mirror of
https://github.com/dashpay/dash.git
synced 2024-12-26 12:32:48 +01:00
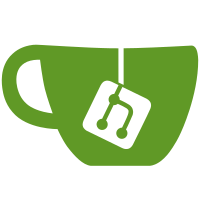
8169fc4e73a87331e02502fc24e293831765c8b1 qt, refactor: Fix code styling of moved InitExecutor class (Hennadii Stepanov) c82165a55701fe4ff604d7f30163051cd47c2363 qt, refactor: Move InitExecutor class into its own module (Hennadii Stepanov) dbcf56b6c6e939923673b3f07bed7bb3632dbeb1 scripted-diff: Rename BitcoinCore class to InitExecutor (Hennadii Stepanov) 19a1d008310f250b69b7aa764a9f26384d5a4a85 qt: Add BitcoinCore::m_thread member (Hennadii Stepanov) Pull request description: This PR makes the `BitcoinCore` class reusable, i.e., it can be used by the widget-based GUI or by the [QML-based](https://github.com/bitcoin-core/gui-qml/tree/main/src/qml) one, and it makes the divergence between these two repos minimal. The small benefit to the current branch is more structured code. Actually, this PR is ported from https://github.com/bitcoin-core/gui-qml/pull/10. The example of the re-using of the `BitcoinCore` class is https://github.com/bitcoin-core/gui-qml/pull/11. ACKs for top commit: laanwj: ACK 8169fc4e73a87331e02502fc24e293831765c8b1 ryanofsky: Code review ACK 8169fc4e73a87331e02502fc24e293831765c8b1. Only change is switching from `m_executor` from pointer to optional type (thanks for update!) Tree-SHA512: a0552c32d26d9acf42921eb12bcdf68f02d52f7183c688c43257b1a58679f64e45f193ee2d316850c7f0f516561e17abe989fe545bfa05e158ad3f4c66d19bca
86 lines
2.4 KiB
C++
86 lines
2.4 KiB
C++
// Copyright (c) 2014-2021 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <qt/initexecutor.h>
|
|
|
|
#include <interfaces/node.h>
|
|
#include <util/system.h>
|
|
#include <util/threadnames.h>
|
|
|
|
#include <exception>
|
|
|
|
#include <QApplication>
|
|
#include <QDebug>
|
|
#include <QObject>
|
|
#include <QProcess>
|
|
#include <QString>
|
|
#include <QThread>
|
|
|
|
InitExecutor::InitExecutor(interfaces::Node& node)
|
|
: QObject(), m_node(node)
|
|
{
|
|
this->moveToThread(&m_thread);
|
|
m_thread.start();
|
|
}
|
|
|
|
InitExecutor::~InitExecutor()
|
|
{
|
|
qDebug() << __func__ << ": Stopping thread";
|
|
m_thread.quit();
|
|
m_thread.wait();
|
|
qDebug() << __func__ << ": Stopped thread";
|
|
}
|
|
|
|
void InitExecutor::handleRunawayException(const std::exception_ptr e)
|
|
{
|
|
PrintExceptionContinue(e, "Runaway exception");
|
|
Q_EMIT runawayException(QString::fromStdString(m_node.getWarnings().translated));
|
|
}
|
|
|
|
void InitExecutor::initialize()
|
|
{
|
|
try {
|
|
util::ThreadRename("qt-init");
|
|
qDebug() << __func__ << ": Running initialization in thread";
|
|
interfaces::BlockAndHeaderTipInfo tip_info;
|
|
bool rv = m_node.appInitMain(&tip_info);
|
|
Q_EMIT initializeResult(rv, tip_info);
|
|
} catch (...) {
|
|
handleRunawayException(std::current_exception());
|
|
}
|
|
}
|
|
|
|
void InitExecutor::restart(QStringList args)
|
|
{
|
|
static bool executing_restart{false};
|
|
|
|
if(!executing_restart) { // Only restart 1x, no matter how often a user clicks on a restart-button
|
|
executing_restart = true;
|
|
try {
|
|
qDebug() << __func__ << ": Running Restart in thread";
|
|
m_node.appPrepareShutdown();
|
|
qDebug() << __func__ << ": Shutdown finished";
|
|
Q_EMIT shutdownResult();
|
|
CExplicitNetCleanup::callCleanup();
|
|
QProcess::startDetached(QApplication::applicationFilePath(), args);
|
|
qDebug() << __func__ << ": Restart initiated...";
|
|
QApplication::quit();
|
|
} catch (...) {
|
|
handleRunawayException(std::current_exception());
|
|
}
|
|
}
|
|
}
|
|
|
|
void InitExecutor::shutdown()
|
|
{
|
|
try {
|
|
qDebug() << __func__ << ": Running Shutdown in thread";
|
|
m_node.appShutdown();
|
|
qDebug() << __func__ << ": Shutdown finished";
|
|
Q_EMIT shutdownResult();
|
|
} catch (...) {
|
|
handleRunawayException(std::current_exception());
|
|
}
|
|
}
|