' n ' ]
break
assert collateral_vout is not None
ipAndPort = ' 127.0.0.1: %d ' % node_p2p_port
operatorReward = idx
2023-05-31 22:34:30 +02:00
protx_result = None
2023-08-17 21:01:12 +02:00
if evo :
protx_result = self . nodes [ 0 ] . protx ( " register_evo " , collateral_txid , collateral_vout , ipAndPort , owner_address , bls [ ' public ' ] , voting_address , operatorReward , reward_address , platform_node_id , platform_p2p_port , platform_http_port , funds_address , True )
2023-05-31 22:34:30 +02:00
else :
protx_result = self . nodes [ 0 ] . protx ( " register " , collateral_txid , collateral_vout , ipAndPort , owner_address , bls [ ' public ' ] , voting_address , operatorReward , reward_address , funds_address , True )
2023-04-09 07:09:18 +02:00
self . wait_for_instantlock ( protx_result , self . nodes [ 0 ] )
2024-09-26 21:13:22 +02:00
tip = self . generate ( self . nodes [ 0 ] , 1 ) [ 0 ]
2023-04-09 07:09:18 +02:00
assert_equal ( self . nodes [ 0 ] . getrawtransaction ( protx_result , 1 , tip ) [ ' confirmations ' ] , 1 )
2023-11-13 17:02:52 +01:00
mn_info = MasternodeInfo ( protx_result , owner_address , voting_address , reward_address , operatorReward , bls [ ' public ' ] , bls [ ' secret ' ] , collateral_address , collateral_txid , collateral_vout , ipAndPort , evo )
2023-02-14 19:48:33 +01:00
self . mninfo . append ( mn_info )
2023-08-17 21:01:12 +02:00
mn_type_str = " EvoNode " if evo else " MN "
2023-02-14 19:48:33 +01:00
self . log . info ( " Prepared %s %d : collateral_txid= %s , collateral_vout= %d , protxHash= %s " % ( mn_type_str , idx , collateral_txid , collateral_vout , protx_result ) )
return mn_info
2023-08-17 21:01:12 +02:00
def dynamically_evo_update_service ( self , evo_info , rnd = None , should_be_rejected = False ) :
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
funds_address = self . nodes [ 0 ] . getnewaddress ( )
operator_reward_address = self . nodes [ 0 ] . getnewaddress ( )
# For the sake of the test, generate random nodeid, p2p and http platform values
2023-04-09 07:09:18 +02:00
r = rnd if rnd is not None else random . randint ( 21000 , 65000 )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
platform_node_id = hash160 ( b ' %d ' % r ) . hex ( )
platform_p2p_port = ' %d ' % ( r + 1 )
platform_http_port = ' %d ' % ( r + 2 )
2023-04-09 07:09:18 +02:00
fund_txid = self . nodes [ 0 ] . sendtoaddress ( funds_address , 1 )
self . wait_for_instantlock ( fund_txid , self . nodes [ 0 ] )
2024-10-01 21:22:56 +02:00
tip = self . generate ( self . nodes [ 0 ] , 1 ) [ 0 ]
2023-04-09 07:09:18 +02:00
assert_equal ( self . nodes [ 0 ] . getrawtransaction ( fund_txid , 1 , tip ) [ ' confirmations ' ] , 1 )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
protx_success = False
try :
2023-08-17 21:01:12 +02:00
protx_result = self . nodes [ 0 ] . protx ( ' update_service_evo ' , evo_info . proTxHash , evo_info . addr , evo_info . keyOperator , platform_node_id , platform_p2p_port , platform_http_port , operator_reward_address , funds_address )
2023-04-09 07:09:18 +02:00
self . wait_for_instantlock ( protx_result , self . nodes [ 0 ] )
2024-10-01 21:22:56 +02:00
tip = self . generate ( self . nodes [ 0 ] , 1 ) [ 0 ]
2023-04-09 07:09:18 +02:00
assert_equal ( self . nodes [ 0 ] . getrawtransaction ( protx_result , 1 , tip ) [ ' confirmations ' ] , 1 )
2023-08-17 21:01:12 +02:00
self . log . info ( " Updated EvoNode %s : platformNodeID= %s , platformP2PPort= %s , platformHTTPPort= %s " % ( evo_info . proTxHash , platform_node_id , platform_p2p_port , platform_http_port ) )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
protx_success = True
except :
2023-08-17 21:01:12 +02:00
self . log . info ( " protx_evo rejected " )
2023-04-09 07:09:18 +02:00
assert_equal ( protx_success , not should_be_rejected )
fix: check HPMNs duplicate on tx broadcast (#5257)
<!--
*** Please remove the following help text before submitting: ***
Provide a general summary of your changes in the Title above
Pull requests without a rationale and clear improvement may be closed
immediately.
Please provide clear motivation for your patch and explain how it
improves
Dash Core user experience or Dash Core developer experience
significantly:
* Any test improvements or new tests that improve coverage are always
welcome.
* All other changes should have accompanying unit tests (see
`src/test/`) or
functional tests (see `test/`). Contributors should note which tests
cover
modified code. If no tests exist for a region of modified code, new
tests
should accompany the change.
* Bug fixes are most welcome when they come with steps to reproduce or
an
explanation of the potential issue as well as reasoning for the way the
bug
was fixed.
* Features are welcome, but might be rejected due to design or scope
issues.
If a feature is based on a lot of dependencies, contributors should
first
consider building the system outside of Dash Core, if possible.
-->
## Issue being fixed or feature implemented
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Before this fix, uniqueness of HPMN `platformNodeID` was checked only
while processing a block containing a `ProRegTx` or a `ProUpServTx`.
This is not enough as a `ProRegTx` or `ProUpServTx` containing duplicate
HPMN `platformNodeID` must be rejected at tx broadcast level.
## What was done?
<!--- Describe your changes in detail -->
Checking uniqueness when calling respective RPC and when receiving such
txs.
## How Has This Been Tested?
<!--- Please describe in detail how you tested your changes. -->
<!--- Include details of your testing environment, and the tests you ran
to -->
<!--- see how your change affects other areas of the code, etc. -->
## Breaking Changes
<!--- Please describe any breaking changes your code introduces -->
## Checklist:
<!--- Go over all the following points, and put an `x` in all the boxes
that apply. -->
- [x] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [x] I have added or updated relevant unit/integration/functional/e2e
tests
- [ ] I have made corresponding changes to the documentation
**For repository code-owners and collaborators only**
- [x] I have assigned this pull request to a milestone
2023-03-16 17:28:38 +01:00
2018-10-20 16:08:02 +02:00
def prepare_masternodes ( self ) :
2019-10-03 12:45:18 +02:00
self . log . info ( " Preparing %d masternodes " % self . mn_count )
2018-10-20 16:08:02 +02:00
for idx in range ( 0 , self . mn_count ) :
2023-11-13 17:02:52 +01:00
self . prepare_masternode ( idx )
2022-04-16 16:46:04 +02:00
self . sync_all ( )
2023-11-13 17:02:52 +01:00
def prepare_masternode ( self , idx ) :
2022-04-16 16:46:04 +02:00
register_fund = ( idx % 2 ) == 0
2019-04-04 08:08:44 +02:00
bls = self . nodes [ 0 ] . bls ( ' generate ' )
address = self . nodes [ 0 ] . getnewaddress ( )
2023-11-13 17:02:52 +01:00
collateral_amount = MASTERNODE_COLLATERAL
2022-04-16 16:46:04 +02:00
txid = None
2023-02-14 19:48:33 +01:00
txid = self . nodes [ 0 ] . sendtoaddress ( address , collateral_amount )
2019-04-04 08:08:44 +02:00
collateral_vout = 0
2022-04-16 16:46:04 +02:00
if not register_fund :
txraw = self . nodes [ 0 ] . getrawtransaction ( txid , True )
for vout_idx in range ( 0 , len ( txraw [ " vout " ] ) ) :
vout = txraw [ " vout " ] [ vout_idx ]
2023-02-14 19:48:33 +01:00
if vout [ " value " ] == collateral_amount :
2022-04-16 16:46:04 +02:00
collateral_vout = vout_idx
self . nodes [ 0 ] . lockunspent ( False , [ { ' txid ' : txid , ' vout ' : collateral_vout } ] )
2019-04-04 08:08:44 +02:00
# send to same address to reserve some funds for fees
self . nodes [ 0 ] . sendtoaddress ( address , 0.001 )
ownerAddr = self . nodes [ 0 ] . getnewaddress ( )
2023-11-13 17:02:52 +01:00
rewardsAddr = self . nodes [ 0 ] . getnewaddress ( )
2022-04-16 16:46:04 +02:00
votingAddr = ownerAddr
2019-04-04 08:08:44 +02:00
port = p2p_port ( len ( self . nodes ) + idx )
2020-12-24 00:24:06 +01:00
ipAndPort = ' 127.0.0.1: %d ' % port
operatorReward = idx
2020-11-28 20:14:29 +01:00
submit = ( idx % 4 ) < 2
2022-04-16 16:46:04 +02:00
if register_fund :
2020-12-24 00:24:06 +01:00
protx_result = self . nodes [ 0 ] . protx ( ' register_fund ' , address , ipAndPort , ownerAddr , bls [ ' public ' ] , votingAddr , operatorReward , rewardsAddr , address , submit )
2019-04-04 08:08:44 +02:00
else :
2024-10-01 21:22:56 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = self . no_op )
2020-12-24 00:24:06 +01:00
protx_result = self . nodes [ 0 ] . protx ( ' register ' , txid , collateral_vout , ipAndPort , ownerAddr , bls [ ' public ' ] , votingAddr , operatorReward , rewardsAddr , address , submit )
2020-11-28 20:14:29 +01:00
if submit :
proTxHash = protx_result
else :
proTxHash = self . nodes [ 0 ] . sendrawtransaction ( protx_result )
2020-12-24 00:24:06 +01:00
if operatorReward > 0 :
2024-10-01 21:22:56 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = self . no_op )
2022-04-16 16:46:04 +02:00
operatorPayoutAddress = self . nodes [ 0 ] . getnewaddress ( )
2020-12-24 00:24:06 +01:00
self . nodes [ 0 ] . protx ( ' update_service ' , proTxHash , ipAndPort , bls [ ' secret ' ] , operatorPayoutAddress , address )
2023-11-13 17:02:52 +01:00
self . mninfo . append ( MasternodeInfo ( proTxHash , ownerAddr , votingAddr , rewardsAddr , operatorReward , bls [ ' public ' ] , bls [ ' secret ' ] , address , txid , collateral_vout , ipAndPort , False ) )
2018-10-20 16:08:02 +02:00
2023-11-13 17:02:52 +01:00
self . log . info ( " Prepared MN %d : collateral_txid= %s , collateral_vout= %d , protxHash= %s " % ( idx , txid , collateral_vout , proTxHash ) )
2019-10-03 12:45:18 +02:00
2021-01-14 21:00:57 +01:00
def remove_masternode ( self , idx ) :
2019-04-04 08:08:44 +02:00
mn = self . mninfo [ idx ]
rawtx = self . nodes [ 0 ] . createrawtransaction ( [ { " txid " : mn . collateral_txid , " vout " : mn . collateral_vout } ] , { self . nodes [ 0 ] . getnewaddress ( ) : 999.9999 } )
2020-12-11 03:25:55 +01:00
rawtx = self . nodes [ 0 ] . signrawtransactionwithwallet ( rawtx )
2019-04-04 08:08:44 +02:00
self . nodes [ 0 ] . sendrawtransaction ( rawtx [ " hex " ] )
2024-09-26 21:13:22 +02:00
self . generate ( self . nodes [ 0 ] , 1 )
2019-04-04 08:08:44 +02:00
self . mninfo . remove ( mn )
2018-10-20 16:08:02 +02:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Removed masternode %d " , idx )
2019-01-23 17:36:51 +01:00
def prepare_datadirs ( self ) :
# stop faucet node so that we can copy the datadir
2019-07-04 16:48:01 +02:00
self . stop_node ( 0 )
2019-01-23 17:36:51 +01:00
start_idx = len ( self . nodes )
for idx in range ( 0 , self . mn_count ) :
2021-01-22 15:58:07 +01:00
copy_datadir ( 0 , idx + start_idx , self . options . tmpdir , self . chain )
2019-01-23 17:36:51 +01:00
# restart faucet node
2019-09-24 00:57:30 +02:00
self . start_node ( 0 )
2020-12-06 00:39:38 +01:00
force_finish_mnsync ( self . nodes [ 0 ] )
2019-01-23 17:36:51 +01:00
2018-12-31 08:12:36 +01:00
def start_masternodes ( self ) :
2019-10-03 12:45:18 +02:00
self . log . info ( " Starting %d masternodes " , self . mn_count )
2018-12-31 08:12:36 +01:00
start_idx = len ( self . nodes )
2019-01-23 17:36:51 +01:00
2019-09-24 00:57:30 +02:00
self . add_nodes ( self . mn_count )
2019-01-23 17:36:51 +01:00
executor = ThreadPoolExecutor ( max_workers = 20 )
def do_connect ( idx ) :
2019-12-05 19:31:55 +01:00
# Connect to the control node only, masternodes should take care of intra-quorum connections themselves
2022-09-24 14:36:35 +02:00
self . connect_nodes ( self . mninfo [ idx ] . nodeIdx , 0 )
2019-01-23 17:36:51 +01:00
jobs = [ ]
# start up nodes in parallel
for idx in range ( 0 , self . mn_count ) :
2020-03-30 11:28:05 +02:00
self . mninfo [ idx ] . nodeIdx = idx + start_idx
jobs . append ( executor . submit ( self . start_masternode , self . mninfo [ idx ] ) )
2019-01-23 17:36:51 +01:00
# wait for all nodes to start up
for job in jobs :
job . result ( )
jobs . clear ( )
executor . shutdown ( )
2024-09-25 13:29:45 +02:00
# connect nodes
for idx in range ( 0 , self . mn_count ) :
do_connect ( idx )
2020-03-30 11:28:05 +02:00
def start_masternode ( self , mninfo , extra_args = None ) :
args = [ ' -masternodeblsprivkey= %s ' % mninfo . keyOperator ] + self . extra_args [ mninfo . nodeIdx ]
if extra_args is not None :
args + = extra_args
self . start_node ( mninfo . nodeIdx , extra_args = args )
mninfo . node = self . nodes [ mninfo . nodeIdx ]
force_finish_mnsync ( mninfo . node )
2023-02-14 19:48:33 +01:00
def dynamically_start_masternode ( self , mnidx , extra_args = None ) :
args = [ ]
if extra_args is not None :
args + = extra_args
self . start_node ( mnidx , extra_args = args )
force_finish_mnsync ( self . nodes [ mnidx ] )
2024-01-27 18:29:53 +01:00
def setup_nodes ( self ) :
Merge #16528: Native Descriptor Wallets using DescriptorScriptPubKeyMan
223588b1bbc63dc57098bbd0baa48635e0cc0b82 Add a --descriptors option to various tests (Andrew Chow)
869f7ab30aeb4d7fbd563c535b55467a8a0430cf tests: Add RPCOverloadWrapper which overloads some disabled RPCs (Andrew Chow)
cf060628590fab87d73f278e744d70ef2d5d81db Correctly check for default wallet (Andrew Chow)
886e0d75f5fea2421190aa4812777d89f68962cc Implement CWallet::IsSpentKey for non-LegacySPKMans (Andrew Chow)
3c19fdd2a2fd5394fcfa75b2ba84ab2277cbdabf Return error when no ScriptPubKeyMan is available for specified type (Andrew Chow)
388ba94231f2f10a0be751c562cdd4650510a90a Change wallet_encryption.py to use signmessage instead of dumpprivkey (Andrew Chow)
1346e14831489f9c8f53a08f9dfed61d55d53c6f Functional tests for descriptor wallets (Andrew Chow)
f193ea889ddb53d9a5c47647966681d525e38368 add importdescriptors RPC and tests for native descriptor wallets (Hugo Nguyen)
ce24a944940019185efebcc5d85eac458ed26016 Add IsLegacy to CWallet so that the GUI knows whether to show watchonly (Andrew Chow)
1cb42b22b11c27e64462afc25a94b2fc50bfa113 Generate new descriptors when encrypting (Andrew Chow)
82ae02b1656819f4bd5023b8955447e1d4ea8692 Be able to create new wallets with DescriptorScriptPubKeyMans as backing (Andrew Chow)
b713baa75a62335ab9c0eed9ef76a95bfec30668 Implement GetMetadata in DescriptorScriptPubKeyMan (Andrew Chow)
8b9603bd0b443e2f7984eb72bf2e21cf02af0bcb Change GetMetadata to use unique_ptr<CKeyMetadata> (Andrew Chow)
72a9540df96ffdb94f039b9c14eaacdc7d961196 Implement FillPSBT in DescriptorScriptPubKeyMan (Andrew Chow)
84b4978c02102171775c77a45f6ec198930f0a88 Implement SignMessage for descriptor wallets (Andrew Chow)
bde7c9fa38775a81d53ac0484fa9c98076a0c7d1 Implement SignTransaction in DescriptorScriptPubKeyMan (Andrew Chow)
d50c8ddd4190f20bf0debd410348b73408ec3143 Implement GetSolvingProvider for DescriptorScriptPubKeyMan (Andrew Chow)
f1ca5feb4ad668a3e1ae543d0addd5f483f1a88f Implement GetKeypoolOldestTime and only display it if greater than 0 (Andrew Chow)
586b57a9a6b4b12a78f792785b63a5a1743bce0c Implement ReturnDestination in DescriptorScriptPubKeyMan (Andrew Chow)
f866957979c23cefd41efa9dae9e53b9177818dc Implement GetReservedDestination in DescriptorScriptPubKeyMan (Andrew Chow)
a775f7c7fd0b9094fcbeee6ba92206d5bbb19164 Implement Unlock and Encrypt in DescriptorScriptPubKeyMan (Andrew Chow)
bfdd0734869a22217c15858d7a76d0dacc2ebc86 Implement GetNewDestination for DescriptorScriptPubKeyMan (Andrew Chow)
58c7651821b0eeff0a99dc61d78d2e9e07986580 Implement TopUp in DescriptorScriptPubKeyMan (Andrew Chow)
e014886a342508f7c8d80323eee9a5f314eaf94c Implement SetupGeneration for DescriptorScriptPubKeyMan (Andrew Chow)
46dfb99768e7d03a3cf552812d5b41ceaebc06be Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file (Andrew Chow)
4cb9b69be031e1dc65d8964794781b347fd948f5 Implement several simple functions in DescriptorScriptPubKeyMan (Andrew Chow)
d1ec3e4f19487b4b100f80ad02eac063c571777d Add IsSingleType to Descriptors (Andrew Chow)
953feb3d2724f5398dd48990c4957a19313d2c8c Implement loading of keys for DescriptorScriptPubKeyMan (Andrew Chow)
2363e9fcaa41b68bf11153f591b95f2d41ff9a1a Load the descriptor cache from the wallet file (Andrew Chow)
46c46aebb7943e1e2e96755e94dc6c197920bf75 Implement GetID for DescriptorScriptPubKeyMan (Andrew Chow)
ec2f9e1178c8e38c0a5ca063fe81adac8f916348 Implement IsHDEnabled in DescriptorScriptPubKeyMan (Andrew Chow)
741122d4c1a62ced3e96d16d67f4eeb3a6522d99 Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan (Andrew Chow)
2db7ca765c8fb2c71dd6f7c4f29ad70e68ff1720 Implement IsMine for DescriptorScriptPubKeyMan (Andrew Chow)
db7177af8c159abbcc209f2caafcd45d54c181c5 Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet (Andrew Chow)
78f8a92910d34247fa5d04368338c598d9908267 Implement SetType in DescriptorScriptPubKeyMan (Andrew Chow)
834de0300cde57ca3f662fb7aa5b1bdaed68bc8f Store WalletDescriptor in DescriptorScriptPubKeyMan (Andrew Chow)
d8132669e10c1db9ae0c2ea0d3f822d7d2f01345 Add a lock cs_desc_man for DescriptorScriptPubKeyMan (Andrew Chow)
3194a7f88ac1a32997b390b4f188c4b6a4af04a5 Introduce WalletDescriptor class (Andrew Chow)
6b13cd3fa854dfaeb9e269bff3d67cacc0e5b5dc Create LegacyScriptPubKeyMan when not a descriptor wallet (Andrew Chow)
aeac157c9dc141546b45e06ba9c2e641ad86083f Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet (Andrew Chow)
96accc73f067c7c95946e9932645dd821ef67f63 Add WALLET_FLAG_DESCRIPTORS (Andrew Chow)
6b8119af53ee2fdb4c4b5b24b4e650c0dc3bd27c Introduce DescriptorScriptPubKeyMan as a dummy class (Andrew Chow)
06620302c713cae65ee8e4ff9302e4c88e2a1285 Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it (Andrew Chow)
Pull request description:
Introducing the wallet of the glorious future (again): native descriptor wallets. With native descriptor wallets, addresses are generated from descriptors. Instead of generating keys and deriving addresses from keys, addresses come from the scriptPubKeys produced by a descriptor. Native descriptor wallets will be optional for now and can only be created by using `createwallet`.
Descriptor wallets will store descriptors, master keys from the descriptor, and descriptor cache entries. Keys are derived from descriptors on the fly. In order to allow choosing different address types, 6 descriptors are needed for normal use. There is a pair of primary and change descriptors for each of the 3 address types. With the default keypool size of 1000, each descriptor has 1000 scriptPubKeys and descriptor cache entries pregenerated. This has a side effect of making wallets large since 6000 pubkeys are written to the wallet by default, instead of the current 2000. scriptPubKeys are kept only in memory and are generated every time a descriptor is loaded. By default, we use the standard BIP 44, 49, 84 derivation paths with an external and internal derivation chain for each.
Descriptors can also be imported with a new `importdescriptors` RPC.
Native descriptor wallets use the `ScriptPubKeyMan` interface introduced in #16341 to add a `DescriptorScriptPubKeyMan`. This defines a different IsMine which uses the simpler model of "does this scriptPubKey exist in this wallet". Furthermore, `DescriptorScriptPubKeyMan` does not have watchonly, so with native descriptor wallets, it is not possible to have a wallet with both watchonly and non-watchonly things. Rather a wallet with `disable_private_keys` needs to be used for watchonly things.
A `--descriptor` option was added to some tests (`wallet_basic.py`, `wallet_encryption.py`, `wallet_keypool.py`, `wallet_keypool_topup.py`, and `wallet_labels.py`) to allow for these tests to use descriptor wallets. Additionally, several RPCs are disabled for descriptor wallets (`importprivkey`, `importpubkey`, `importaddress`, `importmulti`, `addmultisigaddress`, `dumpprivkey`, `dumpwallet`, `importwallet`, and `sethdseed`).
ACKs for top commit:
Sjors:
utACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82 (rebased, nits addressed)
jonatack:
Code review re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82.
fjahr:
re-ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
instagibbs:
light re-ACK 223588b
meshcollider:
Code review ACK 223588b1bbc63dc57098bbd0baa48635e0cc0b82
Tree-SHA512: 59bc52aeddbb769ed5f420d5d240d8137847ac821b588eb616b34461253510c1717d6a70bab8765631738747336ae06f45ba39603ccd17f483843e5ed9a90986
Introduce SetType function to tell ScriptPubKeyMans the type and internal-ness of it
Introduce DescriptorScriptPubKeyMan as a dummy class
Add WALLET_FLAG_DESCRIPTORS
Return nullptr from GetLegacyScriptPubKeyMan if descriptor wallet
Create LegacyScriptPubKeyMan when not a descriptor wallet
Introduce WalletDescriptor class
WalletDescriptor is a Descriptor with other wallet metadata
Add a lock cs_desc_man for DescriptorScriptPubKeyMan
Store WalletDescriptor in DescriptorScriptPubKeyMan
Implement SetType in DescriptorScriptPubKeyMan
Add LoadDescriptorScriptPubKeyMan and SetActiveScriptPubKeyMan to CWallet
Implement IsMine for DescriptorScriptPubKeyMan
Adds a set of scriptPubKeys that DescriptorScriptPubKeyMan tracks.
If the given script is in that set, it is considered ISMINE_SPENDABLE
Implement MarkUnusedAddresses in DescriptorScriptPubKeyMan
Implement IsHDEnabled in DescriptorScriptPubKeyMan
Implement GetID for DescriptorScriptPubKeyMan
Load the descriptor cache from the wallet file
Implement loading of keys for DescriptorScriptPubKeyMan
Add IsSingleType to Descriptors
IsSingleType will return whether the descriptor will give one or multiple scriptPubKeys
Implement several simple functions in DescriptorScriptPubKeyMan
Implements a bunch of one liners: UpgradeKeyMetadata, IsFirstRun, HavePrivateKeys,
KeypoolCountExternalKeys, GetKeypoolSize, GetTimeFirstKey, CanGetAddresses,
RewriteDB
Implement writing descriptorkeys, descriptorckeys, and descriptors to wallet file
Implement SetupGeneration for DescriptorScriptPubKeyMan
Implement TopUp in DescriptorScriptPubKeyMan
Implement GetNewDestination for DescriptorScriptPubKeyMan
Implement Unlock and Encrypt in DescriptorScriptPubKeyMan
Implement GetReservedDestination in DescriptorScriptPubKeyMan
Implement ReturnDestination in DescriptorScriptPubKeyMan
Implement GetKeypoolOldestTime and only display it if greater than 0
Implement GetSolvingProvider for DescriptorScriptPubKeyMan
Internally, a GetSigningProvider function is introduced which allows for
some private keys to be optionally included. This can be called with a
script as the argument (i.e. a scriptPubKey from our wallet when we are
signing) or with a pubkey. In order to know what index to expand the
private keys for that pubkey, we need to also cache all of the pubkeys
involved when we expand the descriptor. So SetCache and TopUp are
updated to do this too.
Implement SignTransaction in DescriptorScriptPubKeyMan
Implement SignMessage for descriptor wallets
Implement FillPSBT in DescriptorScriptPubKeyMan
FillPSBT will add our own scripts to the PSBT if those inputs are ours.
If an input also lists pubkeys that we happen to know the private keys
for, we will sign those inputs too.
Change GetMetadata to use unique_ptr<CKeyMetadata>
Implement GetMetadata in DescriptorScriptPubKeyMan
Be able to create new wallets with DescriptorScriptPubKeyMans as backing
Generate new descriptors when encrypting
Add IsLegacy to CWallet so that the GUI knows whether to show watchonly
add importdescriptors RPC and tests for native descriptor wallets
Co-authored-by: Andrew Chow <achow101-github@achow101.com>
Functional tests for descriptor wallets
Change wallet_encryption.py to use signmessage instead of dumpprivkey
Return error when no ScriptPubKeyMan is available for specified type
When a CWallet doesn't have a ScriptPubKeyMan for the requested type
in GetNewDestination, give a meaningful error. Also handle this in
Qt which did not do anything with errors.
Implement CWallet::IsSpentKey for non-LegacySPKMans
tests: Add RPCOverloadWrapper which overloads some disabled RPCs
RPCOverloadWrapper overloads some deprecated or disabled RPCs with
an implementation using other RPCs to avoid having a ton of code churn
around replacing those RPCs.
Add a --descriptors option to various tests
Adds a --descriptors option globally to the test framework. This will
make the test create and use descriptor wallets. However some tests may
not work with this.
Some tests are modified to work with --descriptors and run with that
option in test_runer:
* wallet_basic.py
* wallet_encryption.py
* wallet_keypool.py <---- wallet_keypool_hd.py actually
* wallet_keypool_topup.py
* wallet_labels.py
* wallet_avoidreuse.py
2019-07-16 19:34:35 +02:00
extra_args = [ [ ] ] * self . num_nodes
2024-01-27 18:29:53 +01:00
if hasattr ( self , " extra_args " ) :
extra_args = self . extra_args
2019-10-03 12:45:18 +02:00
self . log . info ( " Creating and starting controller node " )
2024-01-27 18:29:53 +01:00
num_simple_nodes = self . num_nodes - self . mn_count
self . log . info ( " Creating and starting %s simple nodes " , num_simple_nodes )
for i in range ( 0 , num_simple_nodes ) :
self . create_simple_node ( extra_args )
if self . requires_wallet :
self . import_deterministic_coinbase_privkeys ( )
2023-08-17 21:01:12 +02:00
required_balance = EVONODE_COLLATERAL * self . evo_count
required_balance + = MASTERNODE_COLLATERAL * ( self . mn_count - self . evo_count ) + 100
2019-10-03 12:45:18 +02:00
self . log . info ( " Generating %d coins " % required_balance )
2018-10-20 16:08:02 +02:00
while self . nodes [ 0 ] . getbalance ( ) < required_balance :
2019-08-09 01:14:11 +02:00
self . bump_mocktime ( 1 )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 10 , sync_fun = self . no_op )
2018-12-31 08:12:36 +01:00
# create masternodes
self . prepare_masternodes ( )
2019-01-23 17:36:51 +01:00
self . prepare_datadirs ( )
2018-12-31 08:12:36 +01:00
2024-01-27 18:29:53 +01:00
def setup_network ( self ) :
self . setup_nodes ( )
2019-12-05 19:31:55 +01:00
# non-masternodes where disconnected from the control node during prepare_datadirs,
# let's reconnect them back to make sure they receive updates
2024-01-27 18:29:53 +01:00
num_simple_nodes = self . num_nodes - self . mn_count - 1
2019-12-05 19:31:55 +01:00
for i in range ( 0 , num_simple_nodes ) :
2022-09-24 14:36:35 +02:00
self . connect_nodes ( i + 1 , 0 )
2019-12-05 19:31:55 +01:00
2024-01-27 22:15:09 +01:00
self . start_masternodes ( )
2019-08-09 01:14:11 +02:00
self . bump_mocktime ( 1 )
2024-09-26 21:13:22 +02:00
self . generate ( self . nodes [ 0 ] , 1 )
2022-04-19 18:42:52 +02:00
for i in range ( 0 , num_simple_nodes ) :
force_finish_mnsync ( self . nodes [ i + 1 ] )
2020-12-12 07:57:39 +01:00
# Enable InstantSend (including block filtering) and ChainLocks by default
2022-06-18 18:52:45 +02:00
self . nodes [ 0 ] . sporkupdate ( " SPORK_2_INSTANTSEND_ENABLED " , 0 )
self . nodes [ 0 ] . sporkupdate ( " SPORK_3_INSTANTSEND_BLOCK_FILTERING " , 0 )
self . nodes [ 0 ] . sporkupdate ( " SPORK_19_CHAINLOCKS_ENABLED " , 0 )
2020-12-12 07:57:39 +01:00
self . wait_for_sporks_same ( )
2019-08-09 01:14:11 +02:00
self . bump_mocktime ( 1 )
2018-12-31 08:12:36 +01:00
2018-10-20 16:08:02 +02:00
mn_info = self . nodes [ 0 ] . masternodelist ( " status " )
2021-08-27 21:03:02 +02:00
assert len ( mn_info ) == self . mn_count
2018-10-20 16:08:02 +02:00
for status in mn_info . values ( ) :
2021-08-27 21:03:02 +02:00
assert status == ' ENABLED '
2018-10-20 16:08:02 +02:00
2019-03-18 14:50:44 +01:00
def create_raw_tx ( self , node_from , node_to , amount , min_inputs , max_inputs ) :
2020-10-17 17:57:07 +02:00
# helper which has been supposed to be removed with bitcoin#20159 but we use it
def make_change ( from_node , amount_in , amount_out , fee ) :
"""
Create change output ( s ) , return them
"""
outputs = { }
amount = amount_out + fee
change = amount_in - amount
if change > amount * 2 :
# Create an extra change output to break up big inputs
change_address = from_node . getnewaddress ( )
# Split change in two, being careful of rounding:
outputs [ change_address ] = Decimal ( change / 2 ) . quantize ( Decimal ( ' 0.00000001 ' ) , rounding = ROUND_DOWN )
change = amount_in - amount - outputs [ change_address ]
if change > 0 :
outputs [ from_node . getnewaddress ( ) ] = change
return outputs
2021-08-27 21:03:02 +02:00
assert min_inputs < = max_inputs
2018-10-20 16:08:02 +02:00
# fill inputs
2023-05-07 04:36:45 +02:00
fee = 0.001
2018-10-20 16:08:02 +02:00
inputs = [ ]
balances = node_from . listunspent ( )
in_amount = 0.0
last_amount = 0.0
for tx in balances :
if len ( inputs ) < min_inputs :
input = { }
input [ " txid " ] = tx [ ' txid ' ]
input [ ' vout ' ] = tx [ ' vout ' ]
in_amount + = float ( tx [ ' amount ' ] )
inputs . append ( input )
2023-05-07 04:36:45 +02:00
elif in_amount > = amount + fee :
2018-10-20 16:08:02 +02:00
break
elif len ( inputs ) < max_inputs :
input = { }
input [ " txid " ] = tx [ ' txid ' ]
input [ ' vout ' ] = tx [ ' vout ' ]
in_amount + = float ( tx [ ' amount ' ] )
inputs . append ( input )
else :
input = { }
input [ " txid " ] = tx [ ' txid ' ]
input [ ' vout ' ] = tx [ ' vout ' ]
in_amount - = last_amount
in_amount + = float ( tx [ ' amount ' ] )
inputs [ - 1 ] = input
last_amount = float ( tx [ ' amount ' ] )
2021-08-27 21:03:02 +02:00
assert len ( inputs ) > = min_inputs
assert len ( inputs ) < = max_inputs
2023-05-07 04:36:45 +02:00
assert in_amount > = amount + fee
2018-10-20 16:08:02 +02:00
# fill outputs
2023-05-07 04:36:45 +02:00
outputs = make_change ( node_from , satoshi_round ( in_amount ) , satoshi_round ( amount ) , satoshi_round ( fee ) )
2018-10-20 16:08:02 +02:00
receiver_address = node_to . getnewaddress ( )
outputs [ receiver_address ] = satoshi_round ( amount )
rawtx = node_from . createrawtransaction ( inputs , outputs )
2020-12-11 03:25:55 +01:00
ret = node_from . signrawtransactionwithwallet ( rawtx )
2019-05-07 14:14:33 +02:00
decoded = node_from . decoderawtransaction ( ret [ ' hex ' ] )
ret = { * * decoded , * * ret }
return ret
2018-10-20 16:08:02 +02:00
2023-04-07 17:08:37 +02:00
def wait_for_tx ( self , txid , node , expected = True , timeout = 60 ) :
2019-12-06 10:05:58 +01:00
def check_tx ( ) :
try :
2023-04-07 17:08:37 +02:00
self . bump_mocktime ( 1 )
2019-12-06 10:05:58 +01:00
return node . getrawtransaction ( txid )
except :
return False
2020-09-03 05:47:08 +02:00
if wait_until_helper ( check_tx , timeout = timeout , sleep = 1 , do_assert = expected ) and not expected :
2019-12-06 10:05:58 +01:00
raise AssertionError ( " waiting unexpectedly succeeded " )
2023-11-20 17:17:04 +01:00
def create_isdlock ( self , hextx ) :
2021-06-24 12:47:04 +02:00
tx = tx_from_hex ( hextx )
2020-12-09 20:52:11 +01:00
tx . rehash ( )
request_id_buf = ser_string ( b " islock " ) + ser_compact_size ( len ( tx . vin ) )
inputs = [ ]
for txin in tx . vin :
request_id_buf + = txin . prevout . serialize ( )
inputs . append ( txin . prevout )
request_id = hash256 ( request_id_buf ) [ : : - 1 ] . hex ( )
message_hash = tx . hash
2023-11-20 17:17:04 +01:00
llmq_type = 103
2020-12-09 20:52:11 +01:00
2023-05-07 04:36:45 +02:00
rec_sig = self . get_recovered_sig ( request_id , message_hash , llmq_type = llmq_type )
2021-10-05 19:42:34 +02:00
2023-11-20 17:17:04 +01:00
block_count = self . mninfo [ 0 ] . node . getblockcount ( )
cycle_hash = int ( self . mninfo [ 0 ] . node . getblockhash ( block_count - ( block_count % 24 ) ) , 16 )
2021-07-31 21:23:16 +02:00
isdlock = msg_isdlock ( 1 , inputs , tx . sha256 , cycle_hash , bytes . fromhex ( rec_sig [ ' sig ' ] ) )
2021-10-05 19:42:34 +02:00
2023-11-20 17:17:04 +01:00
return isdlock
2020-12-09 20:52:11 +01:00
2023-04-07 17:08:37 +02:00
def wait_for_instantlock ( self , txid , node , expected = True , timeout = 60 ) :
2019-10-01 16:14:26 +02:00
def check_instantlock ( ) :
2023-04-07 17:08:37 +02:00
self . bump_mocktime ( 1 )
2019-03-19 08:38:16 +01:00
try :
2019-10-01 16:14:26 +02:00
return node . getrawtransaction ( txid , True ) [ " instantlock " ]
2019-03-19 08:38:16 +01:00
except :
2019-10-01 16:14:26 +02:00
return False
2020-09-03 05:47:08 +02:00
if wait_until_helper ( check_instantlock , timeout = timeout , sleep = 1 , do_assert = expected ) and not expected :
2019-12-06 10:05:58 +01:00
raise AssertionError ( " waiting unexpectedly succeeded " )
2018-10-20 16:08:02 +02:00
2019-12-06 10:05:58 +01:00
def wait_for_chainlocked_block ( self , node , block_hash , expected = True , timeout = 15 ) :
2019-10-02 02:11:10 +02:00
def check_chainlocked_block ( ) :
try :
block = node . getblock ( block_hash )
return block [ " confirmations " ] > 0 and block [ " chainlock " ]
except :
2019-01-18 11:51:31 +01:00
return False
2020-09-03 05:47:08 +02:00
if wait_until_helper ( check_chainlocked_block , timeout = timeout , sleep = 0.1 , do_assert = expected ) and not expected :
2019-12-06 10:05:58 +01:00
raise AssertionError ( " waiting unexpectedly succeeded " )
2019-10-02 02:11:10 +02:00
2023-05-31 22:34:14 +02:00
def wait_for_chainlocked_block_all_nodes ( self , block_hash , timeout = 15 , expected = True ) :
2019-10-02 02:11:10 +02:00
for node in self . nodes :
2023-05-31 22:34:14 +02:00
self . wait_for_chainlocked_block ( node , block_hash , expected = expected , timeout = timeout )
2019-10-02 02:11:10 +02:00
2019-10-02 15:24:57 +02:00
def wait_for_best_chainlock ( self , node , block_hash , timeout = 15 ) :
2020-09-03 05:47:08 +02:00
wait_until_helper ( lambda : node . getbestchainlock ( ) [ " blockhash " ] == block_hash , timeout = timeout , sleep = 0.1 )
2019-10-02 02:11:10 +02:00
def wait_for_sporks_same ( self , timeout = 30 ) :
def check_sporks_same ( ) :
2023-02-14 19:48:33 +01:00
self . bump_mocktime ( 1 )
2019-10-02 02:11:10 +02:00
sporks = self . nodes [ 0 ] . spork ( ' show ' )
return all ( node . spork ( ' show ' ) == sporks for node in self . nodes [ 1 : ] )
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_sporks_same , timeout = timeout , sleep = 0.5 )
2019-01-18 11:51:31 +01:00
2022-10-12 19:36:17 +02:00
def wait_for_quorum_connections ( self , quorum_hash , expected_connections , mninfos , llmq_type_name = " llmq_test " , timeout = 60 , wait_proc = None ) :
2020-01-08 08:01:26 +01:00
def check_quorum_connections ( ) :
2022-10-12 19:36:17 +02:00
def ret ( ) :
if wait_proc is not None :
wait_proc ( )
return False
for mn in mninfos :
s = mn . node . quorum ( " dkgstatus " )
for qs in s [ " session " ] :
2022-04-16 16:46:04 +02:00
if qs [ " llmqType " ] != llmq_type_name :
continue
2022-10-12 19:36:17 +02:00
if qs [ " status " ] [ " quorumHash " ] != quorum_hash :
2022-04-16 16:46:04 +02:00
continue
2022-10-12 19:36:17 +02:00
for qc in s [ " quorumConnections " ] :
if " quorumConnections " not in qc :
continue
if qc [ " llmqType " ] != llmq_type_name :
continue
if qc [ " quorumHash " ] != quorum_hash :
continue
if len ( qc [ " quorumConnections " ] ) == 0 :
continue
cnt = 0
for c in qc [ " quorumConnections " ] :
if c [ " connected " ] :
cnt + = 1
if cnt < expected_connections :
return ret ( )
return True
# a session with no matching connections - not ok
return ret ( )
# a node with no sessions - ok
pass
# no sessions at all - not ok
return ret ( )
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_quorum_connections , timeout = timeout , sleep = 1 )
2020-01-08 08:01:26 +01:00
2022-10-12 19:36:17 +02:00
def wait_for_masternode_probes ( self , quorum_hash , mninfos , timeout = 30 , wait_proc = None , llmq_type_name = " llmq_test " ) :
2020-03-30 14:51:26 +02:00
def check_probes ( ) :
2020-04-15 16:18:05 +02:00
def ret ( ) :
if wait_proc is not None :
wait_proc ( )
return False
2020-03-30 14:51:26 +02:00
for mn in mninfos :
2020-04-15 16:18:05 +02:00
s = mn . node . quorum ( ' dkgstatus ' )
2022-10-12 19:36:17 +02:00
for qs in s [ " session " ] :
if qs [ " llmqType " ] != llmq_type_name :
2020-04-15 16:18:05 +02:00
continue
2022-10-12 19:36:17 +02:00
if qs [ " status " ] [ " quorumHash " ] != quorum_hash :
continue
for qc in s [ " quorumConnections " ] :
if qc [ " llmqType " ] != llmq_type_name :
continue
if qc [ " quorumHash " ] != quorum_hash :
continue
for c in qc [ " quorumConnections " ] :
if c [ " proTxHash " ] == mn . proTxHash :
continue
if not c [ " outbound " ] :
mn2 = mn . node . protx ( ' info ' , c [ " proTxHash " ] )
if [ m for m in mninfos if c [ " proTxHash " ] == m . proTxHash ] :
# MN is expected to be online and functioning, so let's verify that the last successful
# probe is not too old. Probes are retried after 50 minutes, while DKGs consider a probe
# as failed after 60 minutes
if mn2 [ ' metaInfo ' ] [ ' lastOutboundSuccessElapsed ' ] > 55 * 60 :
return ret ( )
else :
# MN is expected to be offline, so let's only check that the last probe is not too long ago
if mn2 [ ' metaInfo ' ] [ ' lastOutboundAttemptElapsed ' ] > 55 * 60 and mn2 [ ' metaInfo ' ] [ ' lastOutboundSuccessElapsed ' ] > 55 * 60 :
return ret ( )
2020-03-30 14:51:26 +02:00
return True
2022-10-12 19:36:17 +02:00
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_probes , timeout = timeout , sleep = 1 )
2020-03-30 14:51:26 +02:00
2022-12-17 19:20:52 +01:00
def wait_for_quorum_phase ( self , quorum_hash , phase , expected_member_count , check_received_messages , check_received_messages_count , mninfos , llmq_type_name = " llmq_test " , timeout = 30 , sleep = 0.5 ) :
2019-10-02 02:11:10 +02:00
def check_dkg_session ( ) :
2020-01-07 13:49:51 +01:00
member_count = 0
2020-03-30 10:37:49 +02:00
for mn in mninfos :
2019-02-01 08:49:01 +01:00
s = mn . node . quorum ( " dkgstatus " ) [ " session " ]
2022-04-16 16:46:04 +02:00
for qs in s :
if qs [ " llmqType " ] != llmq_type_name :
continue
qstatus = qs [ " status " ]
if qstatus [ " quorumHash " ] != quorum_hash :
continue
if qstatus [ " phase " ] != phase :
2022-10-12 19:36:17 +02:00
return False
2022-04-16 16:46:04 +02:00
if check_received_messages is not None :
if qstatus [ check_received_messages ] < check_received_messages_count :
2022-10-12 19:36:17 +02:00
return False
member_count + = 1
2022-04-19 18:42:52 +02:00
break
2022-10-12 19:36:17 +02:00
return member_count > = expected_member_count
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_dkg_session , timeout = timeout , sleep = sleep )
2019-01-18 11:51:31 +01:00
2022-04-16 16:46:04 +02:00
def wait_for_quorum_commitment ( self , quorum_hash , nodes , llmq_type = 100 , timeout = 15 ) :
2019-10-02 02:11:10 +02:00
def check_dkg_comitments ( ) :
2020-03-30 10:37:49 +02:00
for node in nodes :
2019-02-01 08:49:01 +01:00
s = node . quorum ( " dkgstatus " )
if " minableCommitments " not in s :
2022-10-12 19:36:17 +02:00
return False
2022-04-16 16:46:04 +02:00
commits = s [ " minableCommitments " ]
c_ok = False
for c in commits :
if c [ " llmqType " ] != llmq_type :
continue
if c [ " quorumHash " ] != quorum_hash :
continue
c_ok = True
2020-03-26 13:25:24 +01:00
break
2022-04-19 18:42:52 +02:00
if not c_ok :
2022-10-12 19:36:17 +02:00
return False
return True
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_dkg_comitments , timeout = timeout , sleep = 1 )
2019-01-18 11:51:31 +01:00
2022-04-16 16:46:04 +02:00
def wait_for_quorum_list ( self , quorum_hash , nodes , timeout = 15 , sleep = 2 , llmq_type_name = " llmq_test " ) :
2020-12-10 00:08:05 +01:00
def wait_func ( ) :
2022-04-16 16:46:04 +02:00
self . log . info ( " quorums: " + str ( self . nodes [ 0 ] . quorum ( " list " ) ) )
if quorum_hash in self . nodes [ 0 ] . quorum ( " list " ) [ llmq_type_name ] :
2020-12-10 00:08:05 +01:00
return True
self . bump_mocktime ( sleep , nodes = nodes )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2020-12-10 00:08:05 +01:00
return False
2020-09-03 05:47:08 +02:00
wait_until_helper ( wait_func , timeout = timeout , sleep = sleep )
2020-12-10 00:08:05 +01:00
2022-04-16 16:46:04 +02:00
def wait_for_quorums_list ( self , quorum_hash_0 , quorum_hash_1 , nodes , llmq_type_name = " llmq_test " , timeout = 15 , sleep = 2 ) :
def wait_func ( ) :
self . log . info ( " h( " + str ( self . nodes [ 0 ] . getblockcount ( ) ) + " ) quorums: " + str ( self . nodes [ 0 ] . quorum ( " list " ) ) )
if quorum_hash_0 in self . nodes [ 0 ] . quorum ( " list " ) [ llmq_type_name ] :
if quorum_hash_1 in self . nodes [ 0 ] . quorum ( " list " ) [ llmq_type_name ] :
return True
self . bump_mocktime ( sleep , nodes = nodes )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2022-04-16 16:46:04 +02:00
return False
2020-09-03 05:47:08 +02:00
wait_until_helper ( wait_func , timeout = timeout , sleep = sleep )
2022-04-16 16:46:04 +02:00
def move_blocks ( self , nodes , num_blocks ) :
self . bump_mocktime ( 1 , nodes = nodes )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , num_blocks , sync_fun = lambda : self . sync_blocks ( nodes ) )
2022-04-16 16:46:04 +02:00
def mine_quorum ( self , llmq_type_name = " llmq_test " , llmq_type = 100 , expected_connections = None , expected_members = None , expected_contributions = None , expected_complaints = 0 , expected_justifications = 0 , expected_commitments = None , mninfos_online = None , mninfos_valid = None ) :
2020-11-28 20:16:31 +01:00
spork21_active = self . nodes [ 0 ] . spork ( ' show ' ) [ ' SPORK_21_QUORUM_ALL_CONNECTED ' ] < = 1
2021-01-11 04:23:01 +01:00
spork23_active = self . nodes [ 0 ] . spork ( ' show ' ) [ ' SPORK_23_QUORUM_POSE ' ] < = 1
2020-11-28 20:16:31 +01:00
if expected_connections is None :
expected_connections = ( self . llmq_size - 1 ) if spork21_active else 2
2020-01-07 13:49:51 +01:00
if expected_members is None :
expected_members = self . llmq_size
if expected_contributions is None :
expected_contributions = self . llmq_size
if expected_commitments is None :
expected_commitments = self . llmq_size
2020-11-19 12:42:35 +01:00
if mninfos_online is None :
mninfos_online = self . mninfo . copy ( )
if mninfos_valid is None :
mninfos_valid = self . mninfo . copy ( )
2020-01-07 13:49:51 +01:00
2022-04-16 16:46:04 +02:00
self . log . info ( " Mining quorum: llmq_type_name= %s , llmq_type= %d , expected_members= %d , expected_connections= %d , expected_contributions= %d , expected_complaints= %d , expected_justifications= %d , "
" expected_commitments= %d " % ( llmq_type_name , llmq_type , expected_members , expected_connections , expected_contributions , expected_complaints ,
2019-10-03 12:45:18 +02:00
expected_justifications , expected_commitments ) )
2020-11-19 12:42:35 +01:00
nodes = [ self . nodes [ 0 ] ] + [ mn . node for mn in mninfos_online ]
2020-03-30 10:37:49 +02:00
2019-01-18 11:51:31 +01:00
# move forward to next DKG
skip_count = 24 - ( self . nodes [ 0 ] . getblockcount ( ) % 24 )
if skip_count != 0 :
2020-04-12 07:44:31 +02:00
self . bump_mocktime ( 1 , nodes = nodes )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , skip_count , sync_fun = self . no_op )
2020-06-21 15:17:31 +02:00
self . sync_blocks ( nodes )
2019-01-18 11:51:31 +01:00
2020-03-26 13:25:24 +01:00
q = self . nodes [ 0 ] . getbestblockhash ( )
2022-04-16 16:46:04 +02:00
self . log . info ( " Expected quorum_hash: " + str ( q ) )
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 1 (init) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 1 , expected_members , None , 0 , mninfos_online , llmq_type_name = llmq_type_name )
2022-10-12 19:36:17 +02:00
self . wait_for_quorum_connections ( q , expected_connections , mninfos_online , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) , llmq_type_name = llmq_type_name )
2021-01-11 04:23:01 +01:00
if spork23_active :
2022-10-12 19:36:17 +02:00
self . wait_for_masternode_probes ( q , mninfos_online , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) )
2022-04-16 16:46:04 +02:00
self . move_blocks ( nodes , 2 )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 2 (contribute) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 2 , expected_members , " receivedContributions " , expected_contributions , mninfos_online , llmq_type_name = llmq_type_name )
self . move_blocks ( nodes , 2 )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 3 (complain) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 3 , expected_members , " receivedComplaints " , expected_complaints , mninfos_online , llmq_type_name = llmq_type_name )
self . move_blocks ( nodes , 2 )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 4 (justify) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 4 , expected_members , " receivedJustifications " , expected_justifications , mninfos_online , llmq_type_name = llmq_type_name )
self . move_blocks ( nodes , 2 )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 5 (commit) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 5 , expected_members , " receivedPrematureCommitments " , expected_commitments , mninfos_online , llmq_type_name = llmq_type_name )
self . move_blocks ( nodes , 2 )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting for phase 6 (mining) " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_phase ( q , 6 , expected_members , None , 0 , mninfos_online , llmq_type_name = llmq_type_name )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Waiting final commitment " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_commitment ( q , nodes , llmq_type = llmq_type )
2019-01-18 11:51:31 +01:00
2019-10-03 12:45:18 +02:00
self . log . info ( " Mining final commitment " )
2020-04-12 07:44:31 +02:00
self . bump_mocktime ( 1 , nodes = nodes )
2021-02-04 04:01:26 +01:00
self . nodes [ 0 ] . getblocktemplate ( ) # this calls CreateNewBlock
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2020-12-10 00:08:05 +01:00
self . log . info ( " Waiting for quorum to appear in the list " )
2022-04-16 16:46:04 +02:00
self . wait_for_quorum_list ( q , nodes , llmq_type_name = llmq_type_name )
2020-12-10 00:08:05 +01:00
2022-04-16 16:46:04 +02:00
new_quorum = self . nodes [ 0 ] . quorum ( " list " , 1 ) [ llmq_type_name ] [ 0 ]
2020-12-10 00:08:05 +01:00
assert_equal ( q , new_quorum )
2022-04-16 16:46:04 +02:00
quorum_info = self . nodes [ 0 ] . quorum ( " info " , llmq_type , new_quorum )
2019-01-18 11:51:31 +01:00
2021-07-17 21:15:21 +02:00
# Mine 8 (SIGN_HEIGHT_OFFSET) more blocks to make sure that the new quorum gets eligible for signing sessions
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 8 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2018-10-20 16:08:02 +02:00
2022-04-16 16:46:04 +02:00
self . log . info ( " New quorum: height= %d , quorumHash= %s , quorumIndex= %d , minedBlock= %s " % ( quorum_info [ " height " ] , new_quorum , quorum_info [ " quorumIndex " ] , quorum_info [ " minedBlock " ] ) )
2019-10-03 12:45:18 +02:00
2019-04-04 08:15:57 +02:00
return new_quorum
2022-04-25 21:12:04 +02:00
def mine_cycle_quorum ( self , llmq_type_name = " llmq_test_dip0024 " , llmq_type = 103 , expected_connections = None , expected_members = None , expected_contributions = None , expected_complaints = 0 , expected_justifications = 0 , expected_commitments = None , mninfos_online = None , mninfos_valid = None ) :
2022-04-16 16:46:04 +02:00
spork21_active = self . nodes [ 0 ] . spork ( ' show ' ) [ ' SPORK_21_QUORUM_ALL_CONNECTED ' ] < = 1
spork23_active = self . nodes [ 0 ] . spork ( ' show ' ) [ ' SPORK_23_QUORUM_POSE ' ] < = 1
if expected_connections is None :
2023-10-30 16:03:22 +01:00
expected_connections = ( self . llmq_size_dip0024 - 1 ) if spork21_active else 2
2022-04-16 16:46:04 +02:00
if expected_members is None :
2023-10-30 16:03:22 +01:00
expected_members = self . llmq_size_dip0024
2022-04-16 16:46:04 +02:00
if expected_contributions is None :
2023-10-30 16:03:22 +01:00
expected_contributions = self . llmq_size_dip0024
2022-04-16 16:46:04 +02:00
if expected_commitments is None :
2023-10-30 16:03:22 +01:00
expected_commitments = self . llmq_size_dip0024
2022-04-16 16:46:04 +02:00
if mninfos_online is None :
mninfos_online = self . mninfo . copy ( )
if mninfos_valid is None :
mninfos_valid = self . mninfo . copy ( )
self . log . info ( " Mining quorum: expected_members= %d , expected_connections= %d , expected_contributions= %d , expected_complaints= %d , expected_justifications= %d , "
" expected_commitments= %d " % ( expected_members , expected_connections , expected_contributions , expected_complaints ,
expected_justifications , expected_commitments ) )
nodes = [ self . nodes [ 0 ] ] + [ mn . node for mn in mninfos_online ]
# move forward to next DKG
skip_count = 24 - ( self . nodes [ 0 ] . getblockcount ( ) % 24 )
self . move_blocks ( nodes , skip_count )
q_0 = self . nodes [ 0 ] . getbestblockhash ( )
self . log . info ( " Expected quorum_0 at: " + str ( self . nodes [ 0 ] . getblockcount ( ) ) )
self . log . info ( " Expected quorum_0 hash: " + str ( q_0 ) )
self . log . info ( " quorumIndex 0: Waiting for phase 1 (init) " )
self . wait_for_quorum_phase ( q_0 , 1 , expected_members , None , 0 , mninfos_online , llmq_type_name )
self . log . info ( " quorumIndex 0: Waiting for quorum connections (init) " )
2022-10-12 19:36:17 +02:00
self . wait_for_quorum_connections ( q_0 , expected_connections , mninfos_online , llmq_type_name , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) )
2022-04-16 16:46:04 +02:00
if spork23_active :
2022-10-12 19:36:17 +02:00
self . wait_for_masternode_probes ( q_0 , mninfos_online , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) , llmq_type_name = llmq_type_name )
2022-04-16 16:46:04 +02:00
self . move_blocks ( nodes , 1 )
q_1 = self . nodes [ 0 ] . getbestblockhash ( )
self . log . info ( " Expected quorum_1 at: " + str ( self . nodes [ 0 ] . getblockcount ( ) ) )
self . log . info ( " Expected quorum_1 hash: " + str ( q_1 ) )
self . log . info ( " quorumIndex 1: Waiting for phase 1 (init) " )
self . wait_for_quorum_phase ( q_1 , 1 , expected_members , None , 0 , mninfos_online , llmq_type_name )
self . log . info ( " quorumIndex 1: Waiting for quorum connections (init) " )
2022-10-12 19:36:17 +02:00
self . wait_for_quorum_connections ( q_1 , expected_connections , mninfos_online , llmq_type_name , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) )
if spork23_active :
self . wait_for_masternode_probes ( q_1 , mninfos_online , wait_proc = lambda : self . bump_mocktime ( 1 , nodes = nodes ) , llmq_type_name = llmq_type_name )
2022-04-16 16:46:04 +02:00
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 0: Waiting for phase 2 (contribute) " )
self . wait_for_quorum_phase ( q_0 , 2 , expected_members , " receivedContributions " , expected_contributions , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 1: Waiting for phase 2 (contribute) " )
self . wait_for_quorum_phase ( q_1 , 2 , expected_members , " receivedContributions " , expected_contributions , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 0: Waiting for phase 3 (complain) " )
self . wait_for_quorum_phase ( q_0 , 3 , expected_members , " receivedComplaints " , expected_complaints , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 1: Waiting for phase 3 (complain) " )
self . wait_for_quorum_phase ( q_1 , 3 , expected_members , " receivedComplaints " , expected_complaints , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 0: Waiting for phase 4 (justify) " )
self . wait_for_quorum_phase ( q_0 , 4 , expected_members , " receivedJustifications " , expected_justifications , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 1: Waiting for phase 4 (justify) " )
self . wait_for_quorum_phase ( q_1 , 4 , expected_members , " receivedJustifications " , expected_justifications , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 0: Waiting for phase 5 (commit) " )
self . wait_for_quorum_phase ( q_0 , 5 , expected_members , " receivedPrematureCommitments " , expected_commitments , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 1: Waiting for phase 5 (commit) " )
self . wait_for_quorum_phase ( q_1 , 5 , expected_members , " receivedPrematureCommitments " , expected_commitments , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 0: Waiting for phase 6 (finalization) " )
self . wait_for_quorum_phase ( q_0 , 6 , expected_members , None , 0 , mninfos_online , llmq_type_name )
self . move_blocks ( nodes , 1 )
self . log . info ( " quorumIndex 1: Waiting for phase 6 (finalization) " )
self . wait_for_quorum_phase ( q_1 , 6 , expected_members , None , 0 , mninfos_online , llmq_type_name )
self . log . info ( " Mining final commitments " )
self . bump_mocktime ( 1 , nodes = nodes )
self . nodes [ 0 ] . getblocktemplate ( ) # this calls CreateNewBlock
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2022-04-16 16:46:04 +02:00
self . log . info ( " Waiting for quorum(s) to appear in the list " )
self . wait_for_quorums_list ( q_0 , q_1 , nodes , llmq_type_name )
quorum_info_0 = self . nodes [ 0 ] . quorum ( " info " , llmq_type , q_0 )
quorum_info_1 = self . nodes [ 0 ] . quorum ( " info " , llmq_type , q_1 )
# Mine 8 (SIGN_HEIGHT_OFFSET) more blocks to make sure that the new quorum gets eligible for signing sessions
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 8 , sync_fun = lambda : self . sync_blocks ( nodes ) )
2022-04-16 16:46:04 +02:00
self . log . info ( " New quorum: height= %d , quorumHash= %s , quorumIndex= %d , minedBlock= %s " % ( quorum_info_0 [ " height " ] , q_0 , quorum_info_0 [ " quorumIndex " ] , quorum_info_0 [ " minedBlock " ] ) )
self . log . info ( " New quorum: height= %d , quorumHash= %s , quorumIndex= %d , minedBlock= %s " % ( quorum_info_1 [ " height " ] , q_1 , quorum_info_1 [ " quorumIndex " ] , quorum_info_1 [ " minedBlock " ] ) )
self . log . info ( " quorum_info_0: " + str ( quorum_info_0 ) )
self . log . info ( " quorum_info_1: " + str ( quorum_info_1 ) )
best_block_hash = self . nodes [ 0 ] . getbestblockhash ( )
block_height = self . nodes [ 0 ] . getblockcount ( )
rpc: fix and simplify `quorum rotationinfo` (#4808)
Issues with current implementation: params list is not mentioning `baseBlockHashes`, `baseBlockHashesNb` looks excessive, no default values, handling of baseBlockHash-es is off by 1 (`3 + i` should be `4 + i`).
before:
```
> help quorum rotationinfo
quorum rotationinfo "blockRequestHash" baseBlockHashesNb extraShare
Get quorum rotation information
Arguments:
1. blockRequestHash (string, required) The blockHash of the request.
2. baseBlockHashesNb (numeric, required) Number of baseBlockHashes
3. extraShare (boolean, required) Extra share
```
after:
```
> help quorum rotationinfo
quorum rotationinfo "blockRequestHash" ( extraShare "baseBlockHash..." )
Get quorum rotation information
Arguments:
1. blockRequestHash (string, required) The blockHash of the request.
2. extraShare (boolean, optional, default=false) Extra share
3. baseBlockHash... (string, optional, default=) baseBlockHashes
```
2022-04-26 19:28:57 +02:00
quorum_rotation_info = self . nodes [ 0 ] . quorum ( " rotationinfo " , best_block_hash )
2022-04-16 16:46:04 +02:00
self . log . info ( " h( " + str ( block_height ) + " ): " + str ( quorum_rotation_info ) )
return ( quorum_info_0 , quorum_info_1 )
2022-04-25 21:12:04 +02:00
def move_to_next_cycle ( self ) :
cycle_length = 24
mninfos_online = self . mninfo . copy ( )
nodes = [ self . nodes [ 0 ] ] + [ mn . node for mn in mninfos_online ]
cur_block = self . nodes [ 0 ] . getblockcount ( )
# move forward to next DKG
skip_count = cycle_length - ( cur_block % cycle_length )
if skip_count != 0 :
self . bump_mocktime ( 1 , nodes = nodes )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , skip_count , sync_fun = self . no_op )
2020-06-21 15:17:31 +02:00
self . sync_blocks ( nodes )
2022-04-25 21:12:04 +02:00
self . log . info ( ' Moved from block %d to %d ' % ( cur_block , self . nodes [ 0 ] . getblockcount ( ) ) )
2023-05-07 04:36:45 +02:00
def wait_for_recovered_sig ( self , rec_sig_id , rec_sig_msg_hash , llmq_type = 100 , timeout = 10 ) :
def check_recovered_sig ( ) :
self . bump_mocktime ( 1 )
for mn in self . mninfo :
if not mn . node . quorum ( " hasrecsig " , llmq_type , rec_sig_id , rec_sig_msg_hash ) :
return False
return True
2020-09-03 05:47:08 +02:00
wait_until_helper ( check_recovered_sig , timeout = timeout , sleep = 1 )
2023-05-07 04:36:45 +02:00
2024-07-07 12:50:04 +02:00
def get_recovered_sig ( self , rec_sig_id , rec_sig_msg_hash , llmq_type = 100 , use_platformsign = False ) :
2021-03-15 03:49:38 +01:00
# Note: recsigs aren't relayed to regular nodes by default,
# make sure to pick a mn as a node to query for recsigs.
2023-05-07 04:36:45 +02:00
try :
quorumHash = self . mninfo [ 0 ] . node . quorum ( " selectquorum " , llmq_type , rec_sig_id ) [ " quorumHash " ]
for mn in self . mninfo :
2024-07-07 12:50:04 +02:00
if use_platformsign :
mn . node . quorum ( " platformsign " , rec_sig_id , rec_sig_msg_hash , quorumHash )
else :
mn . node . quorum ( " sign " , llmq_type , rec_sig_id , rec_sig_msg_hash , quorumHash )
2023-05-07 04:36:45 +02:00
self . wait_for_recovered_sig ( rec_sig_id , rec_sig_msg_hash , llmq_type , 10 )
return self . mninfo [ 0 ] . node . quorum ( " getrecsig " , llmq_type , rec_sig_id , rec_sig_msg_hash )
except JSONRPCException as e :
self . log . info ( f " getrecsig failed with ' { e } ' " )
2021-03-15 03:49:38 +01:00
assert False
2020-11-09 22:58:49 +01:00
2022-04-16 16:46:04 +02:00
def get_quorum_masternodes ( self , q , llmq_type = 100 ) :
qi = self . nodes [ 0 ] . quorum ( ' info ' , llmq_type , q )
2020-03-26 08:42:54 +01:00
result = [ ]
for m in qi [ ' members ' ] :
2020-03-30 09:46:09 +02:00
result . append ( self . get_mninfo ( m [ ' proTxHash ' ] ) )
2020-03-26 08:42:54 +01:00
return result
2020-03-30 09:46:09 +02:00
def get_mninfo ( self , proTxHash ) :
for mn in self . mninfo :
if mn . proTxHash == proTxHash :
return mn
return None
2021-03-16 23:50:41 +01:00
def test_mn_quorum_data ( self , test_mn , quorum_type_in , quorum_hash_in , test_secret = True , expect_secret = True ) :
2021-02-01 17:10:19 +01:00
quorum_info = test_mn . node . quorum ( " info " , quorum_type_in , quorum_hash_in , True )
2021-03-16 23:50:41 +01:00
if test_secret and expect_secret != ( " secretKeyShare " in quorum_info ) :
2021-01-28 23:33:18 +01:00
return False
if " members " not in quorum_info or len ( quorum_info [ " members " ] ) == 0 :
return False
pubkey_count = 0
valid_count = 0
for quorum_member in quorum_info [ " members " ] :
valid_count + = quorum_member [ " valid " ]
pubkey_count + = " pubKeyShare " in quorum_member
return pubkey_count == valid_count
2021-03-16 23:50:41 +01:00
def wait_for_quorum_data ( self , mns , quorum_type_in , quorum_hash_in , test_secret = True , expect_secret = True ,
recover = False , timeout = 60 ) :
2021-01-28 23:33:18 +01:00
def test_mns ( ) :
valid = 0
if recover :
if self . mocktime % 2 :
2021-02-01 17:10:19 +01:00
self . bump_mocktime ( self . quorum_data_request_expiration_timeout + 1 )
2024-09-26 21:17:04 +02:00
self . generate ( self . nodes [ 0 ] , 1 , sync_fun = lambda : self . sync_blocks ( ) )
2021-02-01 17:10:19 +01:00
else :
self . bump_mocktime ( self . quorum_data_thread_request_timeout_seconds + 1 )
2021-01-28 23:33:18 +01:00
for test_mn in mns :
2021-03-16 23:50:41 +01:00
valid + = self . test_mn_quorum_data ( test_mn , quorum_type_in , quorum_hash_in , test_secret , expect_secret )
2021-01-28 23:33:18 +01:00
self . log . debug ( " wait_for_quorum_data: %d / %d - quorum_type= %d quorum_hash= %s " %
( valid , len ( mns ) , quorum_type_in , quorum_hash_in ) )
return valid == len ( mns )
2020-09-03 05:47:08 +02:00
wait_until_helper ( test_mns , timeout = timeout , sleep = 0.5 )
2021-01-28 23:33:18 +01:00
2019-12-06 10:05:58 +01:00
def wait_for_mnauth ( self , node , count , timeout = 10 ) :
def test ( ) :
pi = node . getpeerinfo ( )
c = 0
for p in pi :
if " verified_proregtx_hash " in p and p [ " verified_proregtx_hash " ] != " " :
c + = 1
return c > = count
2020-09-03 05:47:08 +02:00
wait_until_helper ( test , timeout = timeout )