mirror of
https://github.com/dashpay/dash.git
synced 2024-12-27 13:03:17 +01:00
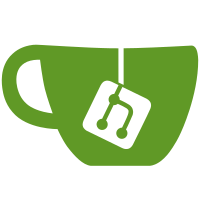
* Disable move ctor/operator for CKeyHolder Also fixes these warnings: ``` In file included from dsnotificationinterface.cpp:12: In file included from ./privatesend/privatesend-client.h:8: ./privatesend/privatesend-util.h:18:5: warning: explicitly defaulted move constructor is implicitly deleted [-Wdefaulted-function-deleted] CKeyHolder(CKeyHolder&&) = default; ^ ./privatesend/privatesend-util.h:13:17: note: move constructor of 'CKeyHolder' is implicitly deleted because field 'reserveKey' has a deleted move constructor CReserveKey reserveKey; ^ ./wallet/wallet.h:1282:5: note: 'CReserveKey' has been explicitly marked deleted here CReserveKey(const CReserveKey&) = delete; ^ In file included from dsnotificationinterface.cpp:12: In file included from ./privatesend/privatesend-client.h:8: ./privatesend/privatesend-util.h:19:17: warning: explicitly defaulted move assignment operator is implicitly deleted [-Wdefaulted-function-deleted] CKeyHolder& operator=(CKeyHolder&&) = default; ^ ./privatesend/privatesend-util.h:13:17: note: move assignment operator of 'CKeyHolder' is implicitly deleted because field 'reserveKey' has a deleted move assignment operator CReserveKey reserveKey; ^ ./wallet/wallet.h:1283:18: note: 'operator=' has been explicitly marked deleted here CReserveKey& operator=(const CReserveKey&) = delete; ^ 2 warnings generated. ``` * Slightly refactor `CKeyHolderStorage::AddKey()` to clarify that it's ptr and not the object itself that we are moving
73 lines
1.8 KiB
C++
73 lines
1.8 KiB
C++
// Copyright (c) 2014-2019 The Dash Core developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include "privatesend-util.h"
|
|
|
|
CKeyHolder::CKeyHolder(CWallet* pwallet) :
|
|
reserveKey(pwallet)
|
|
{
|
|
reserveKey.GetReservedKey(pubKey, false);
|
|
}
|
|
|
|
void CKeyHolder::KeepKey()
|
|
{
|
|
reserveKey.KeepKey();
|
|
}
|
|
|
|
void CKeyHolder::ReturnKey()
|
|
{
|
|
reserveKey.ReturnKey();
|
|
}
|
|
|
|
CScript CKeyHolder::GetScriptForDestination() const
|
|
{
|
|
return ::GetScriptForDestination(pubKey.GetID());
|
|
}
|
|
|
|
|
|
CScript CKeyHolderStorage::AddKey(CWallet* pwallet)
|
|
{
|
|
auto keyHolderPtr = std::unique_ptr<CKeyHolder>(new CKeyHolder(pwallet));
|
|
auto script = keyHolderPtr->GetScriptForDestination();
|
|
|
|
LOCK(cs_storage);
|
|
storage.emplace_back(std::move(keyHolderPtr));
|
|
LogPrintf("CKeyHolderStorage::%s -- storage size %lld\n", __func__, storage.size());
|
|
return script;
|
|
}
|
|
|
|
void CKeyHolderStorage::KeepAll()
|
|
{
|
|
std::vector<std::unique_ptr<CKeyHolder> > tmp;
|
|
{
|
|
// don't hold cs_storage while calling KeepKey(), which might lock cs_wallet
|
|
LOCK(cs_storage);
|
|
std::swap(storage, tmp);
|
|
}
|
|
|
|
if (!tmp.empty()) {
|
|
for (auto& key : tmp) {
|
|
key->KeepKey();
|
|
}
|
|
LogPrintf("CKeyHolderStorage::%s -- %lld keys kept\n", __func__, tmp.size());
|
|
}
|
|
}
|
|
|
|
void CKeyHolderStorage::ReturnAll()
|
|
{
|
|
std::vector<std::unique_ptr<CKeyHolder> > tmp;
|
|
{
|
|
// don't hold cs_storage while calling ReturnKey(), which might lock cs_wallet
|
|
LOCK(cs_storage);
|
|
std::swap(storage, tmp);
|
|
}
|
|
|
|
if (!tmp.empty()) {
|
|
for (auto& key : tmp) {
|
|
key->ReturnKey();
|
|
}
|
|
LogPrintf("CKeyHolderStorage::%s -- %lld keys returned\n", __func__, tmp.size());
|
|
}
|
|
}
|